React Js Array Push Method
React Js Array Push Method:In ReactJS, you can use the push
method to add values or JSON objects to an array. The push
method is a built-in JavaScript function that appends elements to the end of an array.
In ReactJS, you would typically create a new array or clone the existing array, add the desired values or JSON object using push
, and then update the state using setState
or a state management library like Redux.
This ensures that the component re-renders with the updated array, reflecting the added values or JSON object.
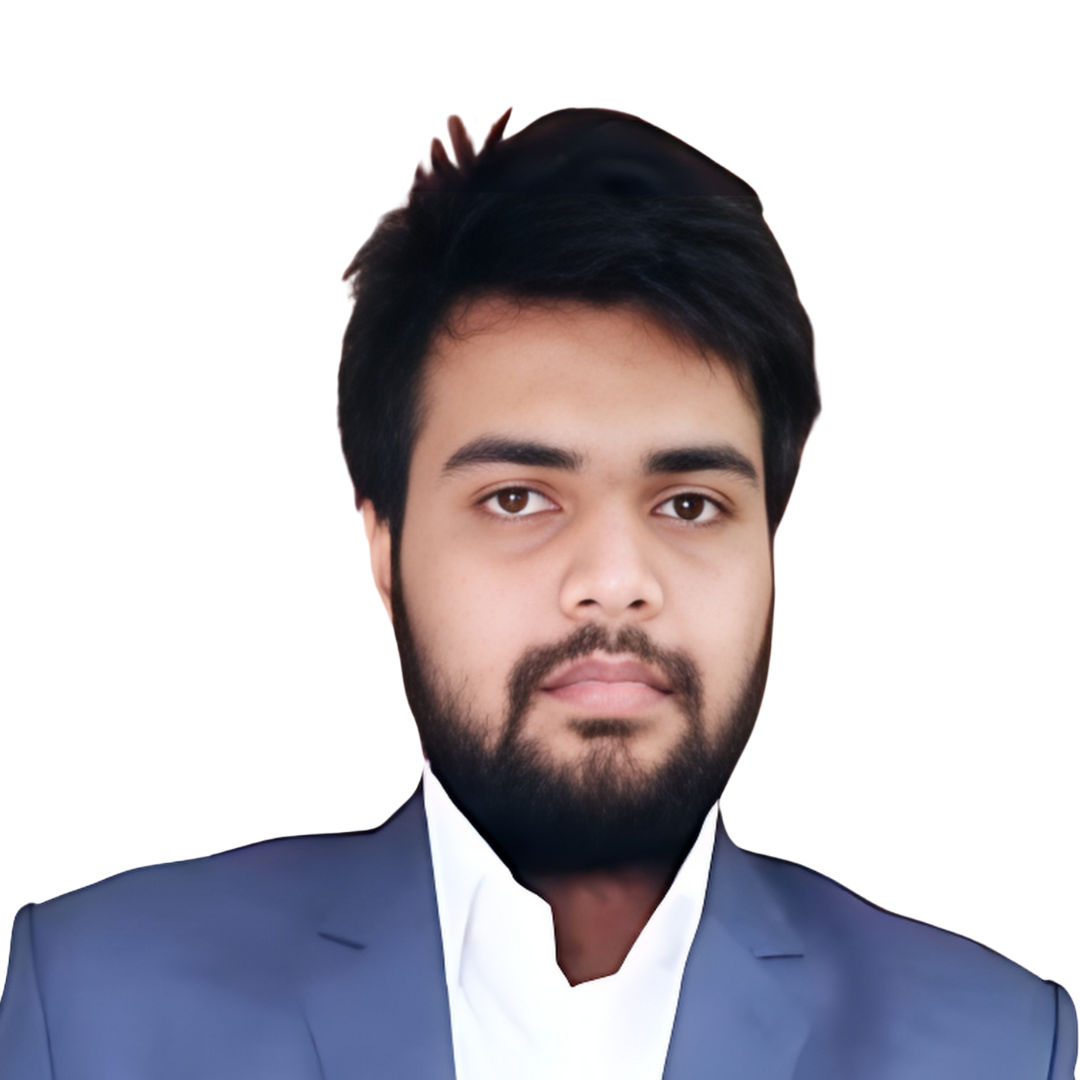
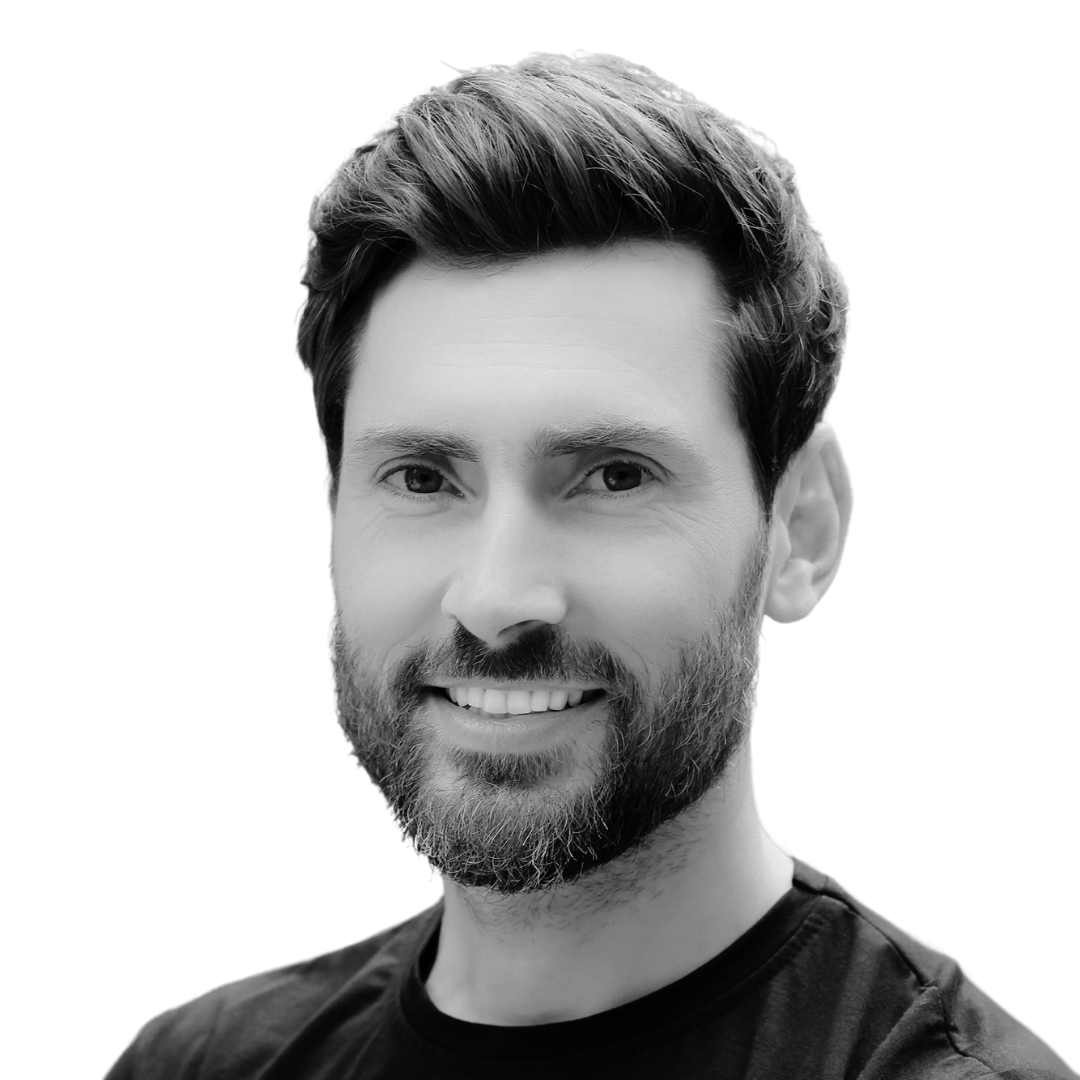
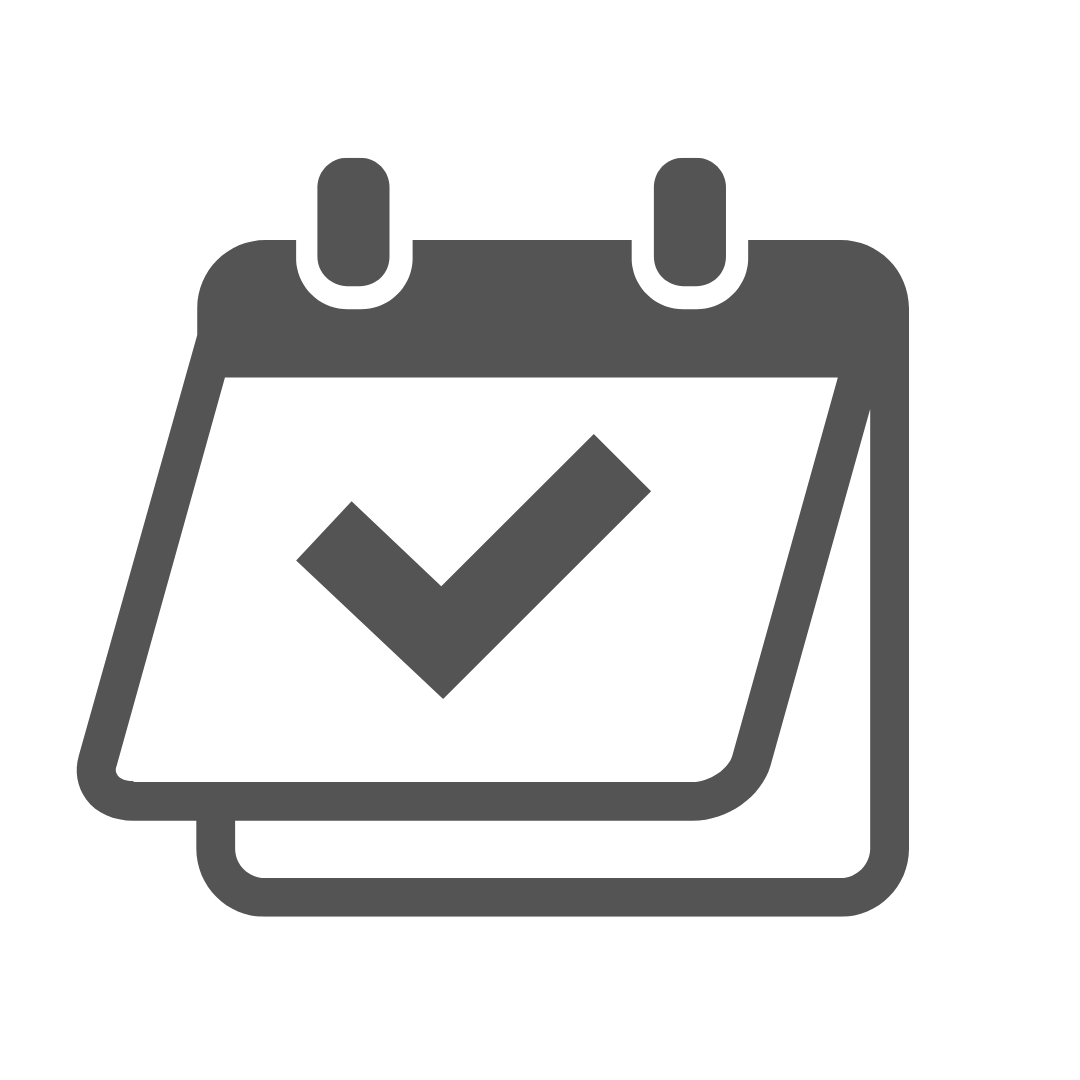
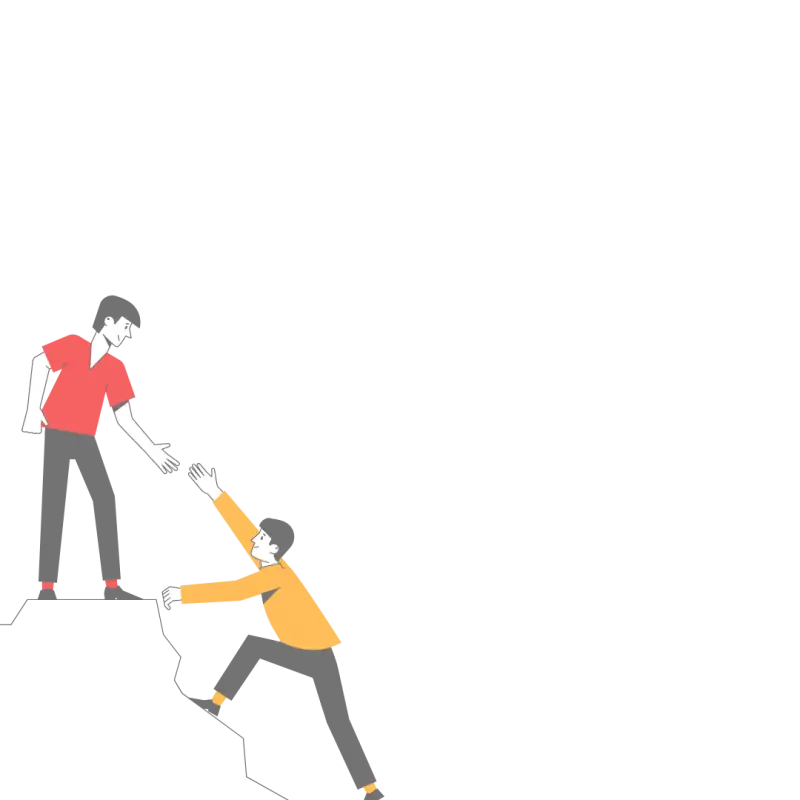
Thanks for your feedback!
Your contributions will help us to improve service.
What is the purpose of the `push` method in React.js when working with arrays?
The code snippet demonstrates the use of the React.js array push method. The initial array, 'myArray', contains two values. When the 'Add Value' button is clicked, the function 'addValueToArray' is executed.
It creates a new array by spreading the existing array and appending a new value, 'Peyush Bansal'. The state is updated with the new array using the 'setMyArray' function.
The updated array is then displayed in an unordered list using the 'map' method.
How can multiple values be added to an array in ReactJS?
In this React.js code snippet, we start by importing the useState
hook from the React library. Inside the functional component App()
, we initialize a state variable called values
using the useState
hook, with an initial array value of [1, 2, 3, 4, 5]
.
The addValues
function is defined to add multiple values to the values
array. Inside this function, a new array called newValues
is created with the values [6, 7, 8, 9, 10]
. Then, using the spread operator (...
), we concatenate the existing values
array with the newValues
array and update the state using setValues
.
In the return statement, we render a button that triggers the addValues
function when clicked. Below the button, an unordered list (<ul>
) is rendered, and each value from the values
array is mapped to a list item (<li>
) using the map()
function.
How can I add a JSON object to an existing array in ReactJS?
This code snippet demonstrates how to add a JSON object to an array in React.js. The App
component initializes a state variable called items
as an empty array using the useState
hook.
The addItem
function is used to add a new JSON object to the items
array. Each object has an id
, name
, and quantity
property. When the "Add Item" button is clicked, a new object is created with a unique ID, the name "Example," and a quantity based on the current length of the items
array.
The setItems
function is used to update the state by appending the new object to the existing array. The rendered output displays the list of items with their respective names and quantities