React Js Show or Hide Navbar on Scroll
React Js Show or Hide Navbar on Scroll:To show or hide a navbar on scroll in React.js, you can utilize event listeners and state management. Create a state variable to track the scroll position. Attach a scroll event listener to the window, updating the state as the user scrolls. Conditionally render the navbar based on the scroll position, using CSS transitions or animation to make it appear or disappear smoothly. Implementing this logic ensures that the navbar is visible when users scroll up and hidden when they scroll down, enhancing the user experience by optimizing screen real estate.
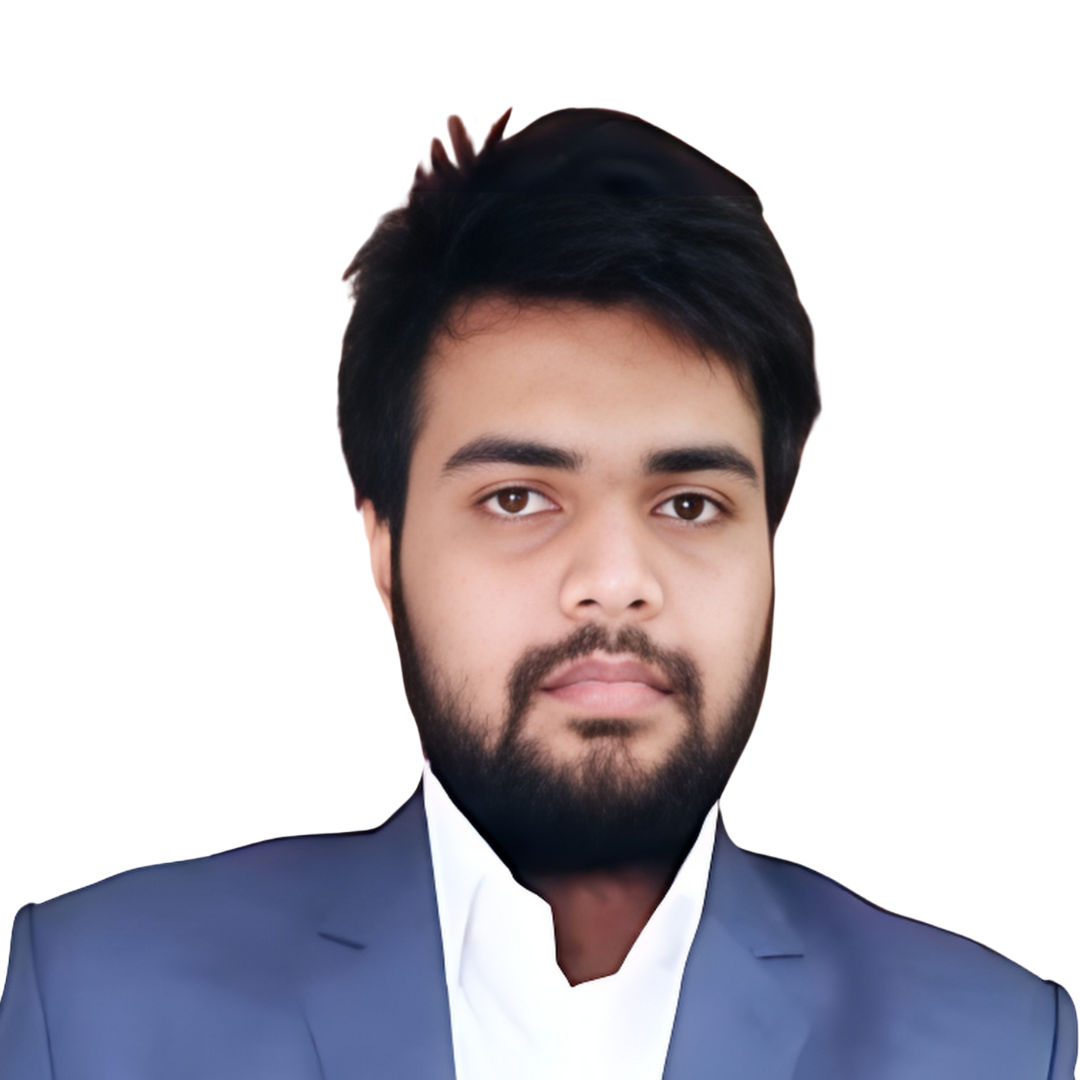
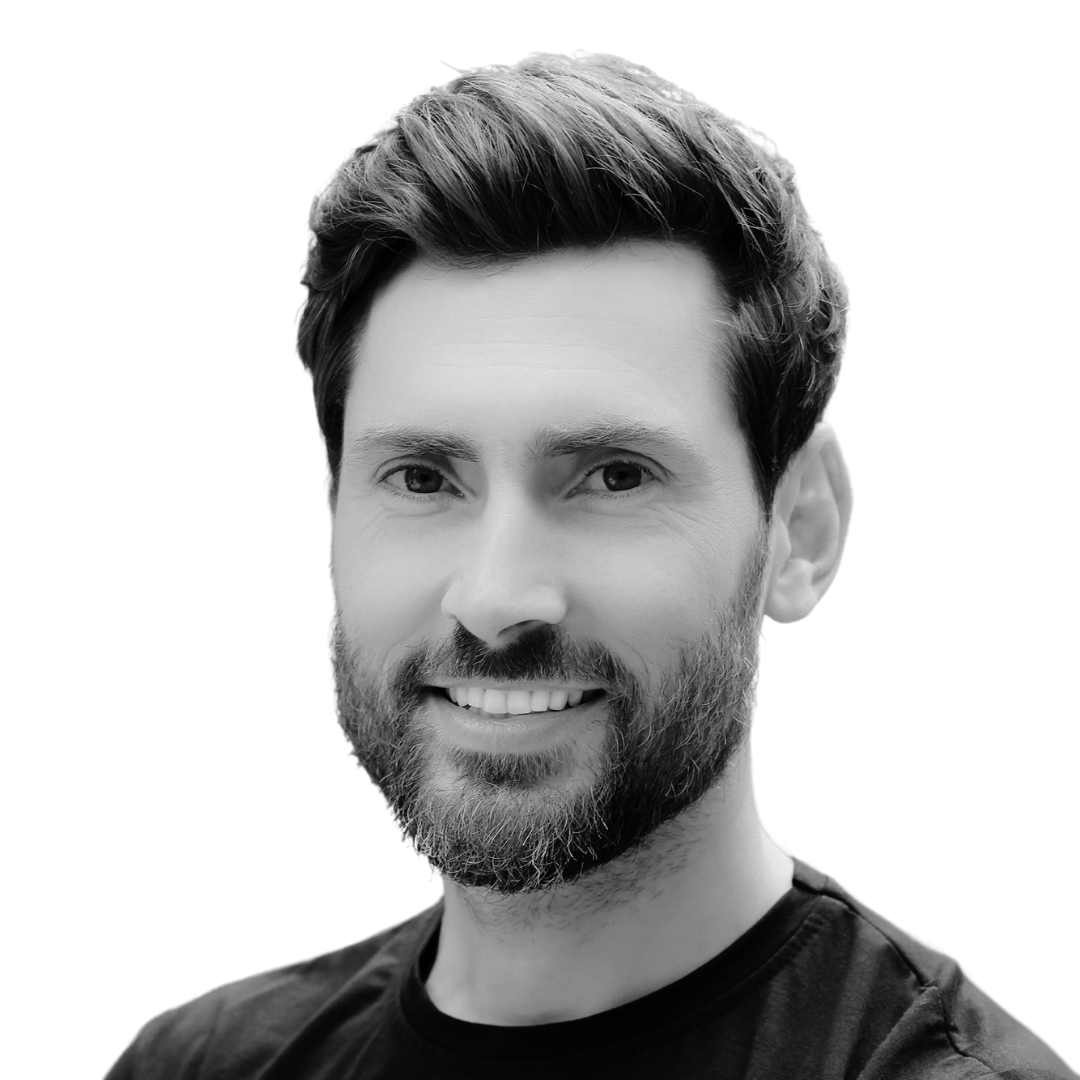
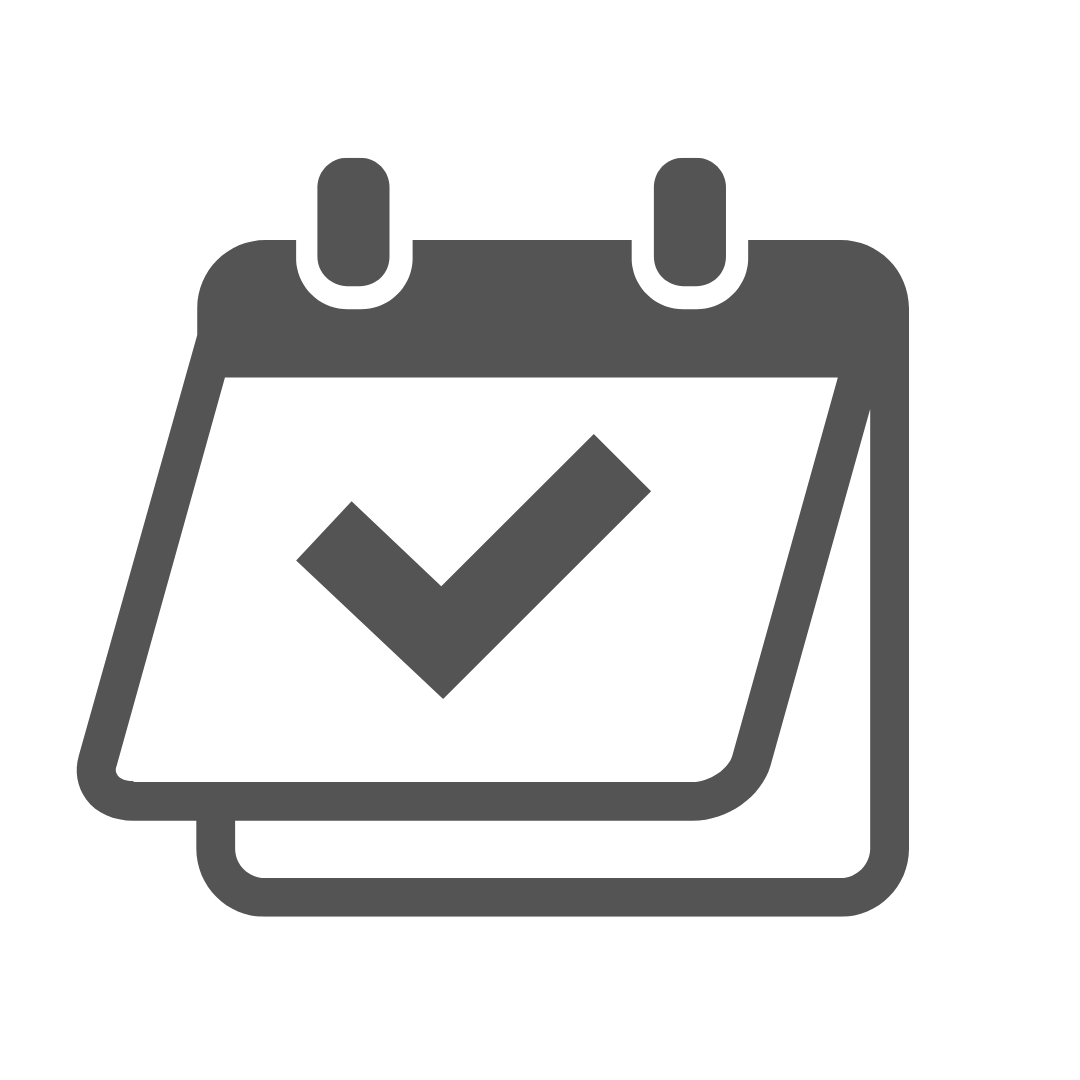
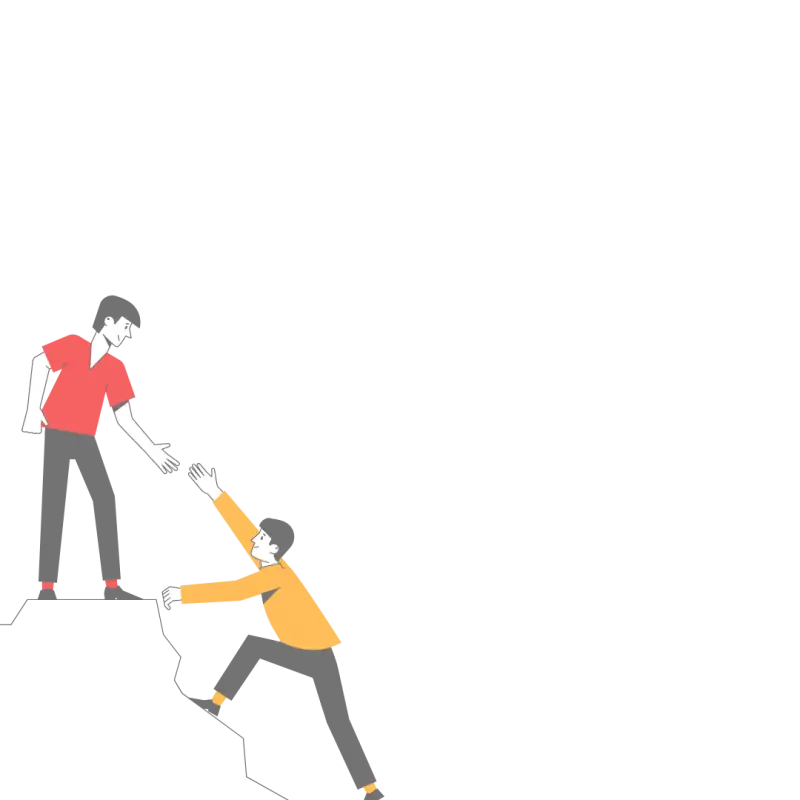
Thanks for your feedback!
Your contributions will help us to improve service.
How can I implement a feature in Reactjs to show or hide a navbar based on the user's scroll position on the page?
This React.js code creates a webpage with a dynamically appearing/disappearing navbar based on the user's scroll position. It uses the useState
and useEffect
hooks to manage the navbar's visibility. When the user scrolls more than 100 pixels down, the handleScroll
function sets isNavbarVisible
to true
, making the navbar visible; otherwise, it hides it. The useEffect
hook adds and removes a scroll event listener to manage this behavior. The navbar's visibility is controlled by conditional CSS classes. This interactive design enhances user experience by minimizing screen clutter and revealing the navbar only when needed.
Output of React Js Show or Hide Navbar on Scroll
Befor Scrolling
After Scrolling