React js Confirm Password Validation
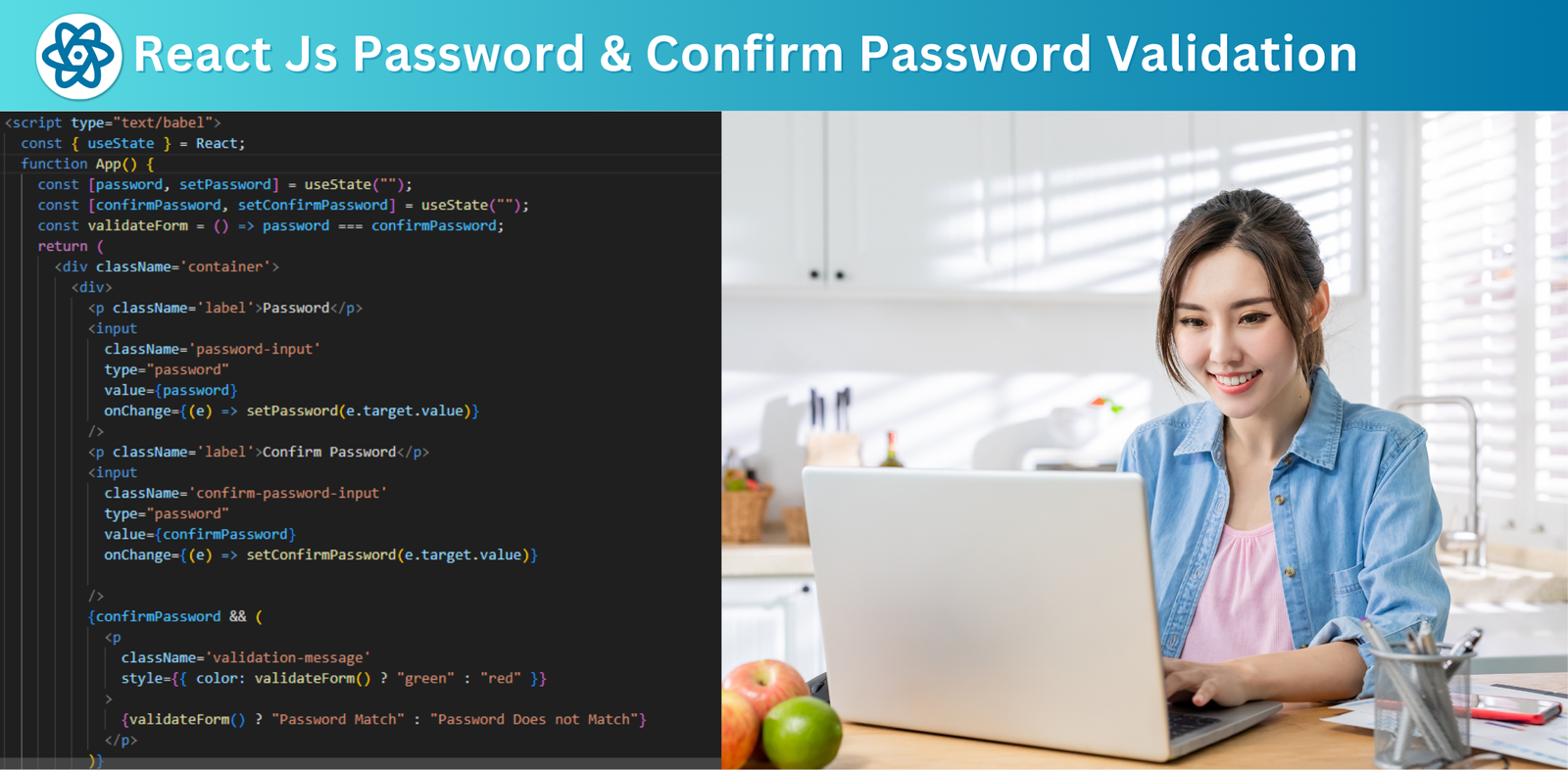
React js Confirm Password Validation: Confirm password validation in React.js involves creating a form with two input fields for password and confirm password, updating the confirm password state variable with an onChange event listener, comparing the password and confirm password fields in the onSubmit function, displaying an error message if they don't match, and disabling the submit button until they match.
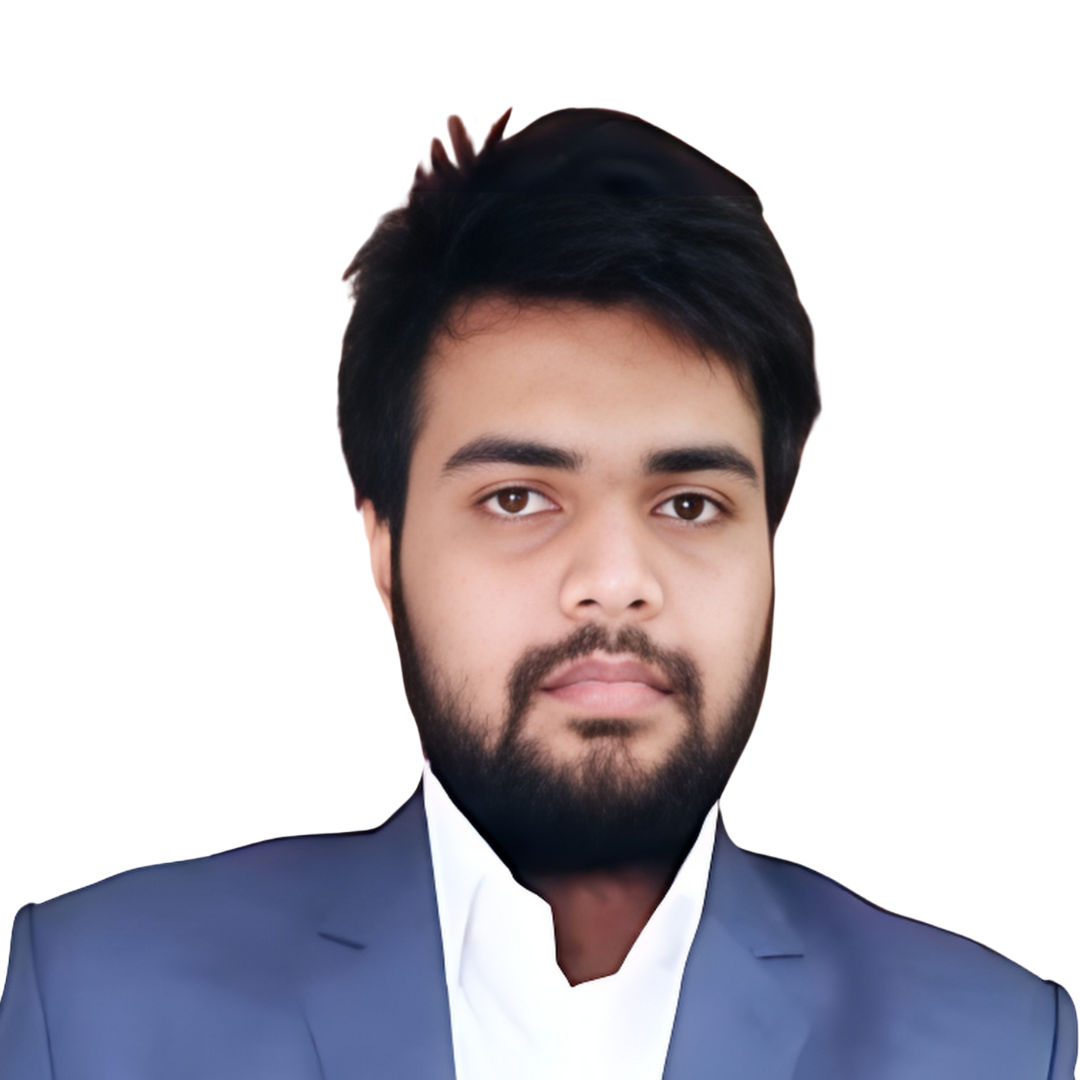
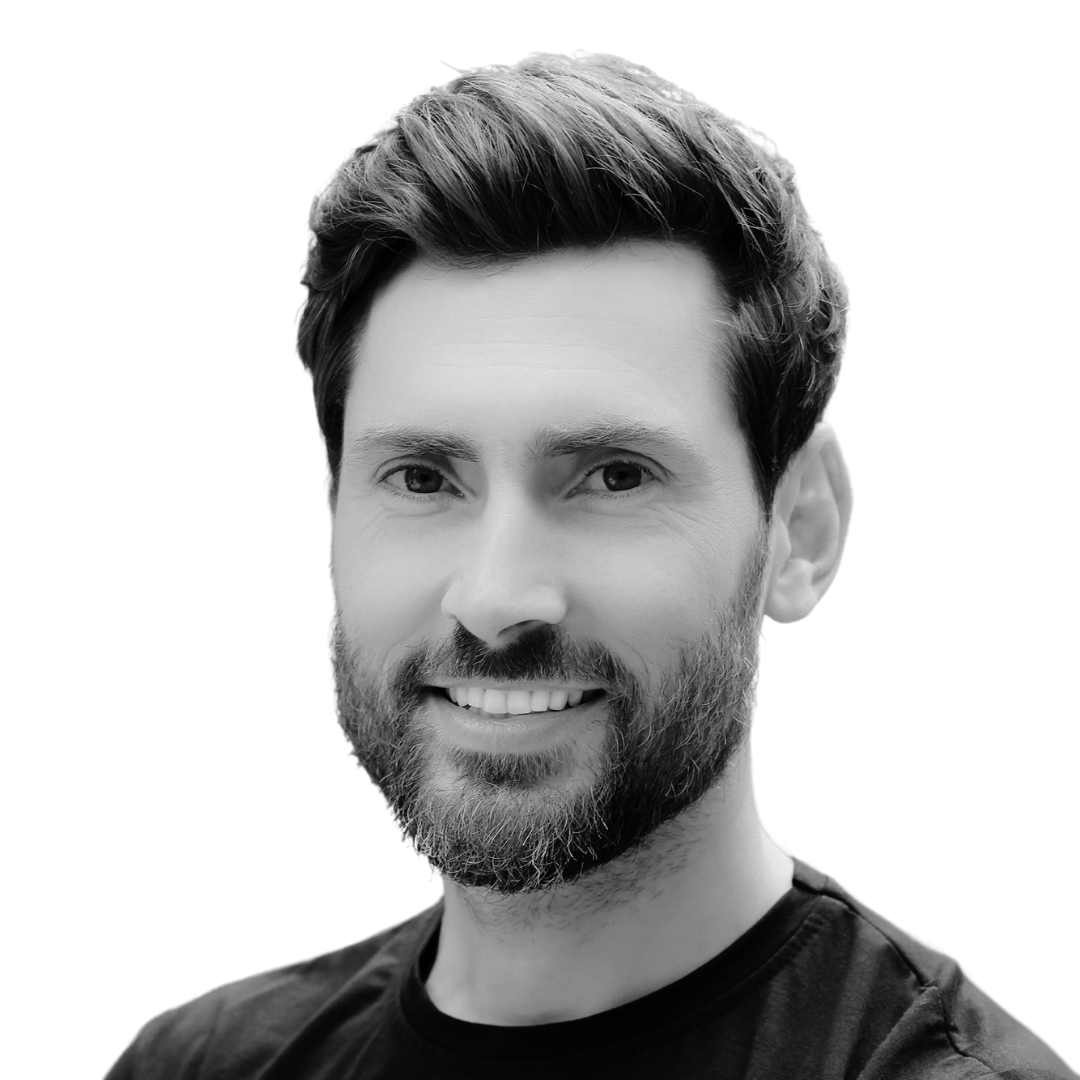
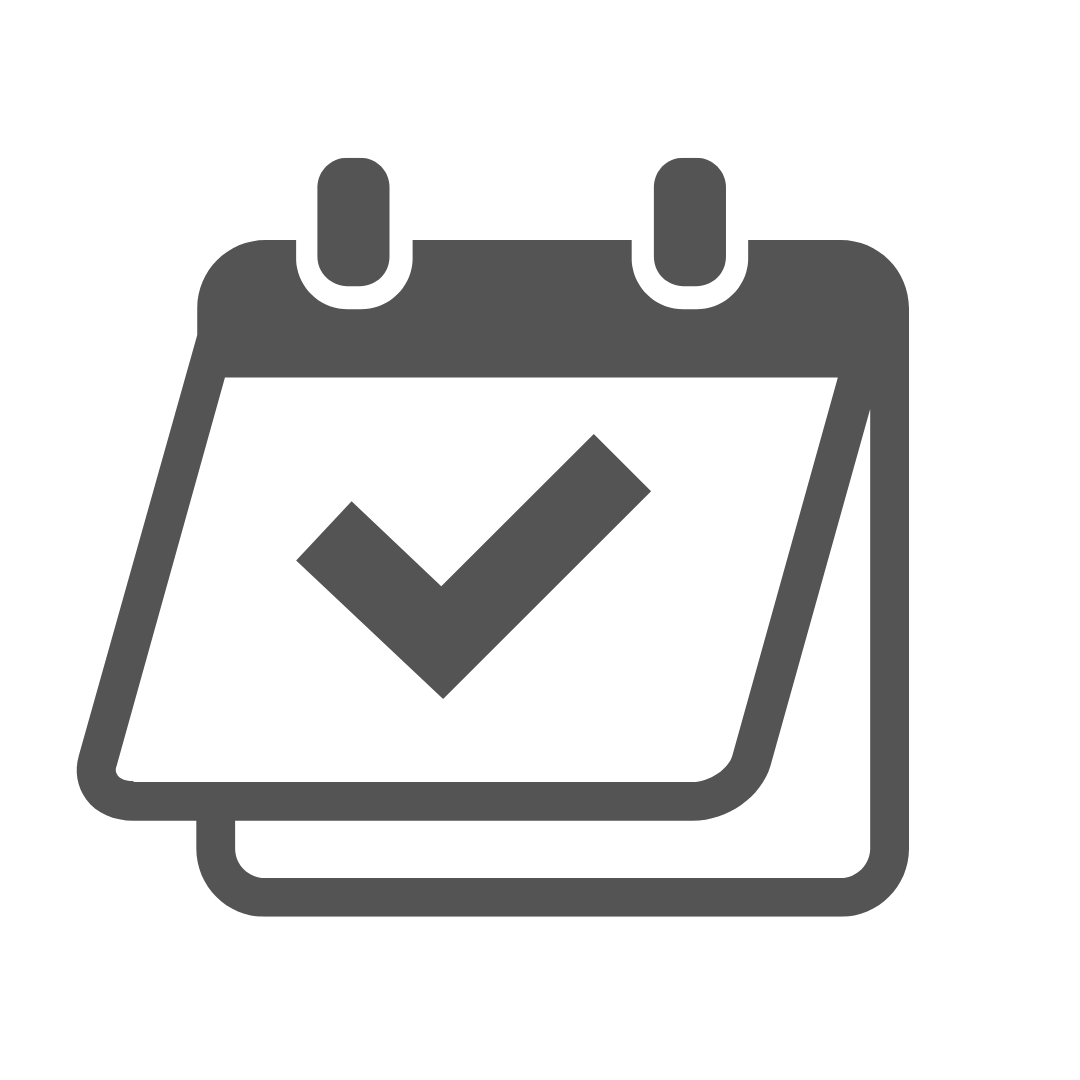
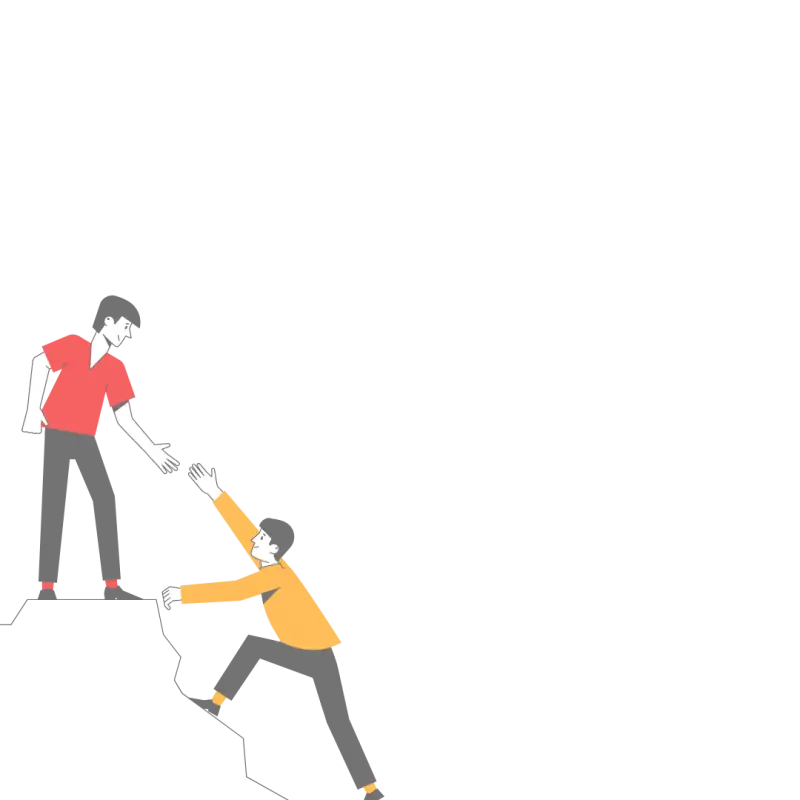
Thanks for your feedback!
Your contributions will help us to improve service.
How do I implement confirm password validation in React.js?
This is a React code that creates a component called App
that renders a form to validate the confirmation of a password. The code uses the useState
hook to create two state variables: password
and confirmPassword
, which represent the values of the two password input fields in the form.
The validateForm
function compares the values of password
and confirmPassword
and returns true
if they match.
The form contains two password input fields, one for the password and one for the confirmation, both of which are bound to their respective state variables using the value
attribute. The onChange
event handler is used to update the state variables whenever the user types into the input fields.
The style
attribute of the confirmation input field is set to change the border color to green or red depending on whether the validateForm
function returns true
or false
.
If the passwords do not match, an error message is displayed below the confirmation password input field using the null
value and a conditional statement to render the error message only when validateForm
returns false
.
The code uses the ReactDOM.render
method to render the App
component to a DOM element with an id
of "app"
. The code also imports React
, useState
, useEffect
, and useRef
from the React library.