React Js focus Next input field on Enter
React Js focus Next input field on Enter:In React.js, to focus the next input field when the "Enter" key is pressed, you can utilize the onKeyDown
event handler.
First, assign a ref
to each input field using the useRef
hook. Then, in the event handler, check if the pressed key is "Enter" (key code 13). If it is, use the current
property of the next input field's ref
to focus it.
Keep in mind that you may need to handle edge cases, such as the last input field in the form. In summary, with proper event handling and the ref
concept, you can achieve the desired functionality in React.js.
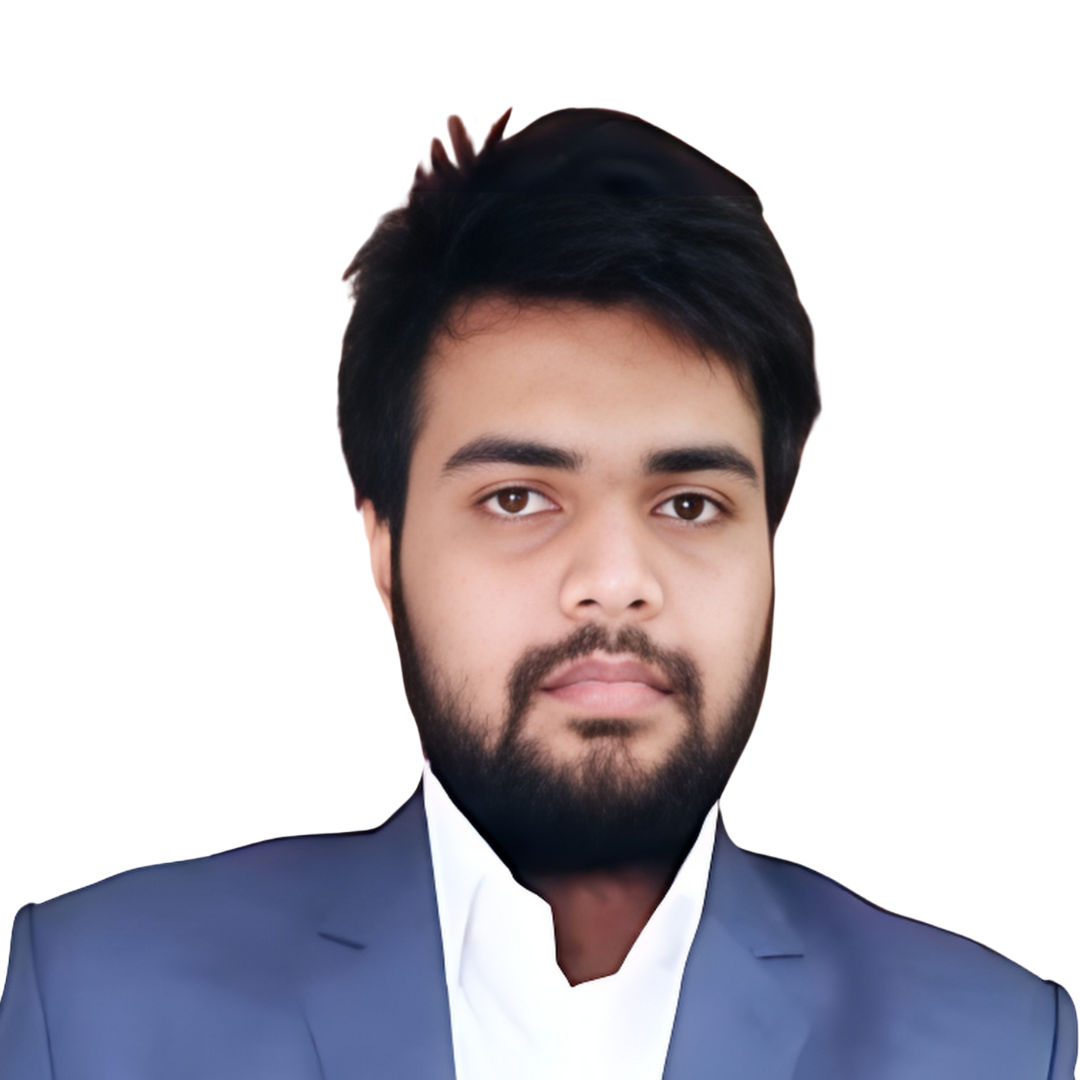
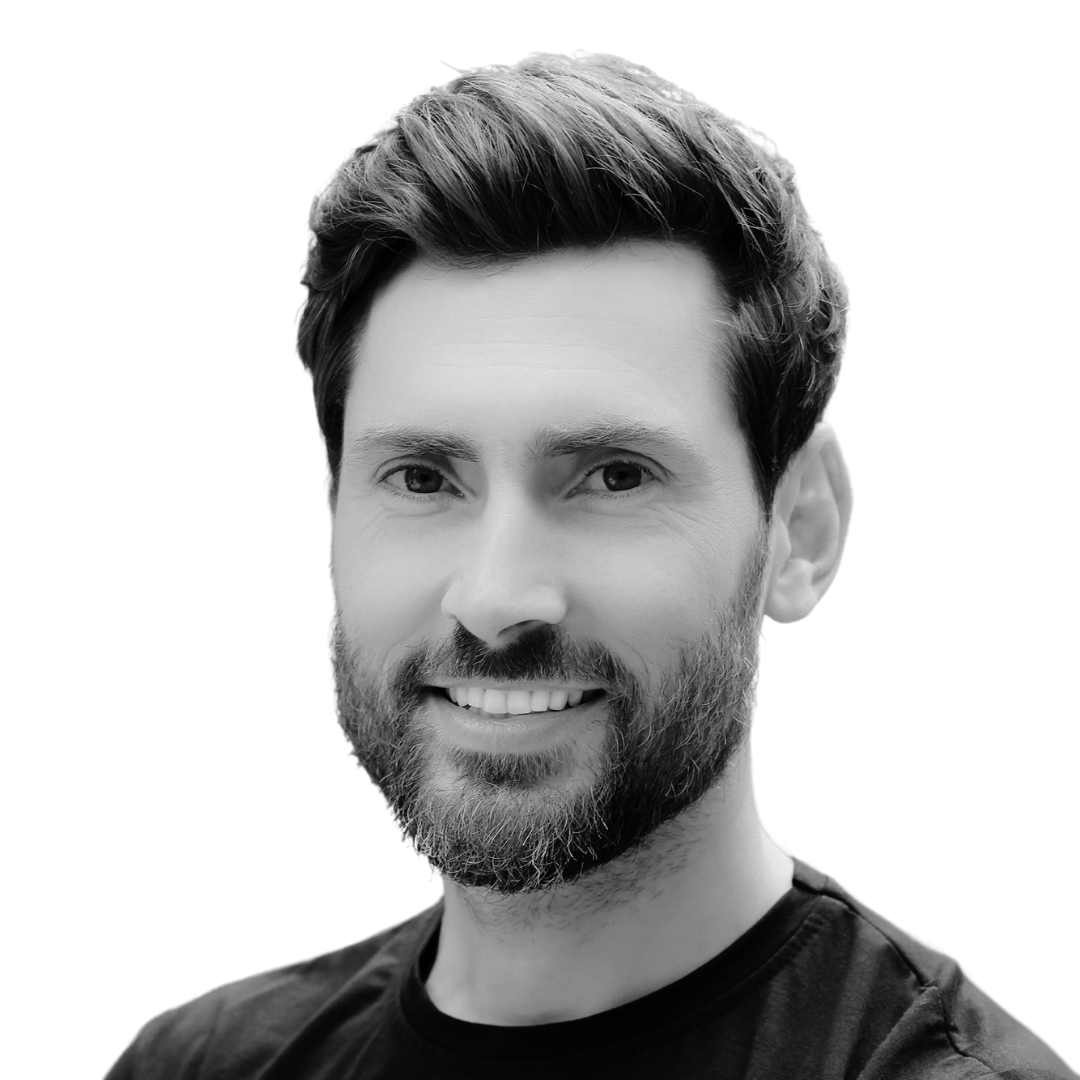
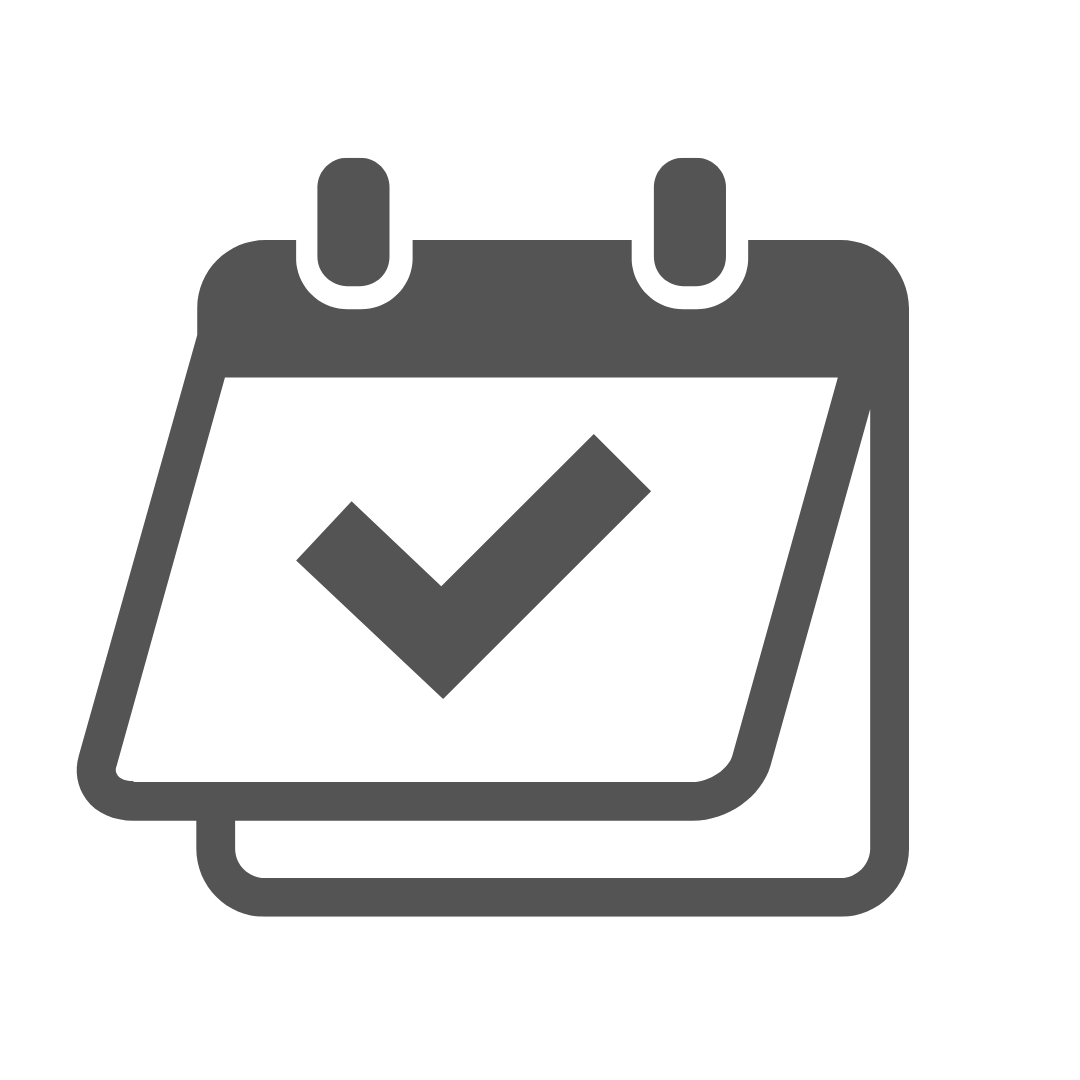
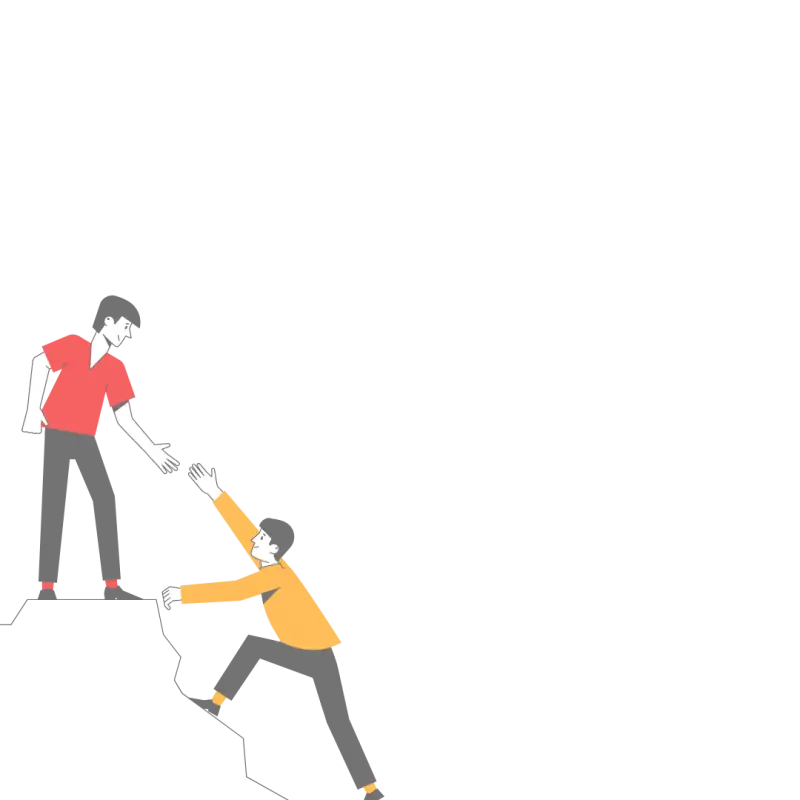
Thanks for your feedback!
Your contributions will help us to improve service.
How can you use React.js to automatically focus on the next input field when the "Enter" key is pressed?
This code snippet demonstrates how to implement a functionality in ReactJS that focuses on the next input field when the "Enter" key is pressed. It starts by using the useRef
hook to create a reference to the input fields.
The handleKeyDown
function is called when a key is pressed within an input field. If the pressed key is "Enter," it prevents the default behavior and calculates the index of the next input field. If there is a next input field, it sets the focus on it using the focus()
method. Otherwise, it sets the focus on the first input field.
The handleInputChange
function can be used to handle any input change logic. In this example, it logs the input value to the console.
The addInputRef
function adds the input field reference to the inputRefs
array. If the reference is not already present, it adds it to the array and assigns the handleKeyDown
function to the input field's onkeydown
event if it's the last input field.
Finally, the App
component renders a container with three input fields. Each input field has a reference assigned and an onChange
event handler that calls the handleInputChange
function.
When the code is executed, pressing "Enter" in an input field will shift the focus to the next input field, and if the last input field is reached, it will cycle back to the first input field.
React Js focus Next input field on Enter Example
xxxxxxxxxx
<script type="text/babel">
const { useRef } = React
function App() {
const inputRefs = useRef([]);
const handleKeyDown = (e, index) => {
if (e.key === 'Enter') {
e.preventDefault();
const nextIndex = index + 1;
if (nextIndex < inputRefs.current.length) {
inputRefs.current[nextIndex].focus();
} else {
inputRefs.current[0].focus(); // Focus on the first input field
}
}
};
const handleInputChange = (e, index) => {
// Handle your input change logic here
console.log(e.target.value);
};
const addInputRef = (ref, index) => {
if (ref && !inputRefs.current.includes(ref)) {
inputRefs.current.push(ref);
if (index === inputRefs.current.length - 1) {
ref.onkeydown = (e) => handleKeyDown(e, index);
}
}
};
return (
<div className='container'>
<h3>React Js focus Next input field on Enter</h3>
<input
ref={(ref) => addInputRef(ref, 0)}
onChange={(e) => handleInputChange(e, 0)}
/><br />
<input
ref={(ref) => addInputRef(ref, 1)}
onChange={(e) => handleInputChange(e, 1)}
/><br />
<input
ref={(ref) => addInputRef(ref, 2)}
onChange={(e) => handleInputChange(e, 2)}
/>
</div>
);
}
ReactDOM.render(<App />, document.getElementById("app"));
</script>