React Js Convert String to Array | Splitting by commas.
.jpg)
React Js Convert String to Array: To convert a string to an array in React.js, you can use the split()
method. This method splits a string into an array of substrings based on a specified delimiter.
For example, if you have a string called str
and want to convert it to an array using a space as the delimiter, you can use the following code: const arr = str.split(' ');
.
This will create an array where each word in the string becomes an element. You can then access and manipulate the array as needed within your React.js application.
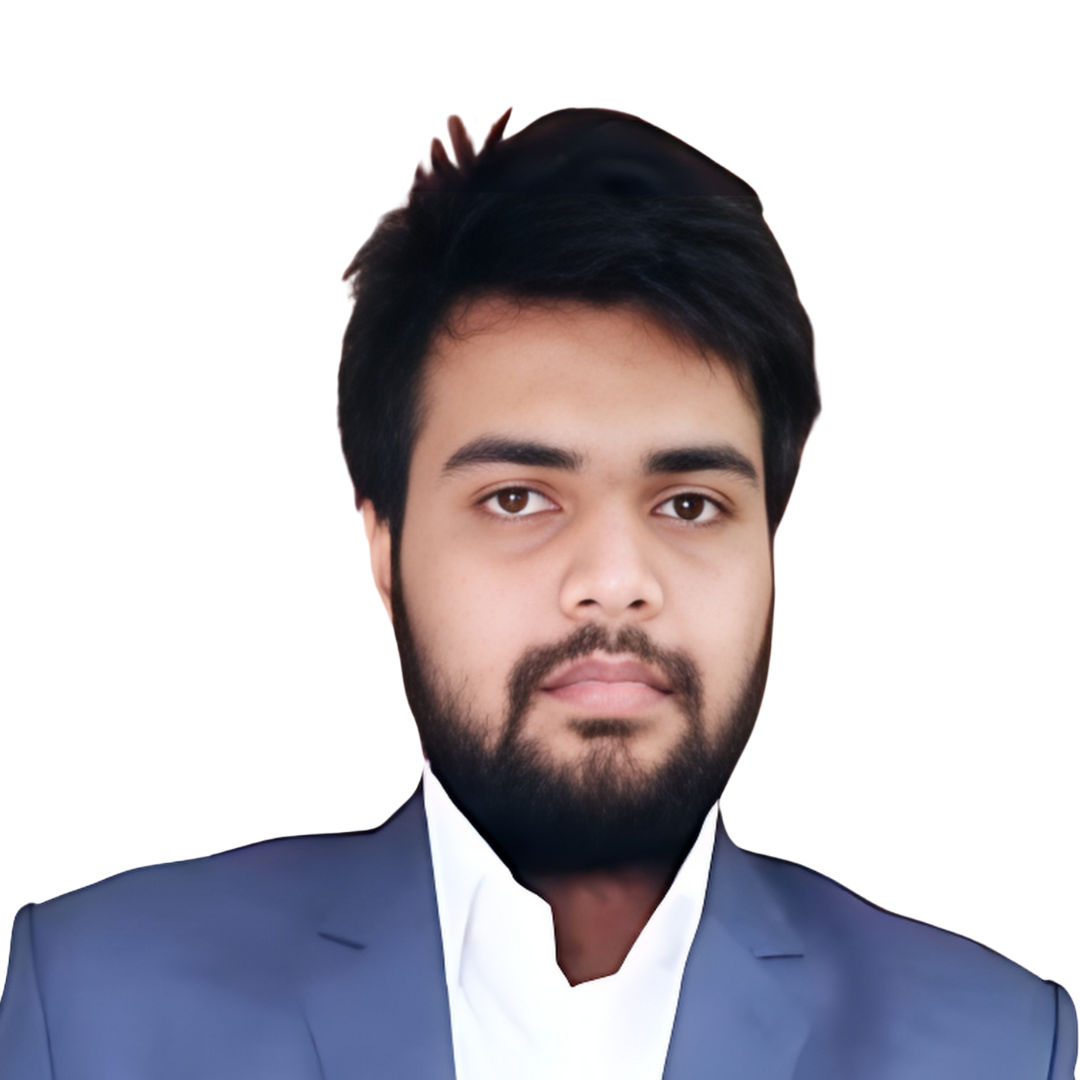
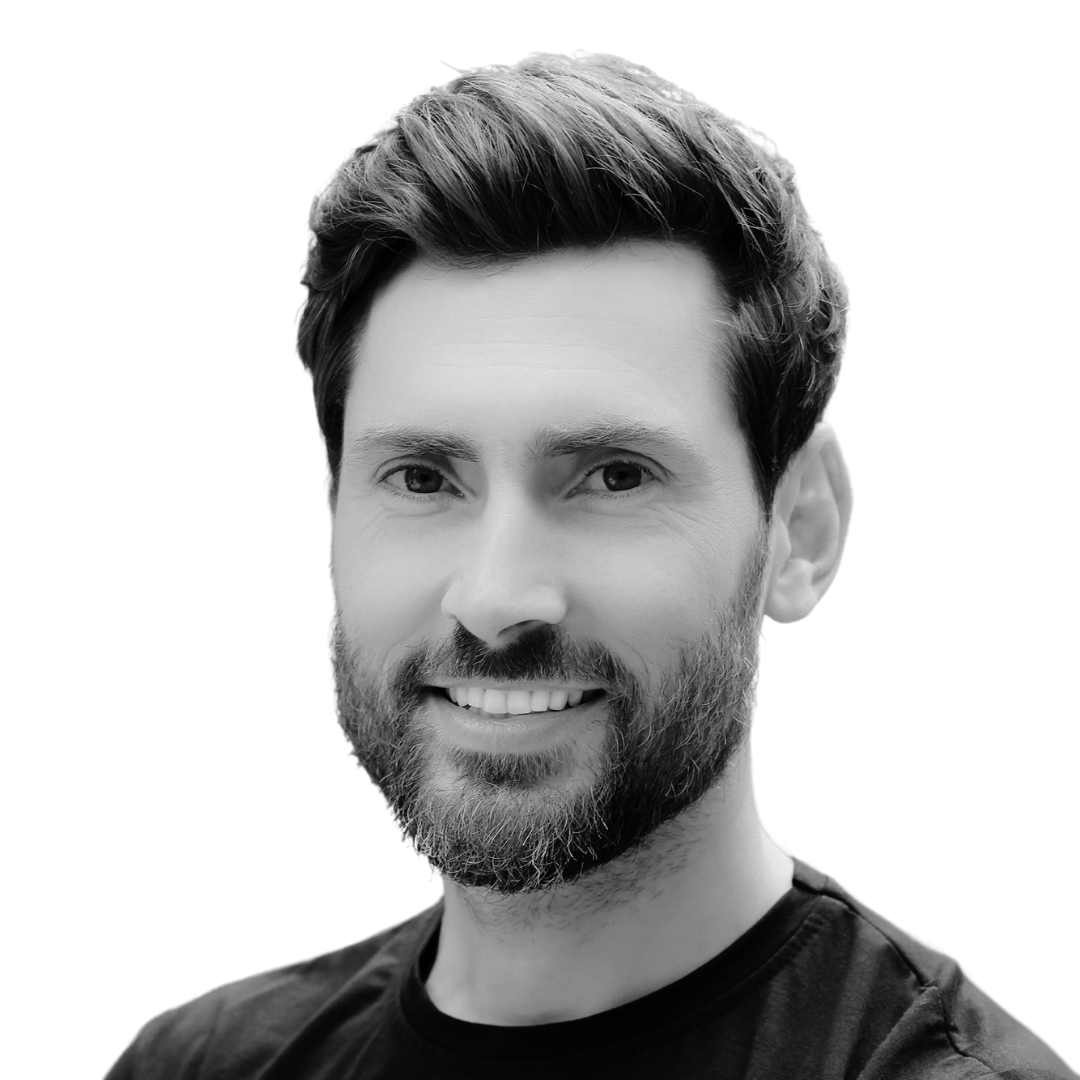
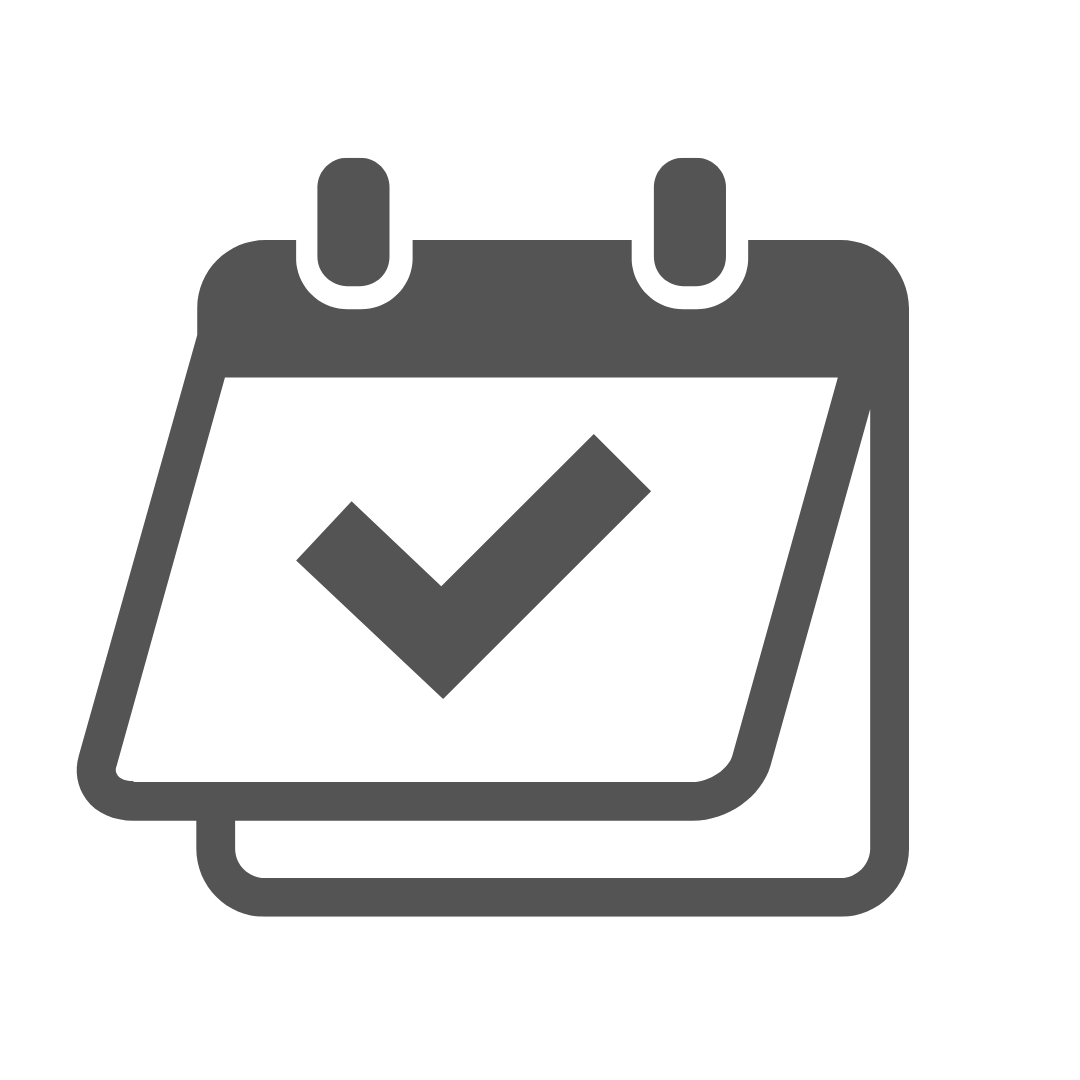
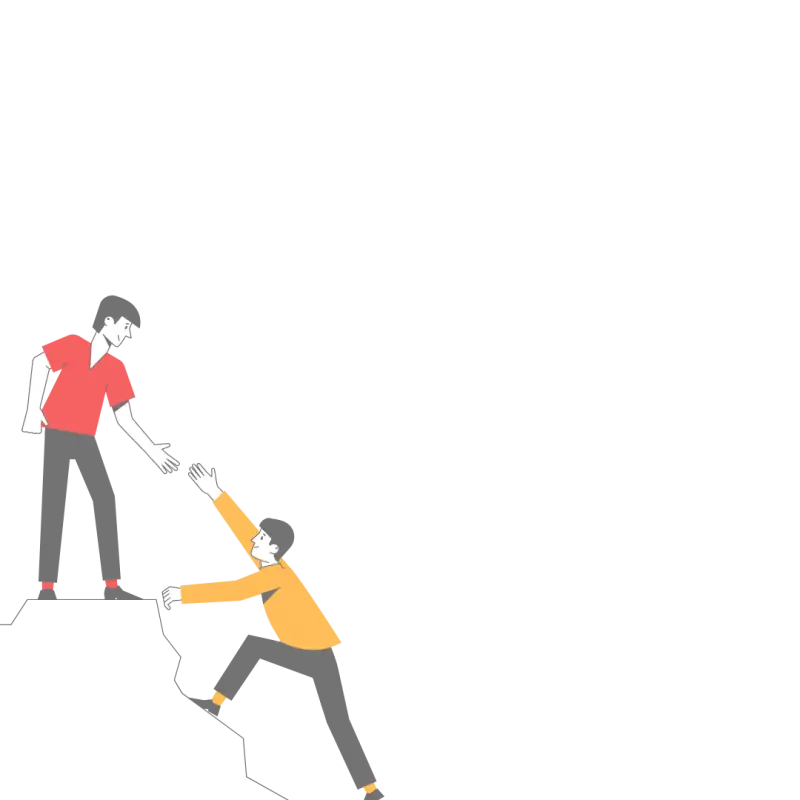
Thanks for your feedback!
Your contributions will help us to improve service.
How can I convert a string to an array in React JS?
In the provided code snippet, a React component is created to convert a string into an array. The component initializes two states: testString
with the value "font awesome icons" and testArray
as an empty array.
The useEffect
hook is used to convert the string into an array using the split
method, which splits the string into an array of substrings based on a specified separator (in this case, a space).
The effect runs only once on mount due to the empty dependency array. The rendered output displays the original string and the converted array using JSON.stringify
..
Output of React Js Convert String to Array
How can I convert a string to an array in React.js by splitting it at each comma?
The provided code is a React.js component that converts a string into an array using the split()
method. The initial value of the testString
state is set to "font,awesome,icons".
When the component mounts, the useEffect()
hook is triggered, splitting the string into an array using the "|" character as the delimiter.
The resulting array is stored in the testArray
state. The rendered output displays the original string and the converted array.
Output of React js convert string to array
How can I convert a string to an array in React.js by splitting it at each newline character ('\n')?
This React.js code converts a string into an array by splitting it at each newline character ("\n").
The code initializes a state variable called testString
with the string "font\n awesome\n icons", and another state variable called testArray
as an empty array.
The useEffect
hook is used to split the string into an array and update the testArray
state variable
How can I convert a string to an array in React.js by splitting it at each pipeline character ('|')?
The provided code snippet demonstrates how to convert a string into an array using React.js. The initial string "font|awesome|icons" is split into an array using the "|" character as the delimiter.
The resulting array is filtered to remove any empty items.
The converted array is stored in the state variable "testArray" using the useState hook. The converted string and array are then displayed on the web page using JSX.