React Js Remove Vowels from a String

React Js Remove Vowels from a String :Removing vowels from a string is a common task in text manipulation. With ReactJS, you can create a simple web application to demonstrate this functionality. In this example, we'll create a React component that takes an input string, removes its vowels, and displays the result. The code you provided accomplishes this task.
The code snippet you shared is a ReactJS application that removes vowels from a given string and displays the result
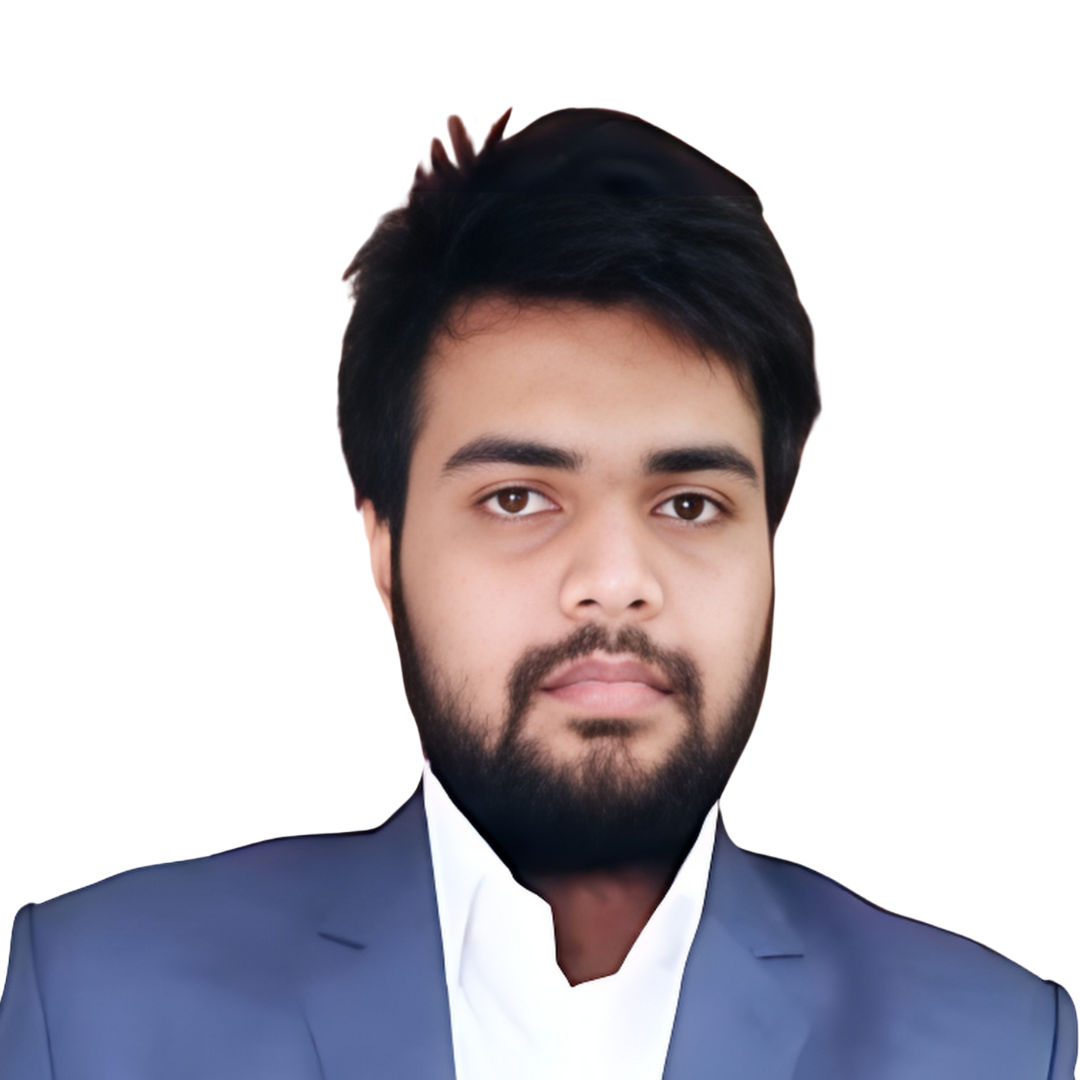
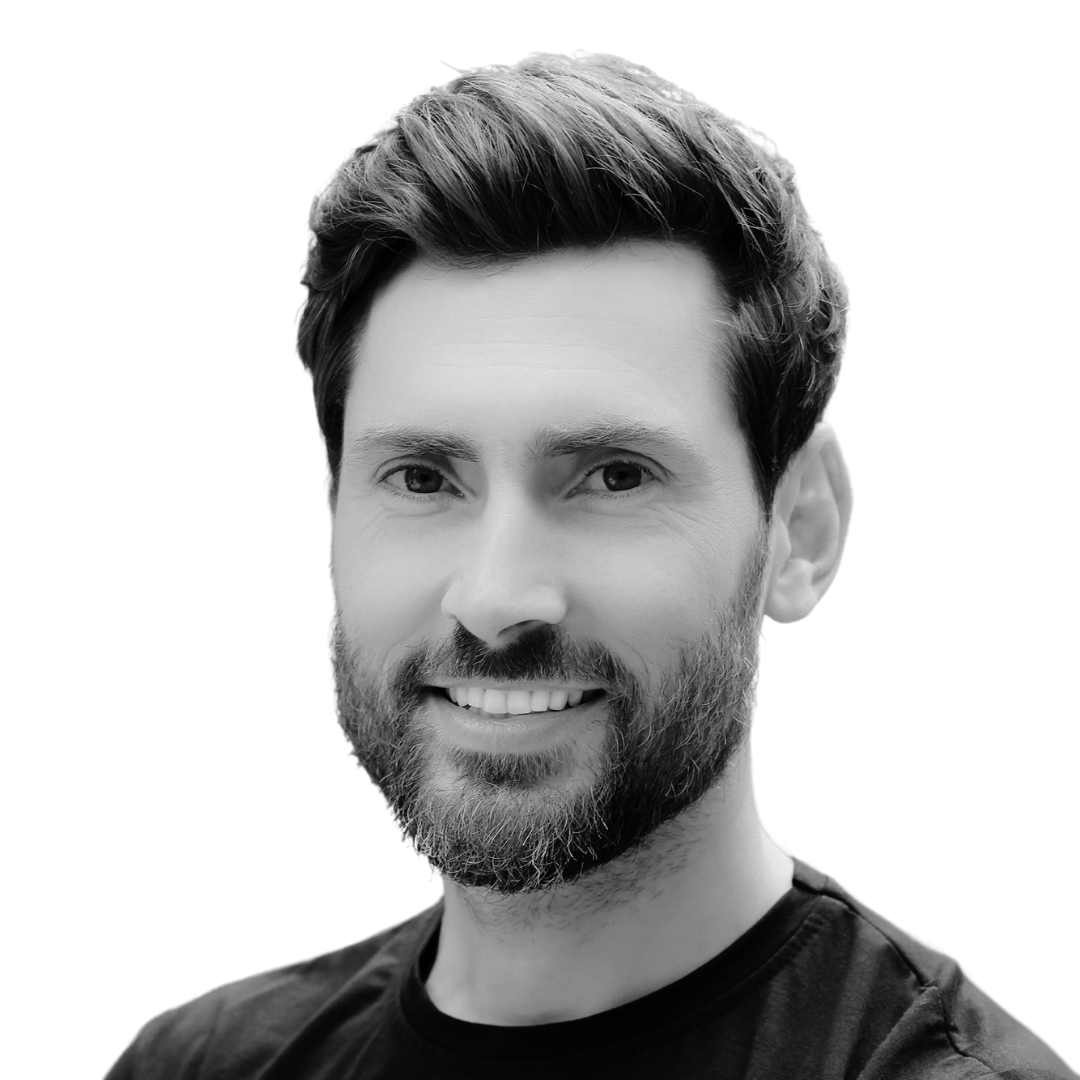
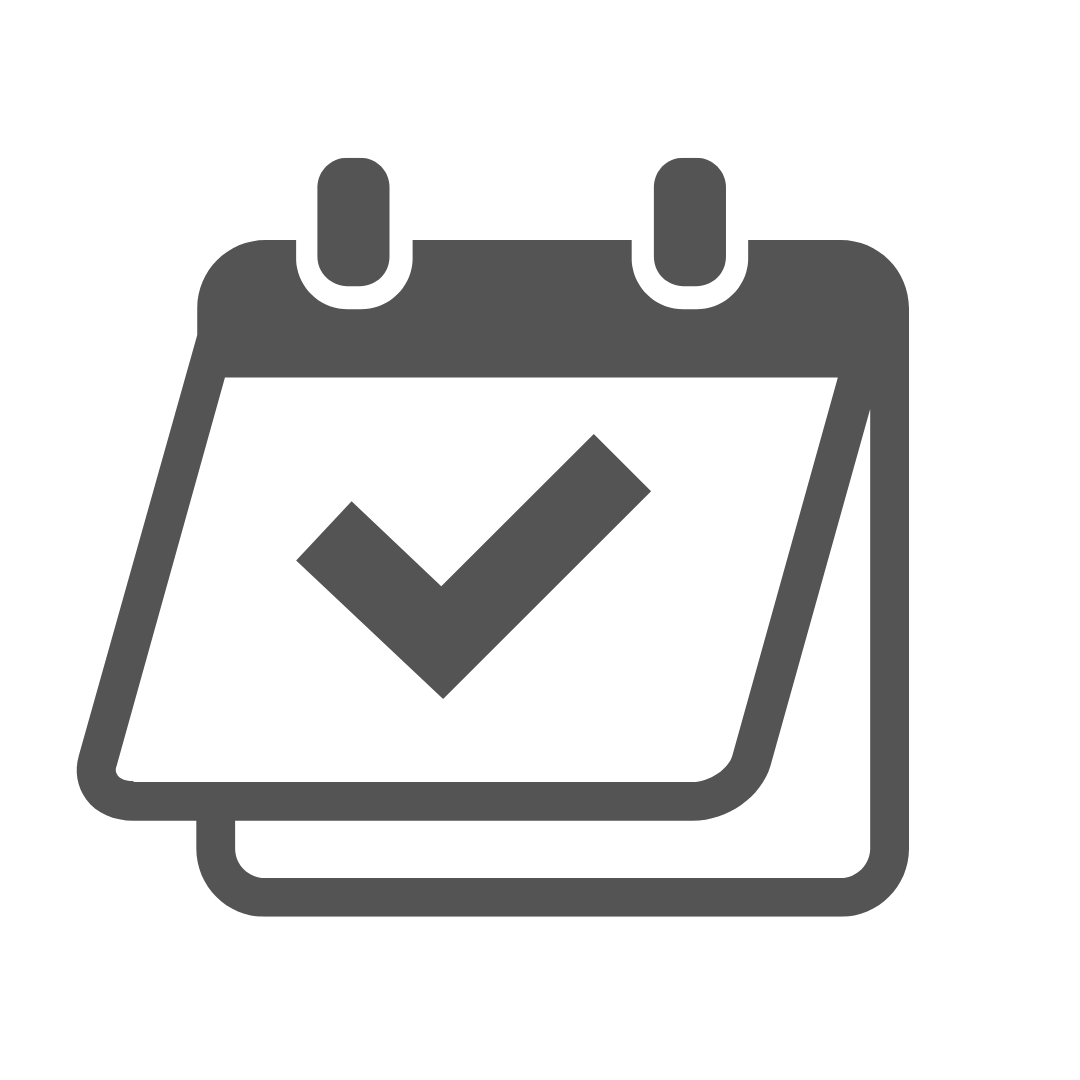
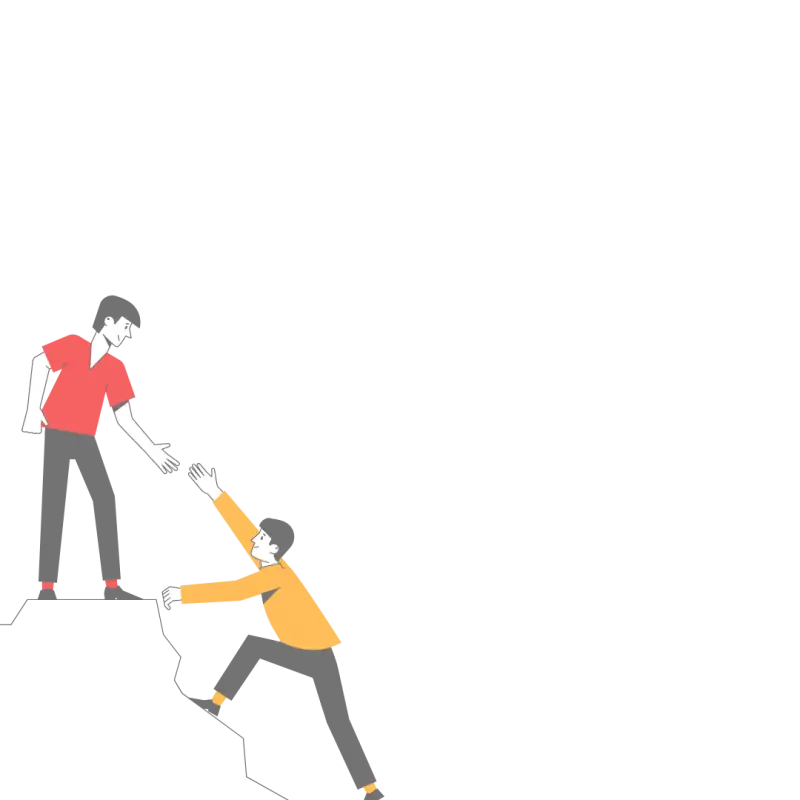
Thanks for your feedback!
Your contributions will help us to improve service.
Understanding the Implementation: Step-by-Step Code
React Component Setup
We start by setting up a React functional component named App
. Within this component, we use the useState
hook from React to manage two pieces of state: inputString
and resultString
. The inputString
state will hold the input string provided by the user, and the resultString
state will store the string with vowels removed.
React Js Remove Vowels From A String
We initialize inputString
with a default value for demonstration purposes, but you can set it to an empty string or any other value as needed.
React Remove Vowels Function
We define a function named removeVowels
within the component. This function uses the JavaScript replace
method with a regular expression to find and replace all vowels (both lowercase and uppercase) in the inputString
with an empty string, effectively removing them.
Rendering the User Interface
The render
method of our component returns JSX, which defines the user interface. We have a simple UI that consists of an input field, a button, and a display area for the result
The input field is controlled by React state, with its value linked to the inputString
state variable. When the user types in the input field, the onChange
handler updates the inputString
state.
Clicking the "Remove Vowels" button calls the removeVowels
function, which modifies the resultString
state, and the result is displayed in the paragraph with the class 'result.'
The output of this code will be :
For example, if you enter "Font Awesome Icons" into the input field and click the "Remove Vowels" button, the "Result String" section will display:
Result String: Fnt wsm Icns
summary
The provided React.js code snippet demonstrates a straightforward way to create a web application that removes vowels from a given string, providing a clear example of how React hooks can be used to manage state and update the UI dynamically.