React Js Generate Random Number
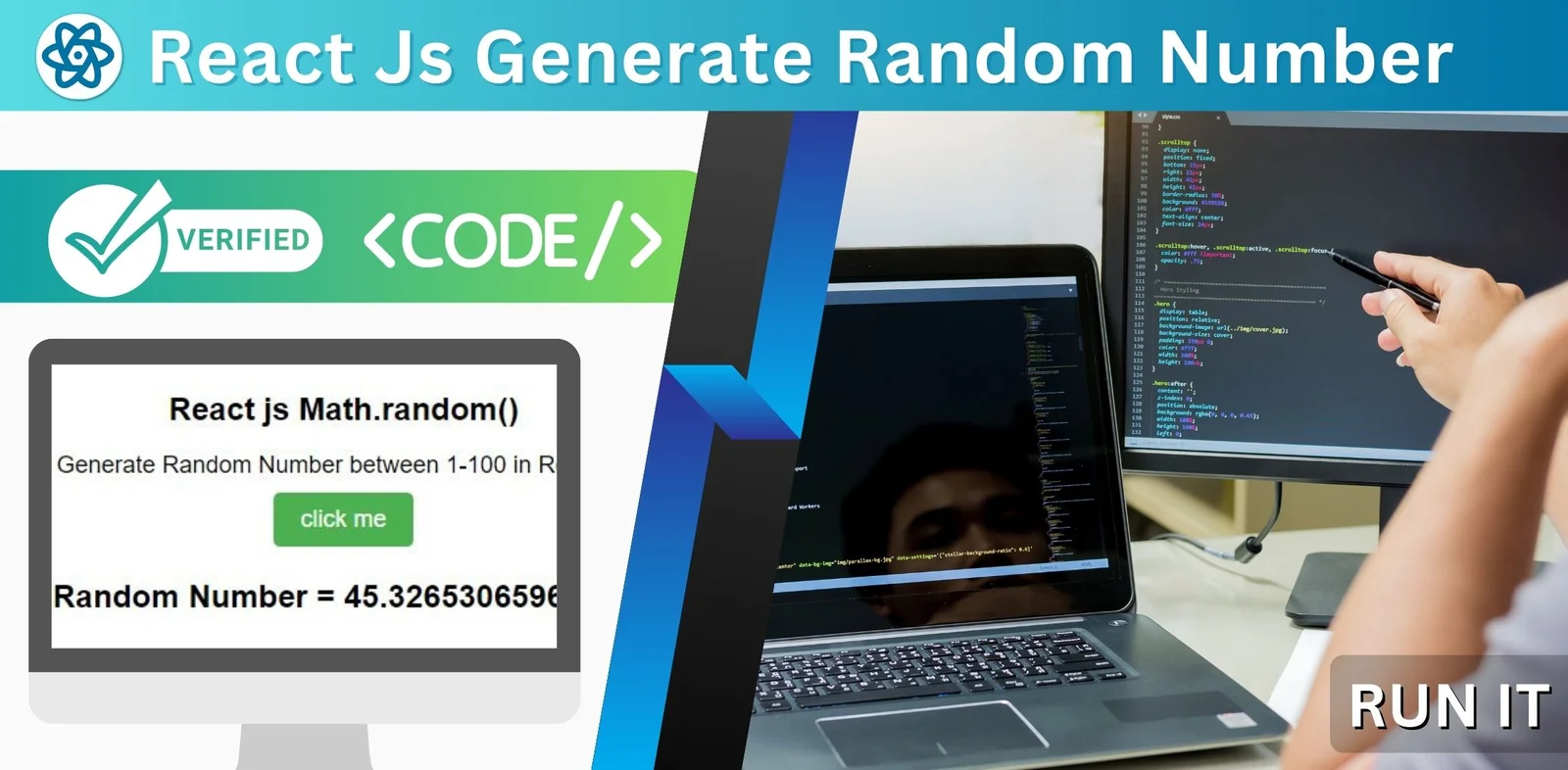
React Js Generate Random Number: In ReactJS, generating a random number can be done by using the Math.random()
method, which generates a random decimal between 0 and 1. To get a random integer within a specific range, we can multiply the result of Math.random()
by the desired range and then use the Math.floor()
method to round down to the nearest integer.
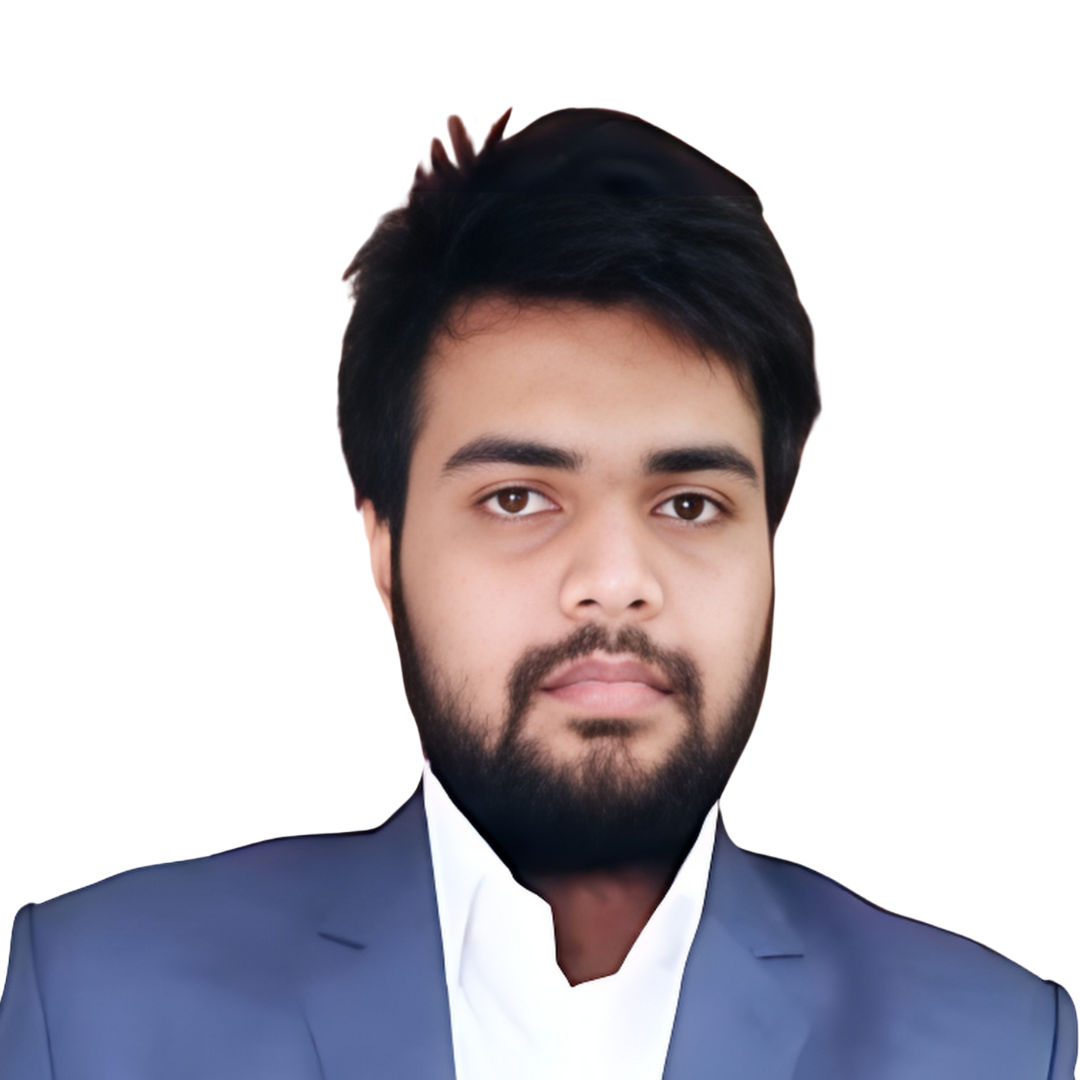
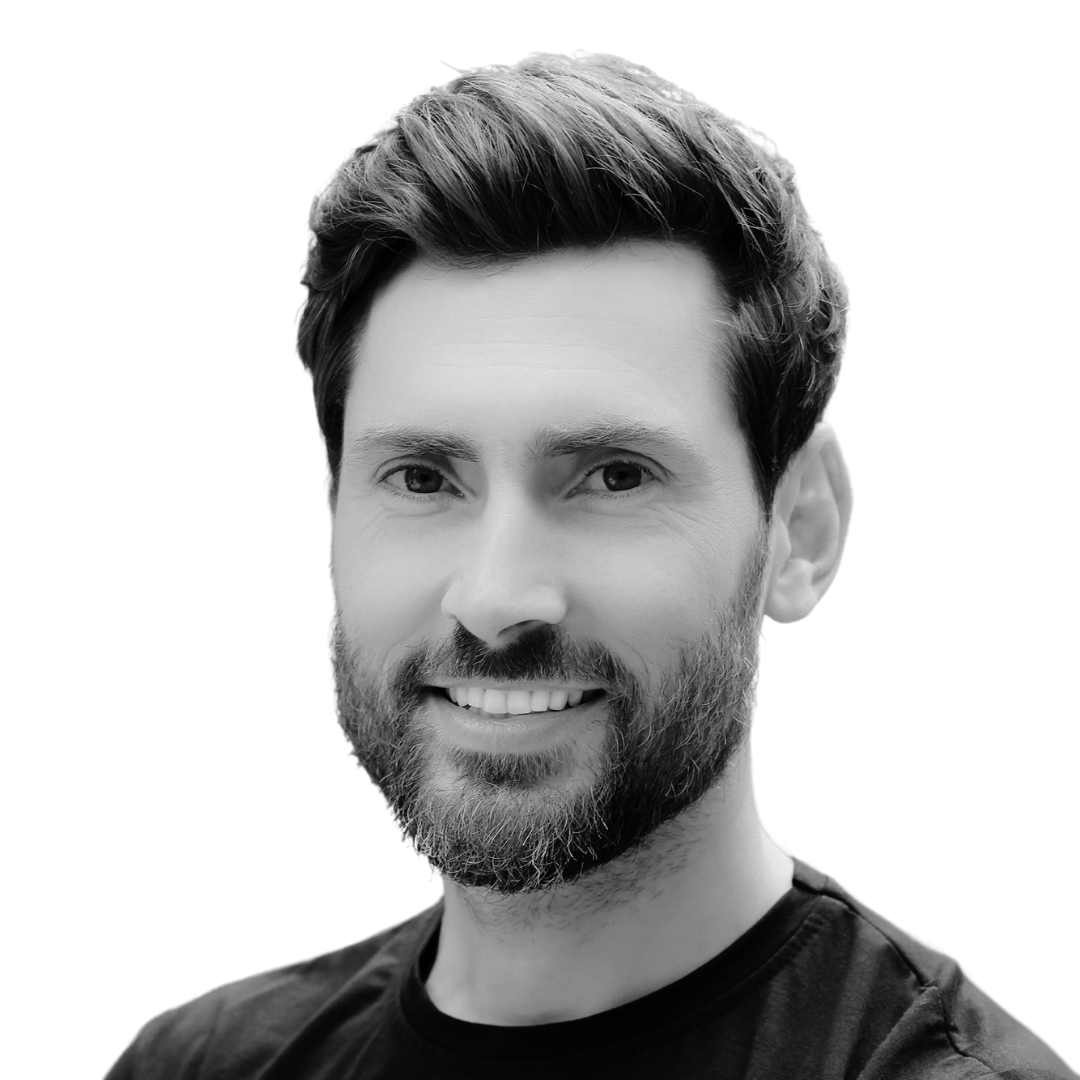
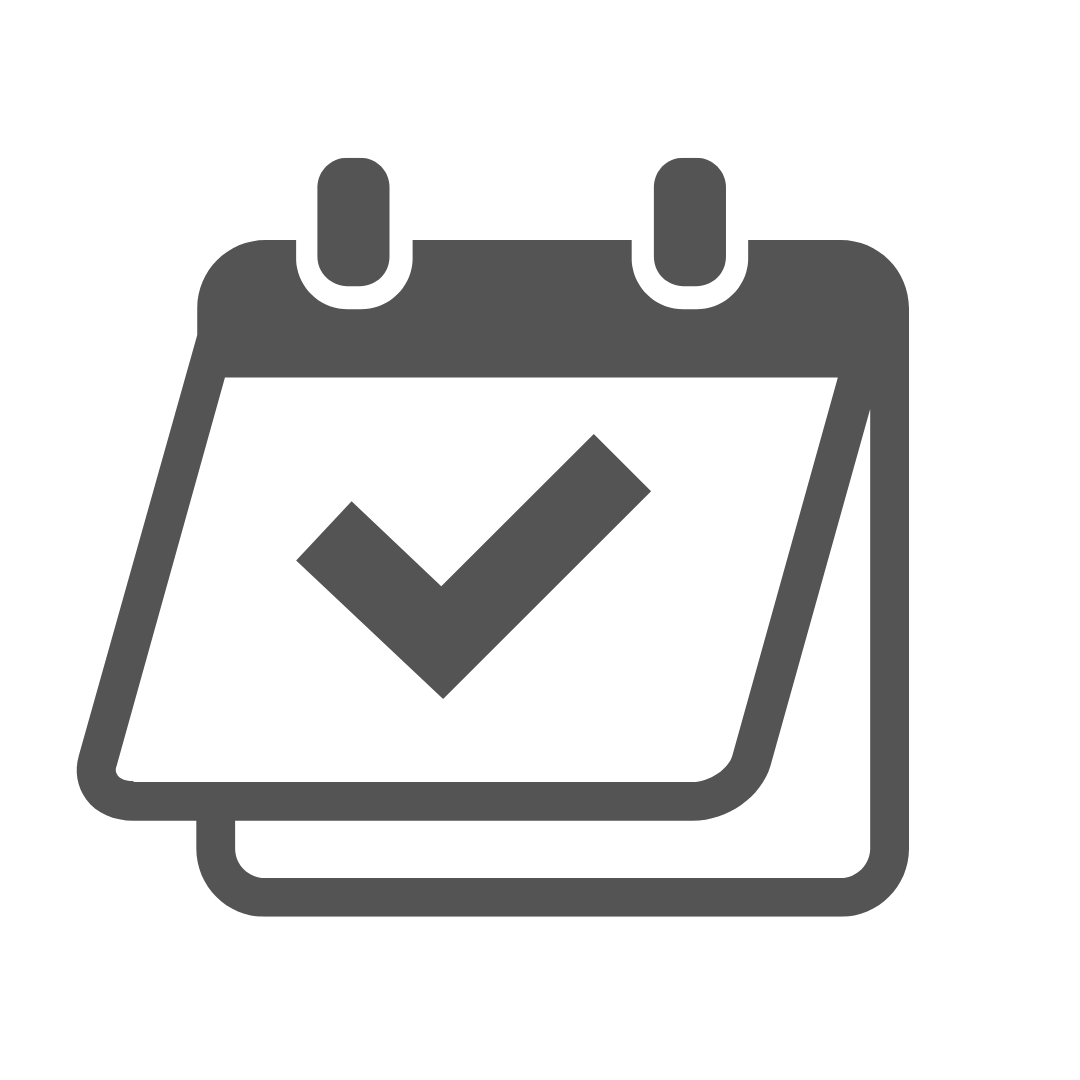
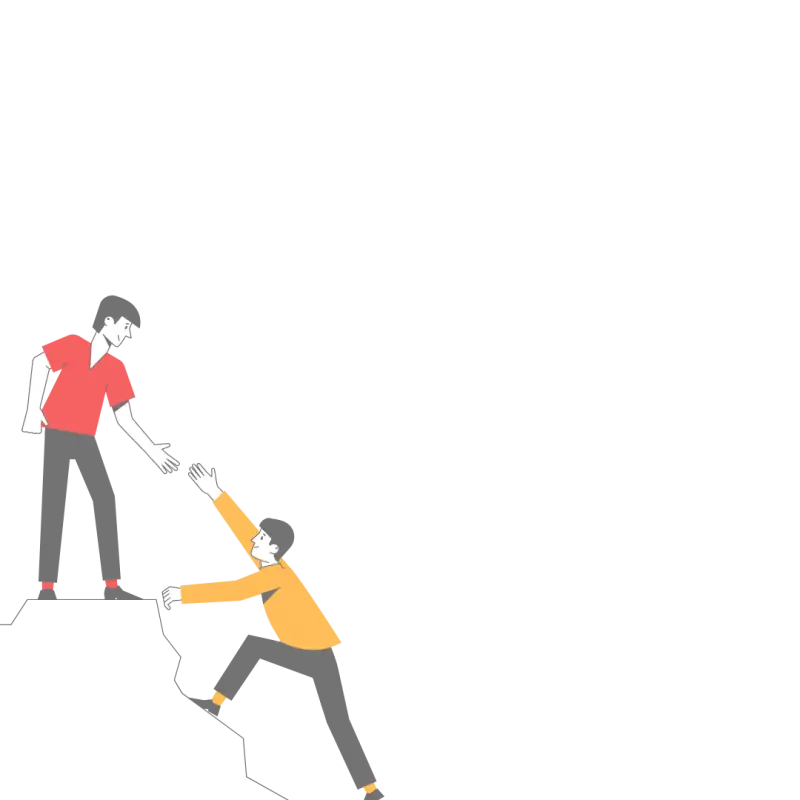
Thanks for your feedback!
Your contributions will help us to improve service.
How can I generate a random number in a React JS component?
This code snippet shows a React component that generates a random number between 0 and 100 when a button is clicked, and displays it on the screen using the useState hook.
The useState hook is used to create a state variable called randomNumber and a function called setRandomNumber that can be used to update its value. Initially, the randomNumber state variable is set to an empty string.
When the button is clicked, the myFunction function is called, which updates the randomNumber state variable to a new random number between 0 and 100 using the Math.random() method.
The updated value of randomNumber is then displayed on the screen using the {randomNumber} expression within the paragraph tag.
Note that this code requires the Babel transpiler to convert the JSX syntax into regular JavaScript code that can be run in the browser.
Output of React Js Generate Random Number
How can I generate a random number between 50 and 100 in a Reactjs?
This React JS code defines a functional component called App
. It utilizes the useState
hook to manage a state variable called randomNum
. When the "Generate" button is clicked, the generateRandomNumber
function is called. This function calculates and sets a random number between 50 and 100 (inclusive) to the randomNum
state using Math.random()
and Math.floor()
. The rendered component displays a title, the generated random number, and a button to trigger the number generation. This simple React app allows users to generate and display random numbers between 50 and 100 by clicking the "Generate" button.
Output of React Js Generate Random Number Between 50-100
How can I generate a random number between 1 and 1000 in Reactjs without allowing any repetition?
This Reactjs code generates random numbers between 1 to 1000 without repetition. It uses the useState hook to maintain an array of generated numbers. When the "Generate Random Number" button is clicked, it generates a random number and checks if it already exists in the state. If it does, it recursively generates a new number. If the number is unique, it adds it to the state and displays the list of generated numbers in reverse order.
Output of React Js Generate Random Number Between 1 - 1000 without repetition
How can I use Reactjs to generate a random number between 1 and 10?
This Reactjs code generates a random number between 1 and 10 when a button is clicked. It uses the useState hook to manage state and stores the random number in the 'randomNumber' variable. The 'generateRandomNumber' function calculates the random number using Math.random() and updates the state with the generated number. The random number is displayed on the screen when it is generated.
Output of React Js Generate Random Number Between 1 - 10
How can I use Reactjs to generate a random number between 1 and 6?
This Reactjs code generates a random number between 1 and 6 when the "Generate Random Number" button is clicked. It uses the useState hook to maintain the state of the random number. The generateRandomNumber function calculates the random number using Math.random() and updates the state with setRandomNumber. The result is displayed on the web page under "Random Number" when generated.