React Js Download Text on Click
.jpg)
React Js Download Text on Click: To download text on click in ReactJS, you can create a download button with an onClick event handler that calls a function to generate a download link. Within the function, you can create a new Blob object with the text content and set its type to 'text/plain'. Then, you can create a URL object using window.URL.createObjectURL() and set the Blob as its parameter. Finally, you can create a link element with the href attribute set to the URL object and the download attribute set to the desired file name, and trigger a click event on it using a ref. This will download the text file when the button is clicked.
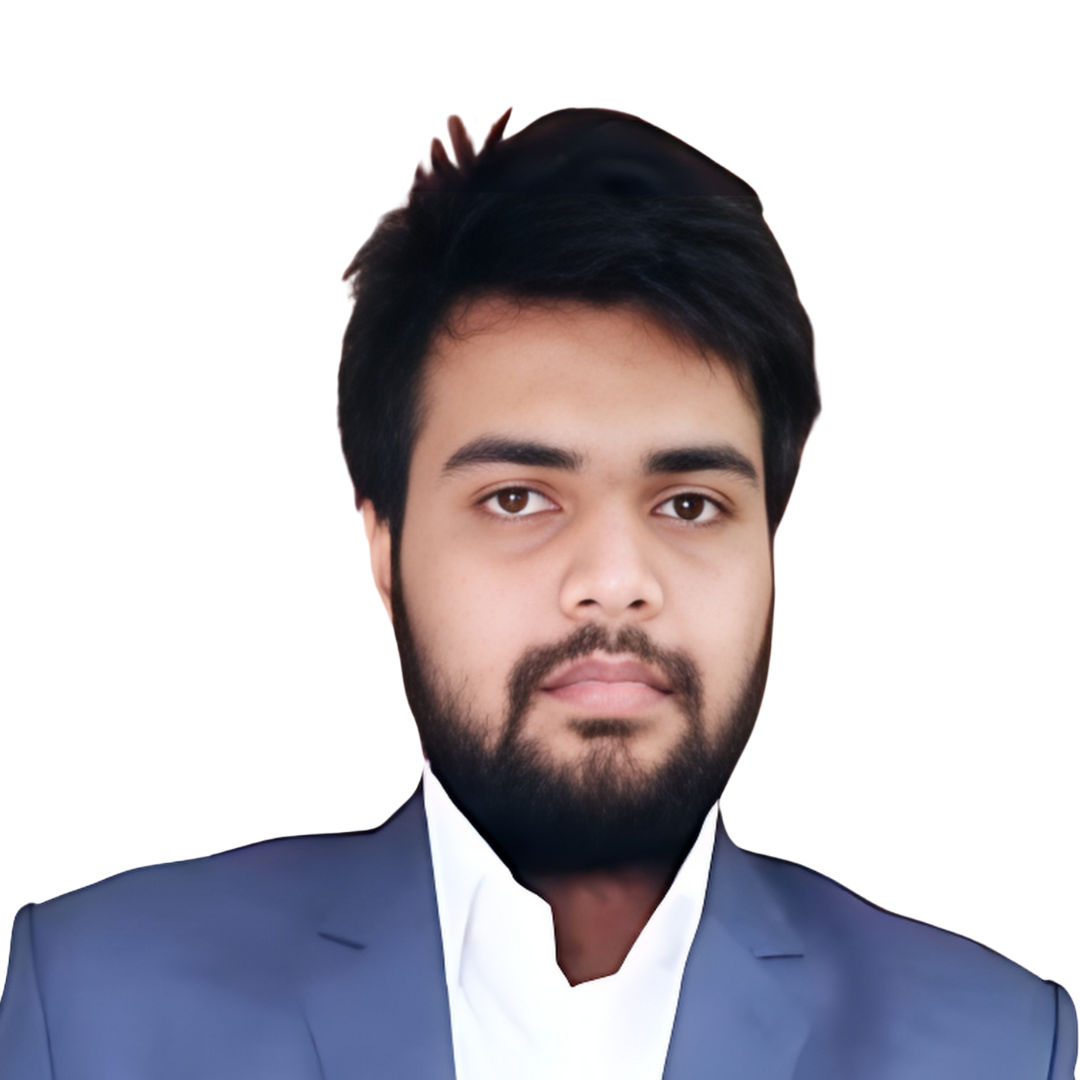
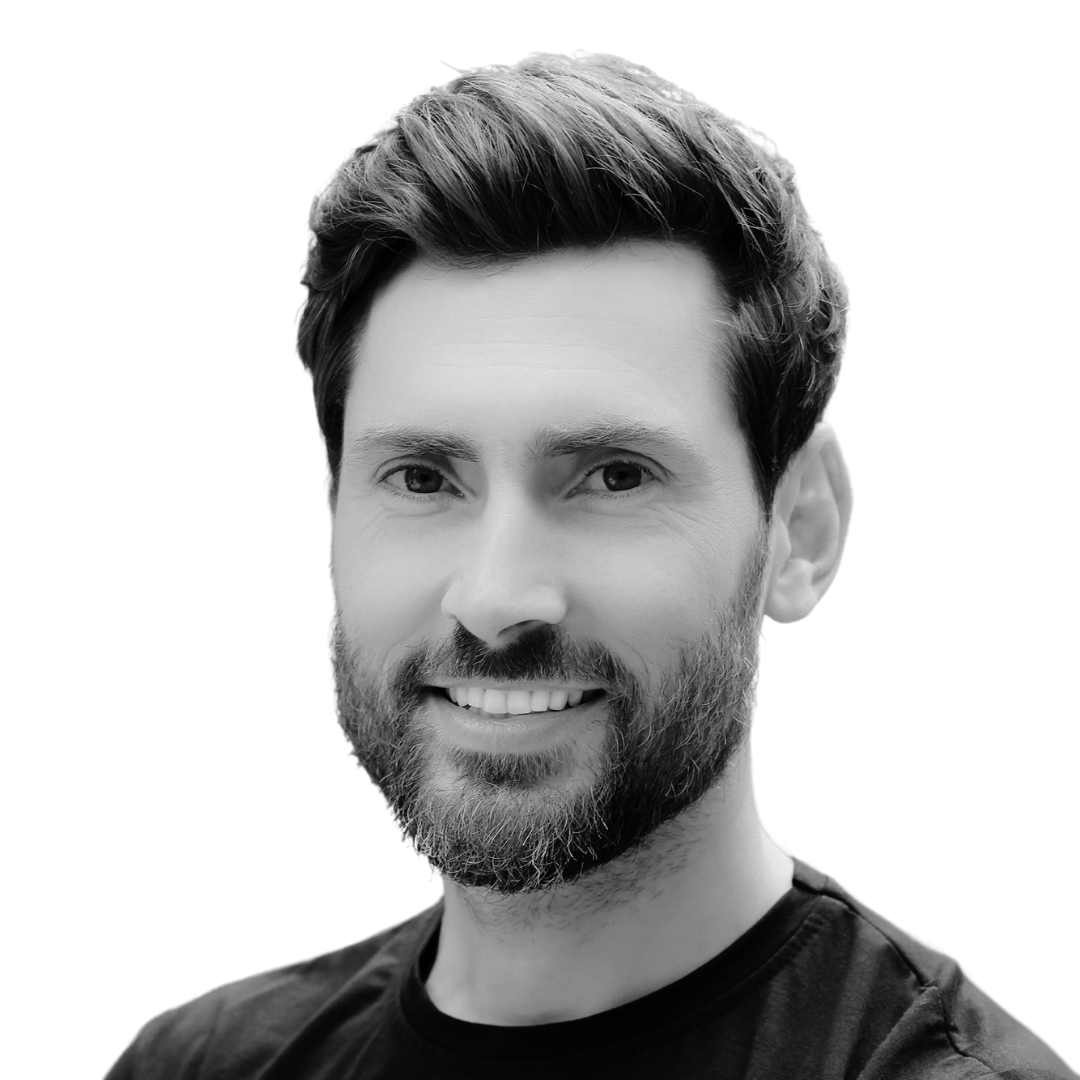
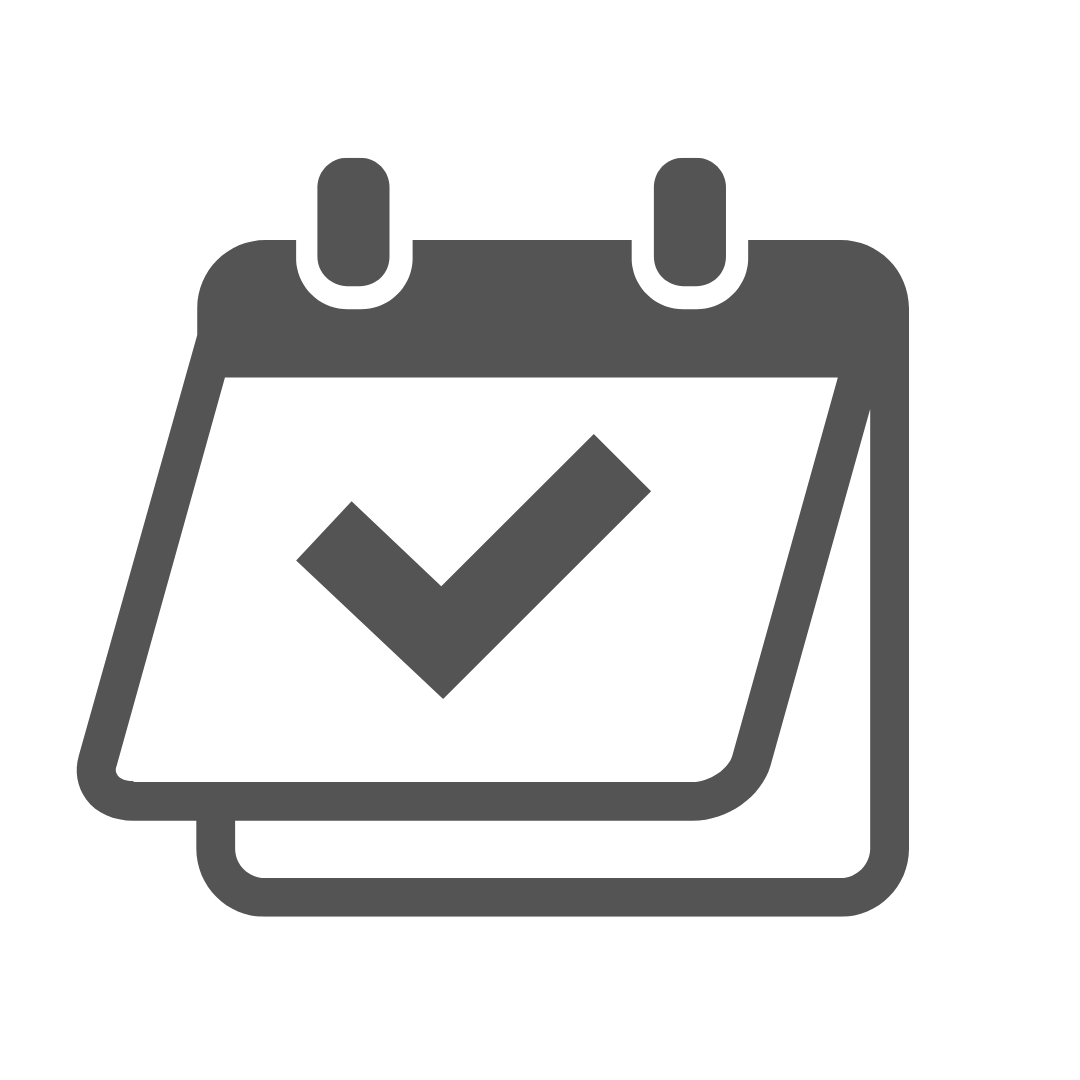
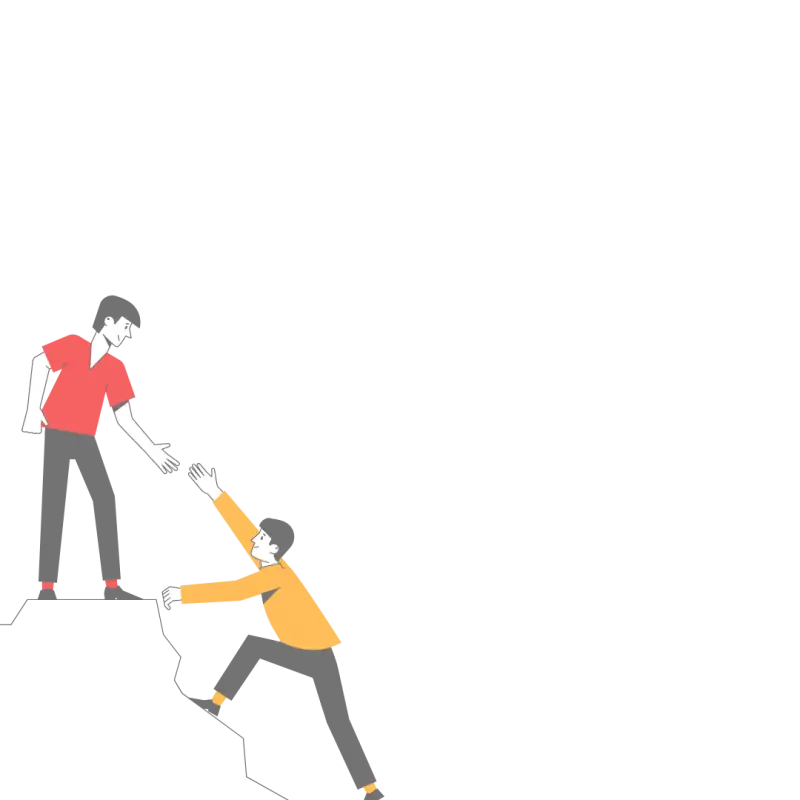
Thanks for your feedback!
Your contributions will help us to improve service.
How can I enable text file download on button click in ReactJS?
This code is a React component that displays a textarea element and a button. The textarea element contains some default text, but the user can edit it. When the button is clicked, the content of the textarea is downloaded as a text file.
The code uses the React useState hook to manage the state of the textarea content. When the button is clicked, a function called downloadText is called.
This function creates a new Blob object with the text content, creates a temporary <a> element with a download attribute, sets the href attribute to the URL of the Blob object, triggers a click event on the <a> element to download the file, and cleans up the URL by revoking the object URL after some time..
Output of React Js Download Text on Click