React Js Generate Random Password

React Js Password Generator:A React js password generator is a tool that generates passwords with various combinations. It can include alphanumeric characters, lowercase and uppercase letters, custom characters, and numbers. Users can customize the password generation by selecting their preferred combination options. This generator utilizes the React.js framework to create an interactive user interface, allowing users to easily specify their password requirements. By incorporating different character types, the generator enhances password strength and security by producing a wide range of possible combinations for users to choose from
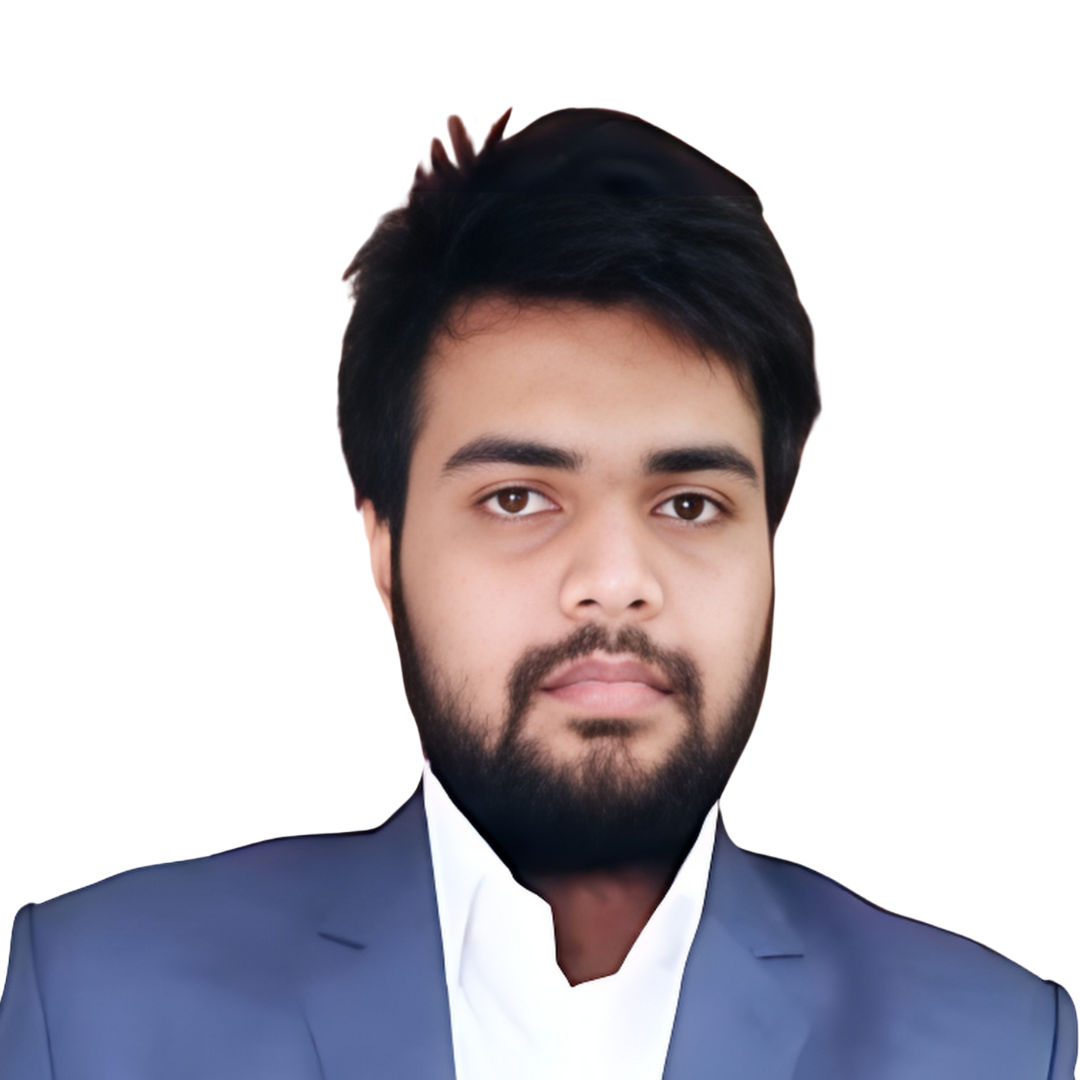
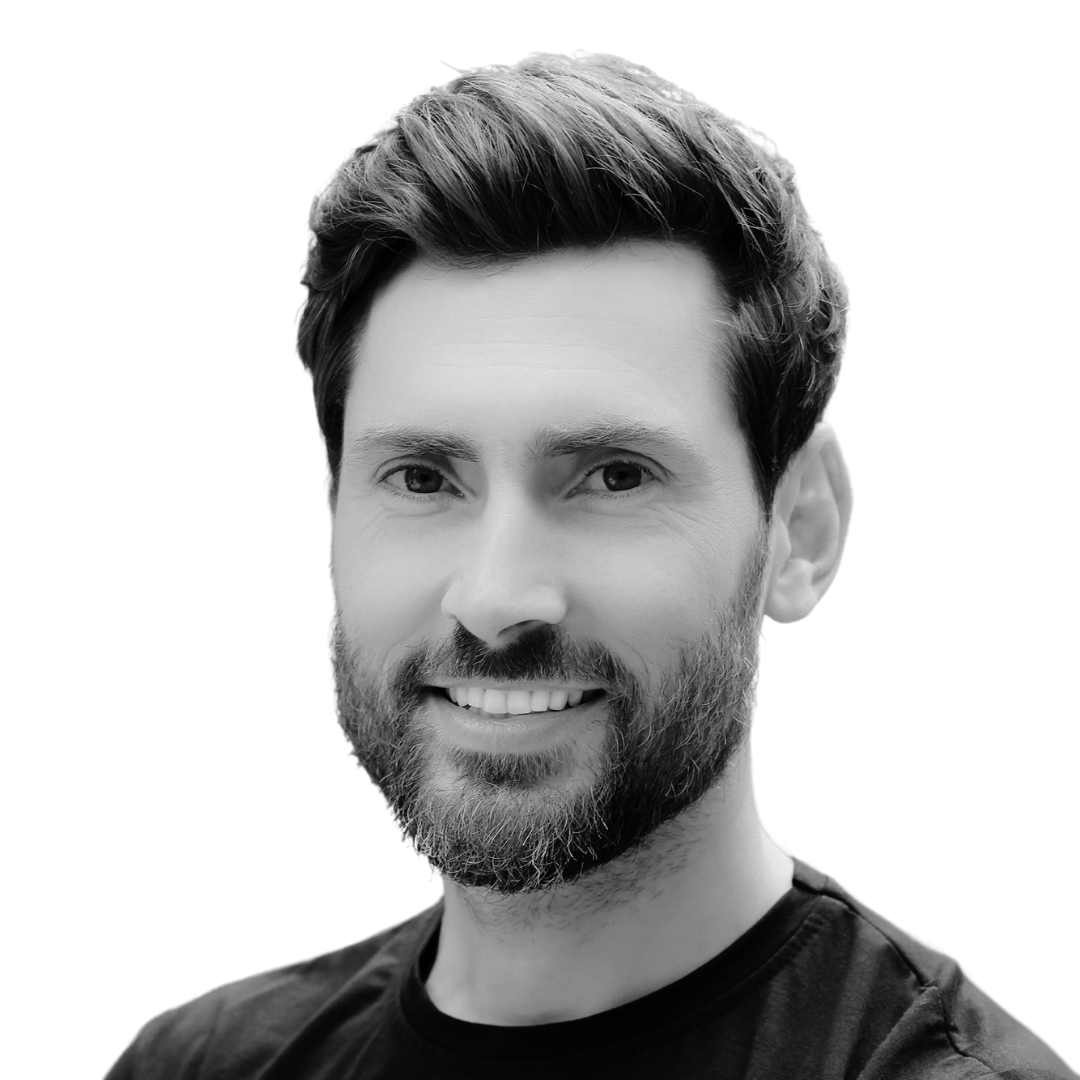
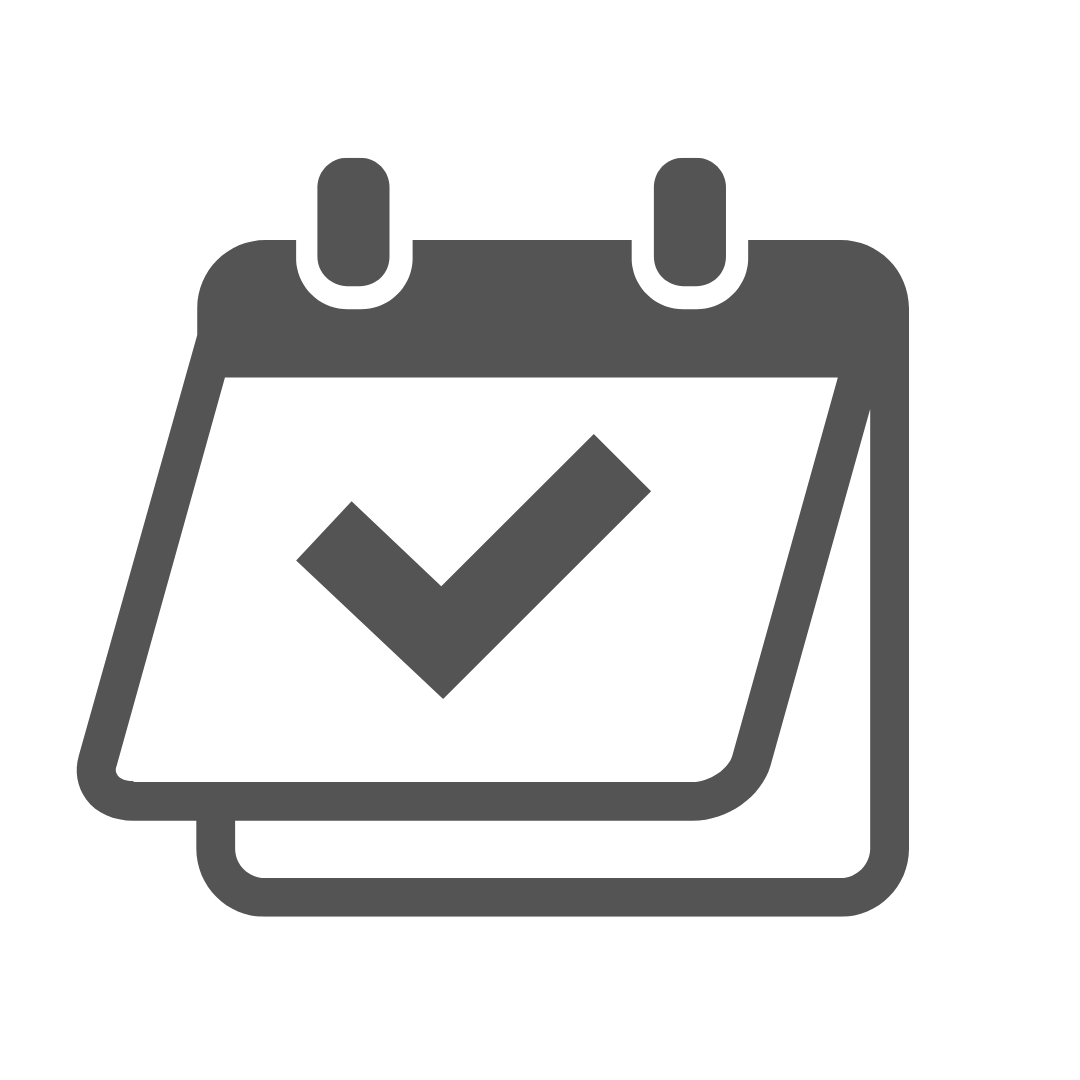
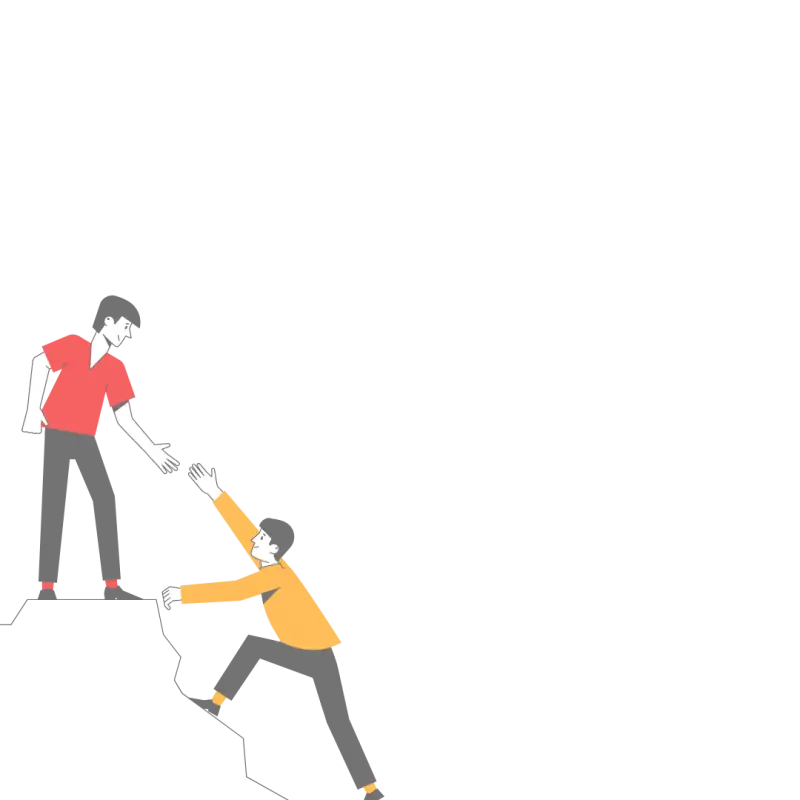
Thanks for your feedback!
Your contributions will help us to improve service.
How can I Generate a Random Password using React js?
This React.js code creates a password generator. It allows users to select a difficulty level (easy, medium, or hard) and set a password length. When the "Generate Password" button is clicked, it generates a random password based on the chosen difficulty level and length, displaying it on the page.
The code uses React's useState for managing state variables and a switch statement to determine character sets based on difficulty. It then constructs a password by randomly selecting characters from the chosen character set. Finally, it updates the password state variable and renders it in the UI.
Output of React Js Password Generator
Easy Password Generator
Medium Password Generator
Hard Password Generator
How can I create a password generator in React.js that includes both lowercase and uppercase letters
The React JS Password Generator is a tool that generates passwords using both lowercase and uppercase letters. It is built using the React JavaScript library and allows users to create strong and secure passwords.
By combining letters from both lowercase and uppercase alphabets, the generator enhances password complexity, making them harder to guess or crack
Output of React Js Password Generator
How can I create a ReactJS password generator that generates passwords using alphanumeric letters?
The React JS Password Generator is a tool that generates passwords using alphanumeric letters.
It is built using React JS, a popular JavaScript library for building user interfaces.
This generator creates passwords consisting of a combination of both letters and numbers, providing a stronger level of security.
How can I create a React.js password generator that includes uppercase letters?
A React.js password generator is a tool that generates passwords using uppercase letters. It utilizes the React.js library to create an interactive user interface.
The generator randomly selects uppercase letters and combines them to form a password. Users can adjust the length of the password and customize other options as well.
What is a React.js implementation for generating passwords consisting only of lowercase letters?
A React.js password generator is a tool that generates passwords using only lowercase letters.
It utilizes the React.js framework to create a user interface where users can specify the length of the password they want.
The generator then randomly selects lowercase letters and combines them to form a password of the desired length
Output of React Js Password Generator
How can I create a password generator in React.js that includes numbers?
It utilizes the React.js framework to create an interactive user interface. By specifying the desired length of the password, the generator randomly selects numbers and combines them to form a unique password.
This approach provides a simple and efficient way to create secure passwords with numeric characters.