React Js Fetch Data from API | load data without reloading page
React Js Fetch Data from API:React.js provides multiple options to fetch data from an API, including the fetch() method and the axios library.
The fetch() method is built into modern browsers and offers a simple way to make HTTP requests. It returns a Promise that can be handled using the .then() and .catch() methods.
On the other hand, axios is a third-party library that simplifies HTTP requests by providing a more intuitive and feature-rich API.
React JS allows developers to fetch data from APIs without reloading the entire page using asynchronous calls. By leveraging technologies like fetch()
or libraries like Axios, React can retrieve data from an API in the background.
The retrieved data is then used to update the components dynamically, rendering new content without requiring a full page refresh.
This approach ensures a smooth user experience, as only the relevant parts of the page are updated with the latest information from the API respons.
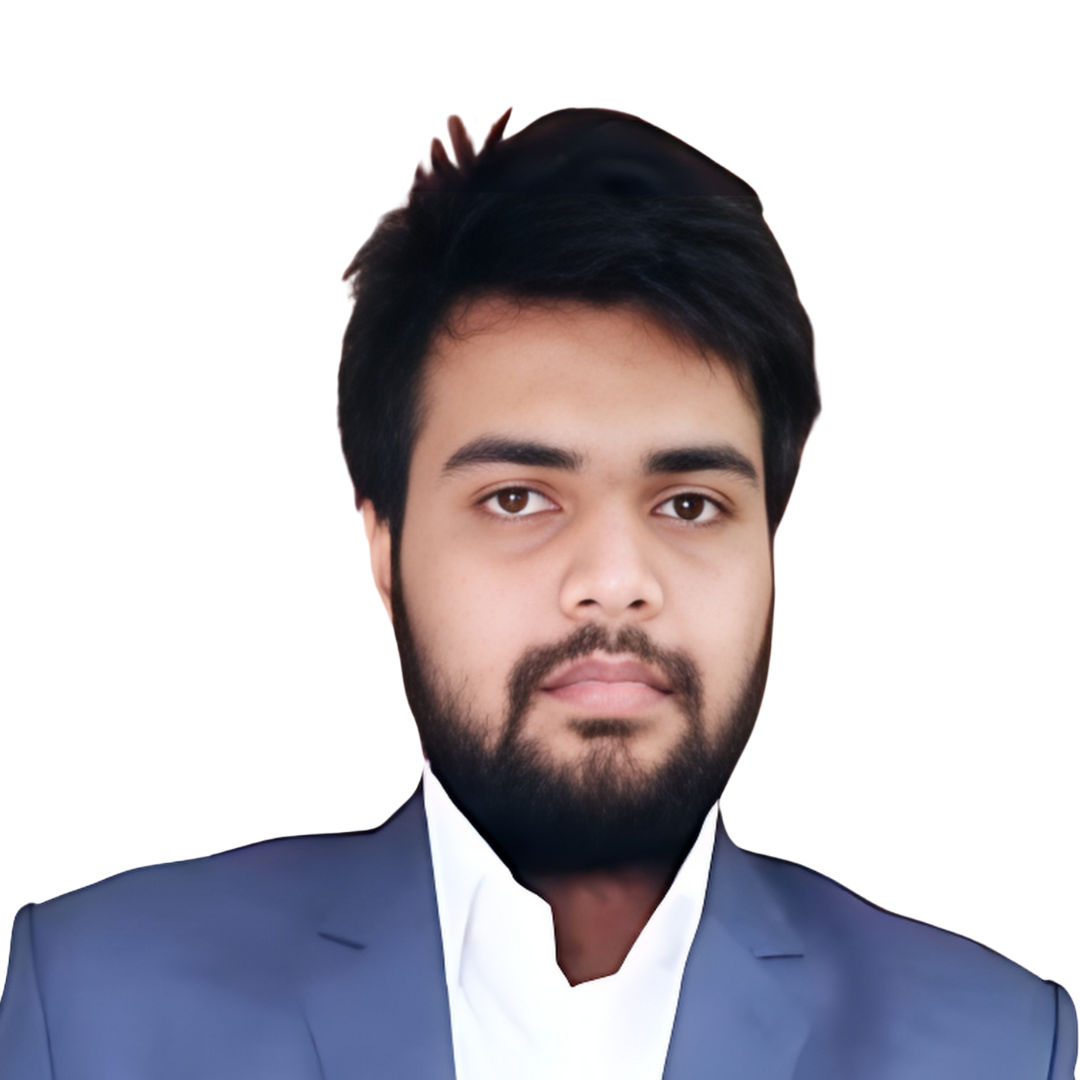
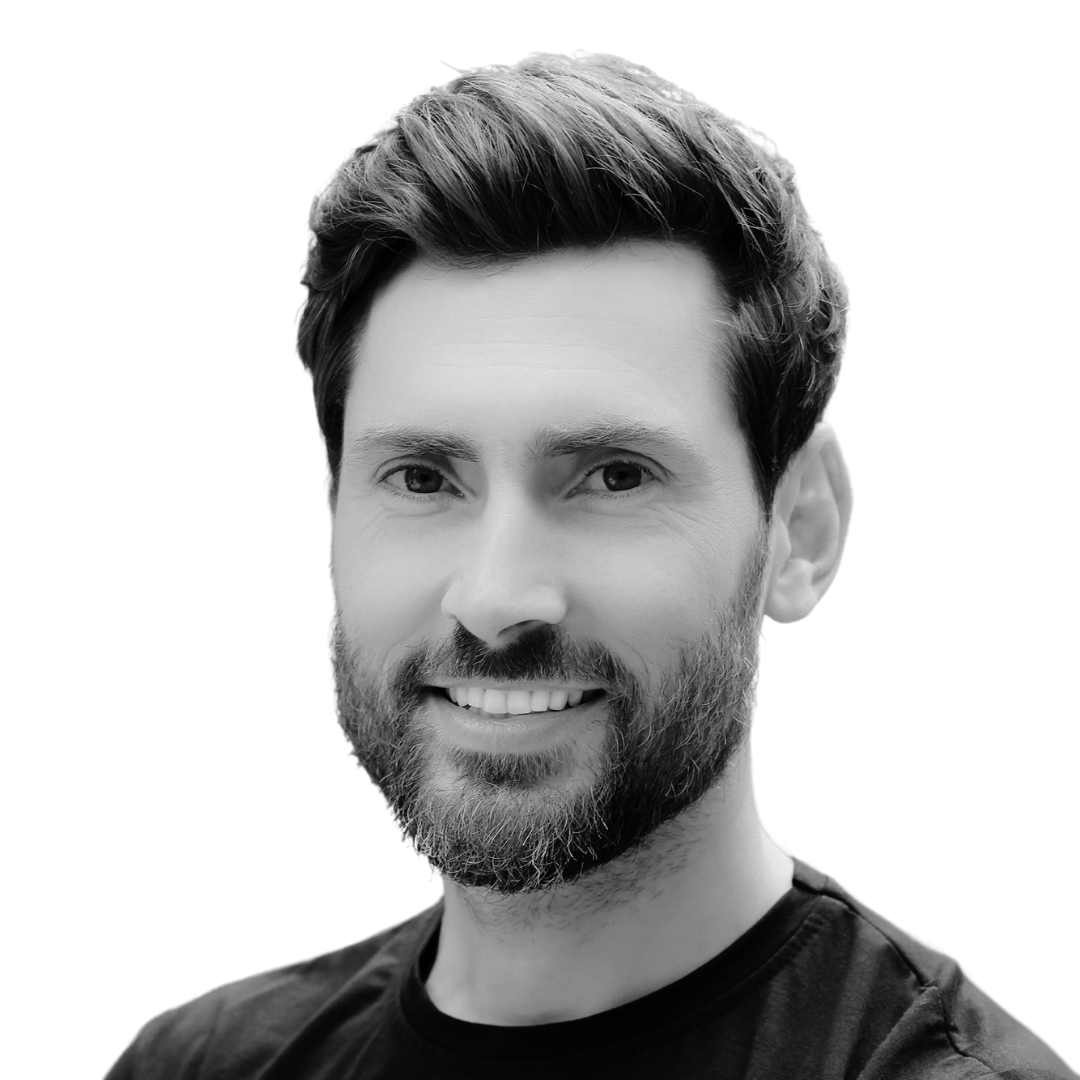
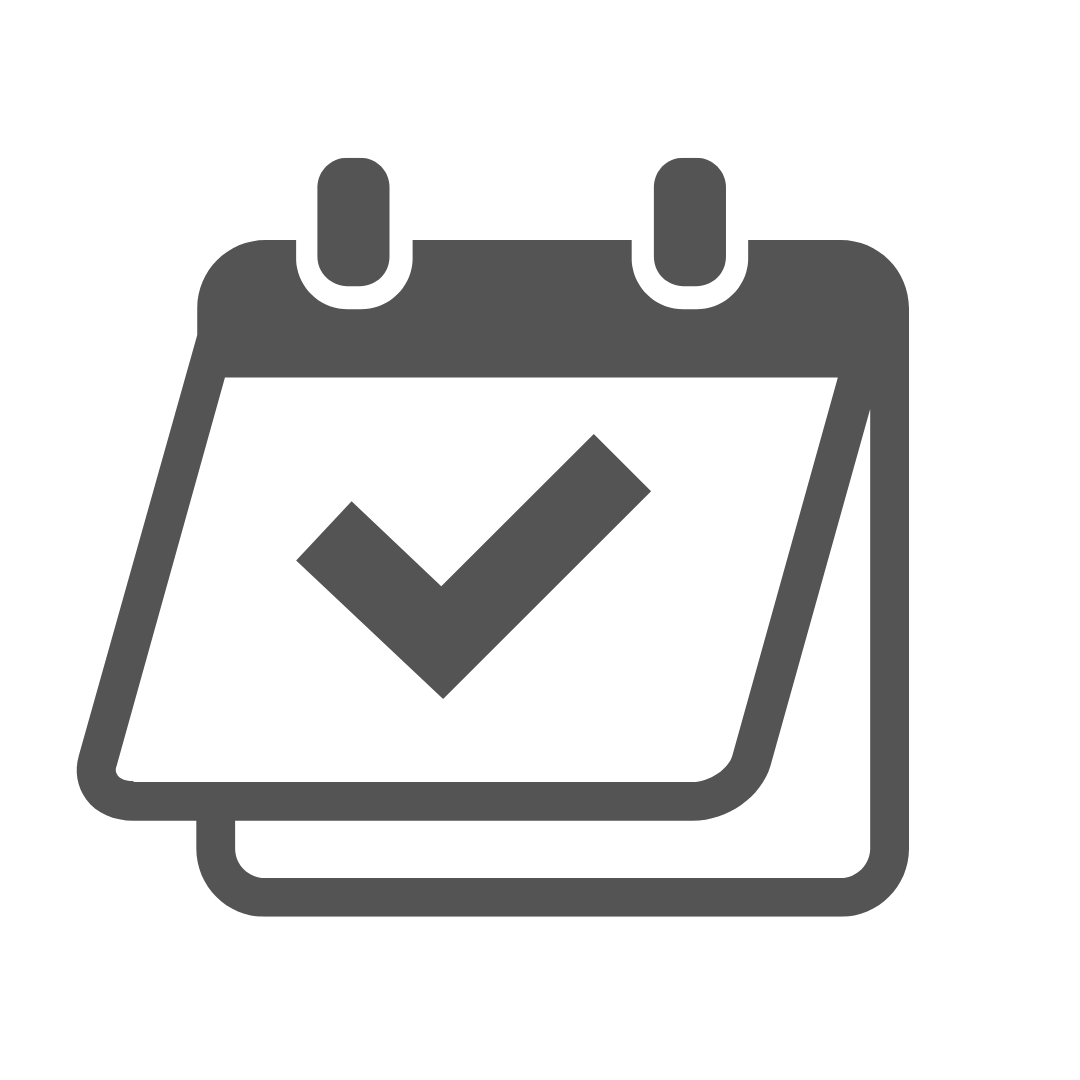
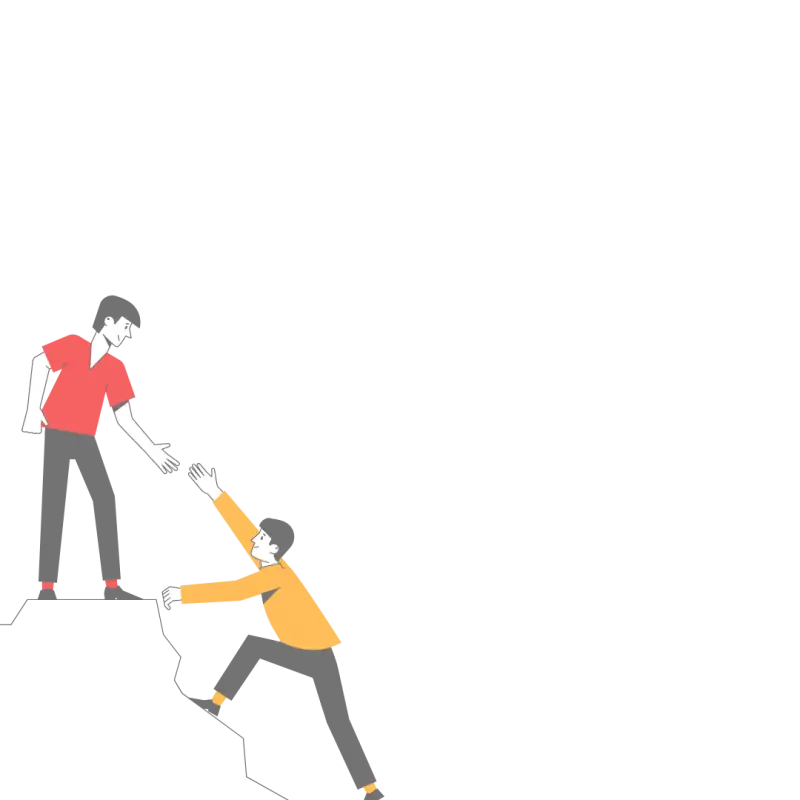
Thanks for your feedback!
Your contributions will help us to improve service.
How can I use the fetch()
Method in React.js to retrieve data from an external API?
This code snippet demonstrates how to fetch data from an API using the fetch()
method in React.js. It defines a functional component called App
that uses the useState
and useEffect
hooks.
Inside the useEffect
hook, the fetch()
function is used to retrieve data from the specified API endpoint. If the response is successful, the data is parsed and stored using the setData
function. If there is an error, it is caught and stored using the setError
function.
The component then conditionally renders the error message or a loading indicator while waiting for the data to load. Once the data is available, it is rendered in a table format.
Output of React Js Fetch Data from API
How can you fetch data from an API using Axios in React.js?
This code snippet demonstrates how to use Axios in React.js to fetch data from an API. It starts by importing the necessary dependencies and setting up the App
component. Inside the component, it defines two state variables: data
and error
.
The useEffect
hook is used to make an HTTP GET request to the specified API endpoint using Axios. If the request is successful, the data is stored in the data
state variable. If an error occurs, the error message is stored in the error
state variable.
The component then renders different content based on the state. If there is an error, it displays an error message. If the data is still loading, it displays a loading message.
Finally, if the data is available, it renders a table with the fetched data, mapping over the data
object to generate rows for each currency and its corresponding rate.
How can I use Reactjs to load data without reloading the page and automatically update the data when changes occur?
The given Reactjs code uses the useState
, useEffect
, and axios
libraries to load data from the Coindesk API without reloading the page. It fetches cryptocurrency rates every 6 seconds, updates the state, and displays the data in a table. A toast notification is shown when the data is successfully reloaded, and it automatically disappears after 3 seconds. The code sets up an interval to periodically fetch and update the data, ensuring that the page displays the latest cryptocurrency rates without any manual refresh.
Output of React Js load data without reloading page
How can you handle HTTP requests using async/await in Reactjs with an API example?
This Reactjs code uses async/await and the Axios library to handle an HTTP request to the 'https://api.coindesk.com/v1/bpi/currentprice.json' API. It fetches Bitcoin price data, displaying it in a table with currency and rate information. The code initializes state variables for data and error handling. The useEffect hook ensures the API call is made when the component mounts. If successful, it populates the data state; otherwise, it sets the error state. The UI displays "Loading..." while fetching and renders the data in a table when available.