React Js Fullscreen Api
React Js Fullscreen Api:The Fullscreen API in React.js enables developers to create fullscreen experiences in web applications. With this API, developers can toggle specific elements, like images or videos, into fullscreen mode, providing users with an immersive viewing experience. React.js provides methods and event handlers, such as requestFullscreen()
, exitFullscreen()
, and the fullscreenchange
event, which can be used to interact with the Fullscreen API.
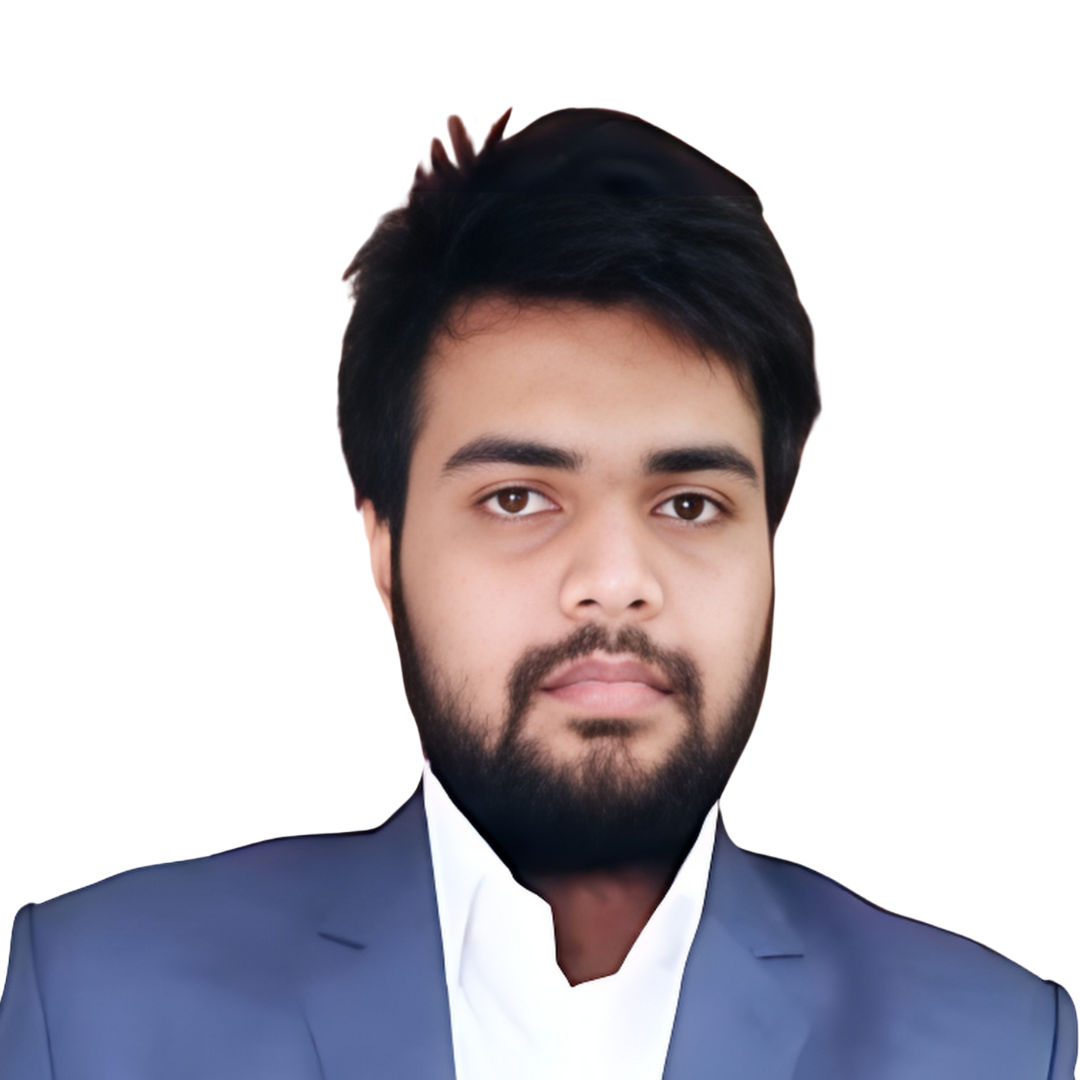
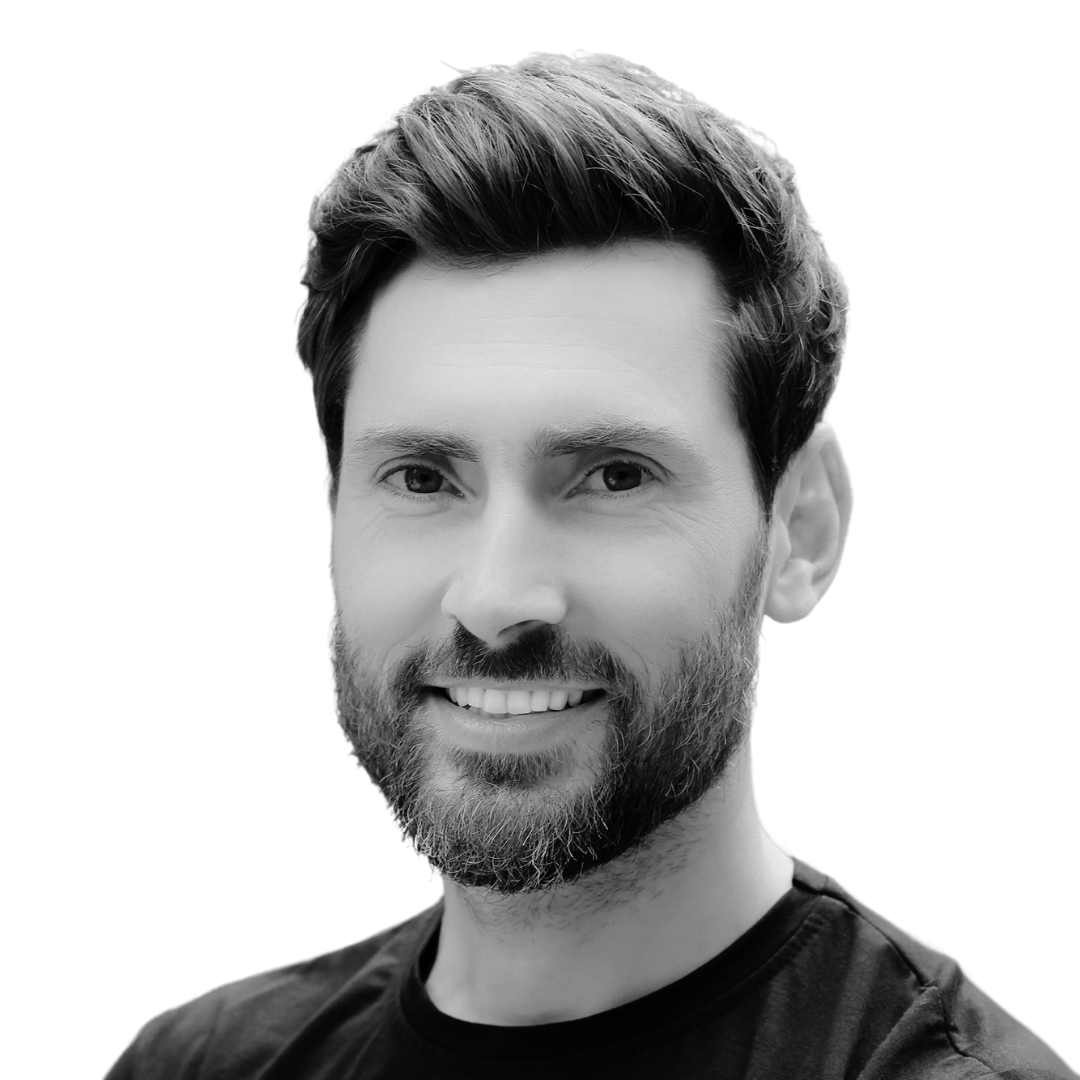
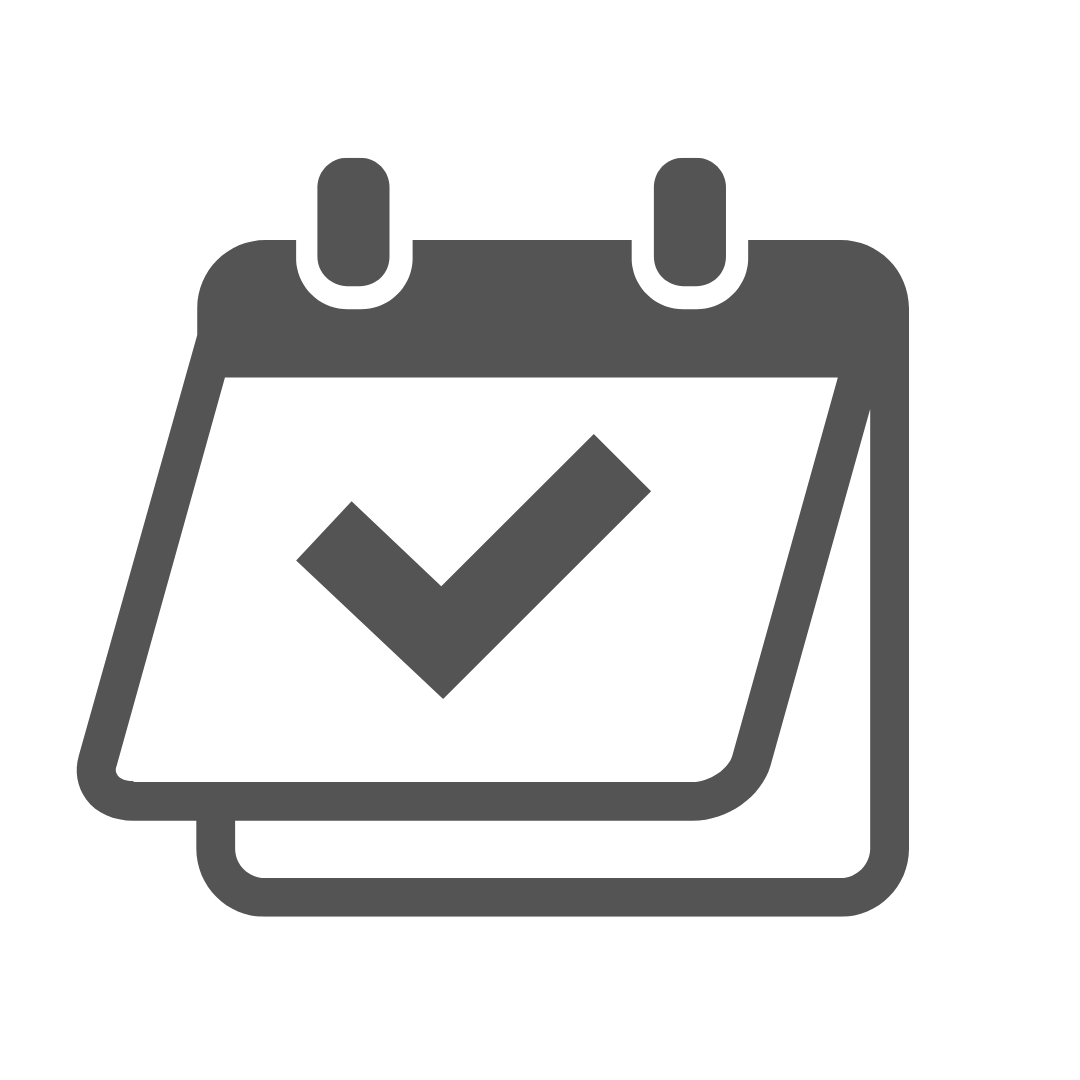
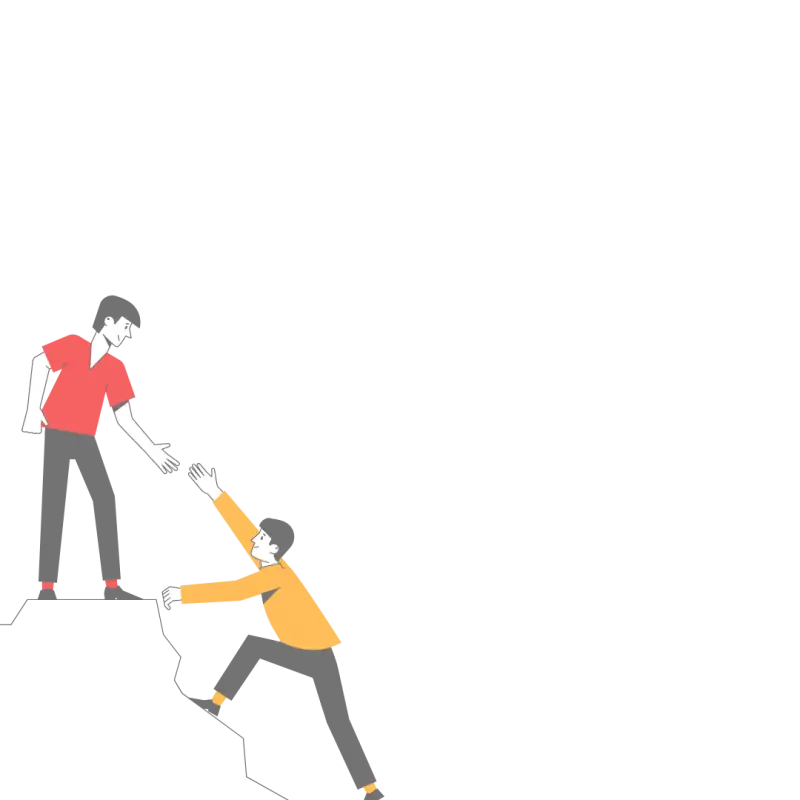
Thanks for your feedback!
Your contributions will help us to improve service.
How can I implement fullscreen mode in a React.js application?
This code snippet demonstrates how to implement a fullscreen feature in a React.js application using the Fullscreen API. By using the requestFullscreen()
and exitFullscreen()
methods, the code toggles the application's fullscreen mode. The useState
and useEffect
hooks are utilized to manage the fullscreen state and handle changes. The rendering part of the code displays a message indicating whether the app is in fullscreen mode or not, along with a button to toggle the fullscreen state.
How can the React.js Fullscreen API be utilized with CSS to create a fullscreen
This code snippet demonstrates how to implement a fullscreen feature in a React.js application using CSS classes. The useState
hook is used to manage the fullscreen state, and the toggleFullscreen
function is called when the button is clicked to toggle the value. Based on the fullscreen state, a CSS class called "fullscreen" is conditionally applied to the container div, which can be styled to adjust the appearance of the app when in fullscreen mode.