React js onclick pass parameter
React js onclick pass parameter:In ReactJS, passing parameters to functions during onClick events can be achieved through various techniques. Arrow functions in JSX can directly pass parameters by enclosing the function call within an arrow function, like onClick={() => handleFunction(parameter)}
. Alternatively, the Function Bind syntax (onClick={handleFunction.bind(this, parameter)}
) can be used to pre-set parameters. Currying involves creating a function that returns another function, allowing parameter passing like onClick={curriedFunction(parameter)}
. Another approach is using data-*
attributes where data can be attached to elements and accessed via event.target.dataset
within the onClick handler. While these methods work, care should be taken with performance implications, especially when re-rendering components. Choose the technique that best fits your use case while considering readability, maintainability, and performance.
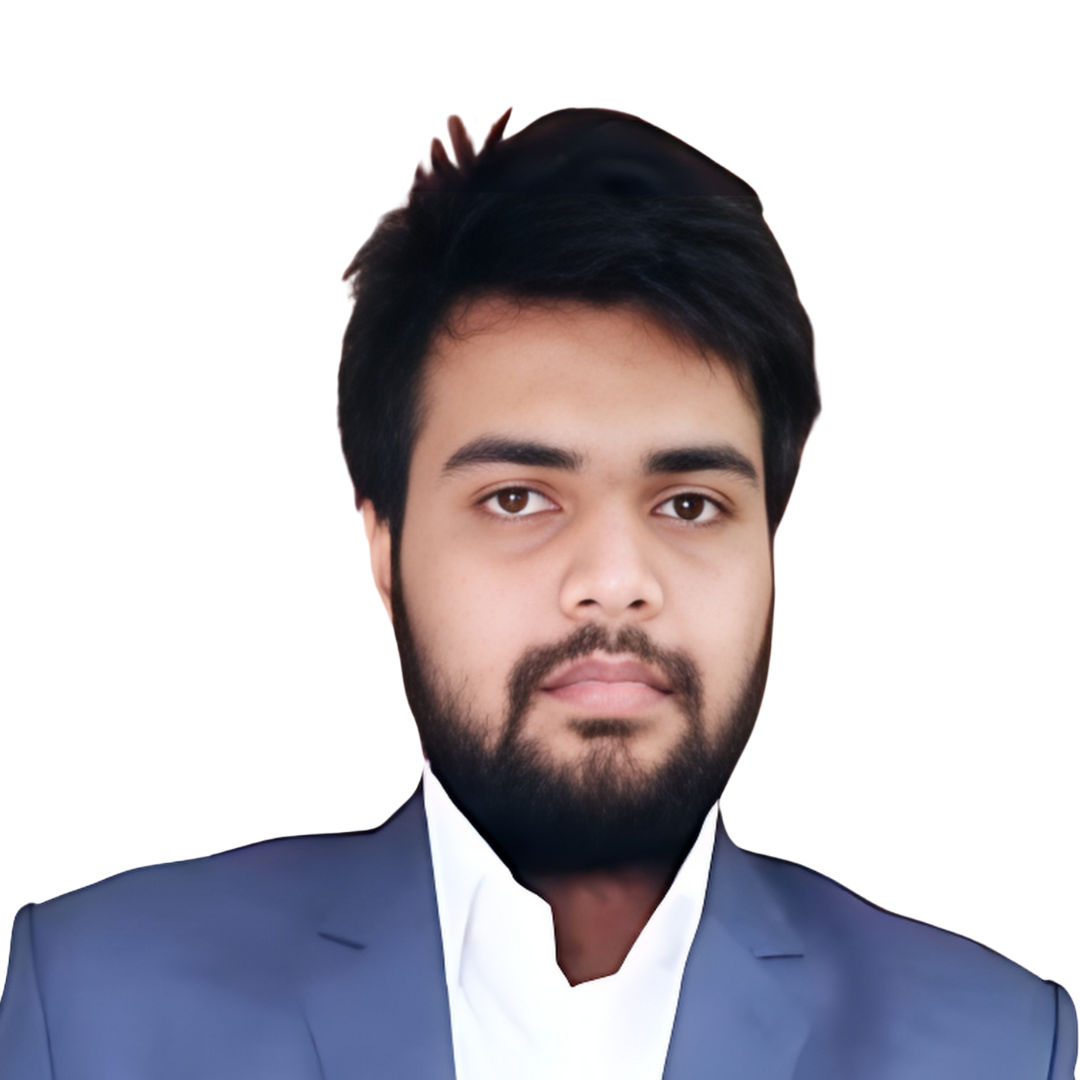
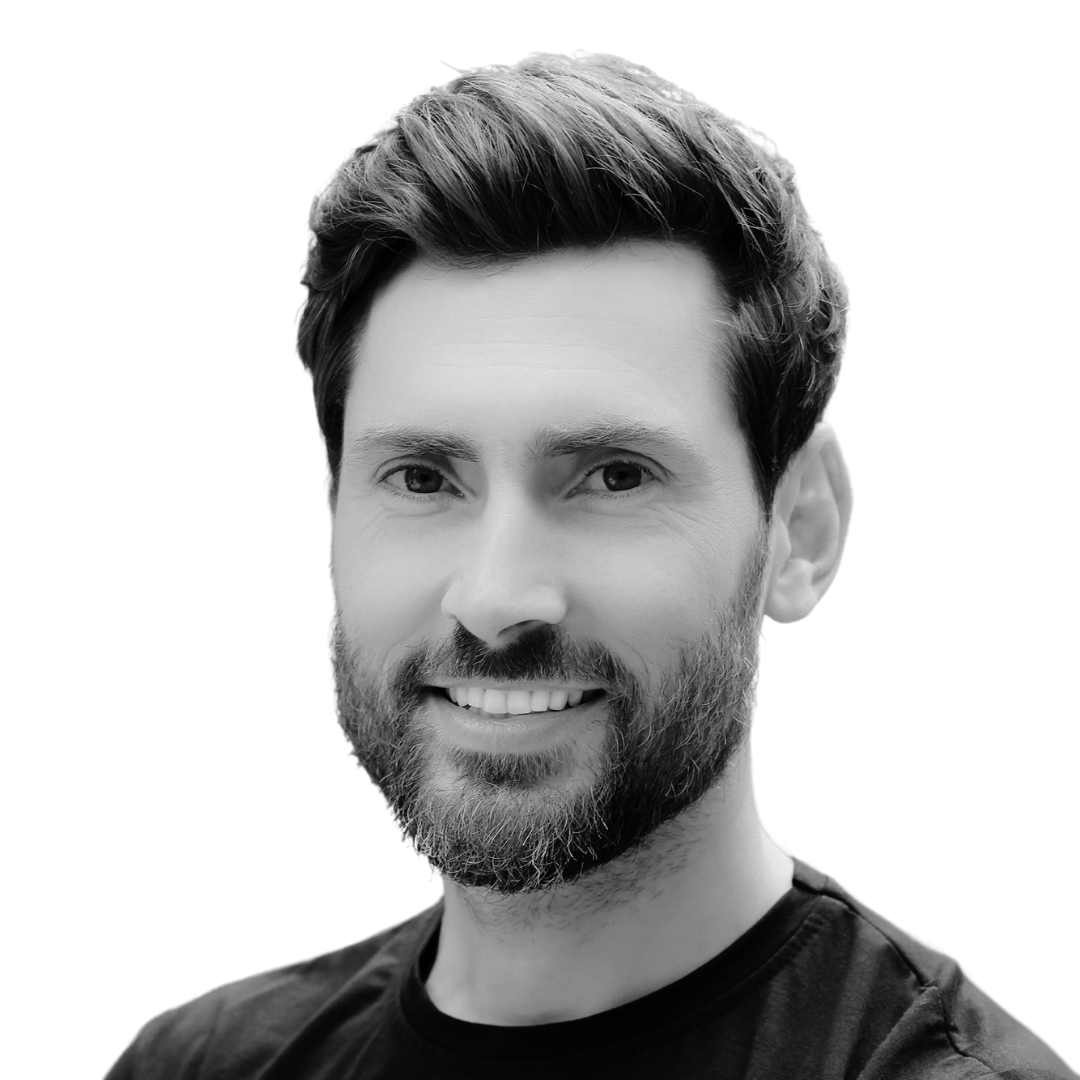
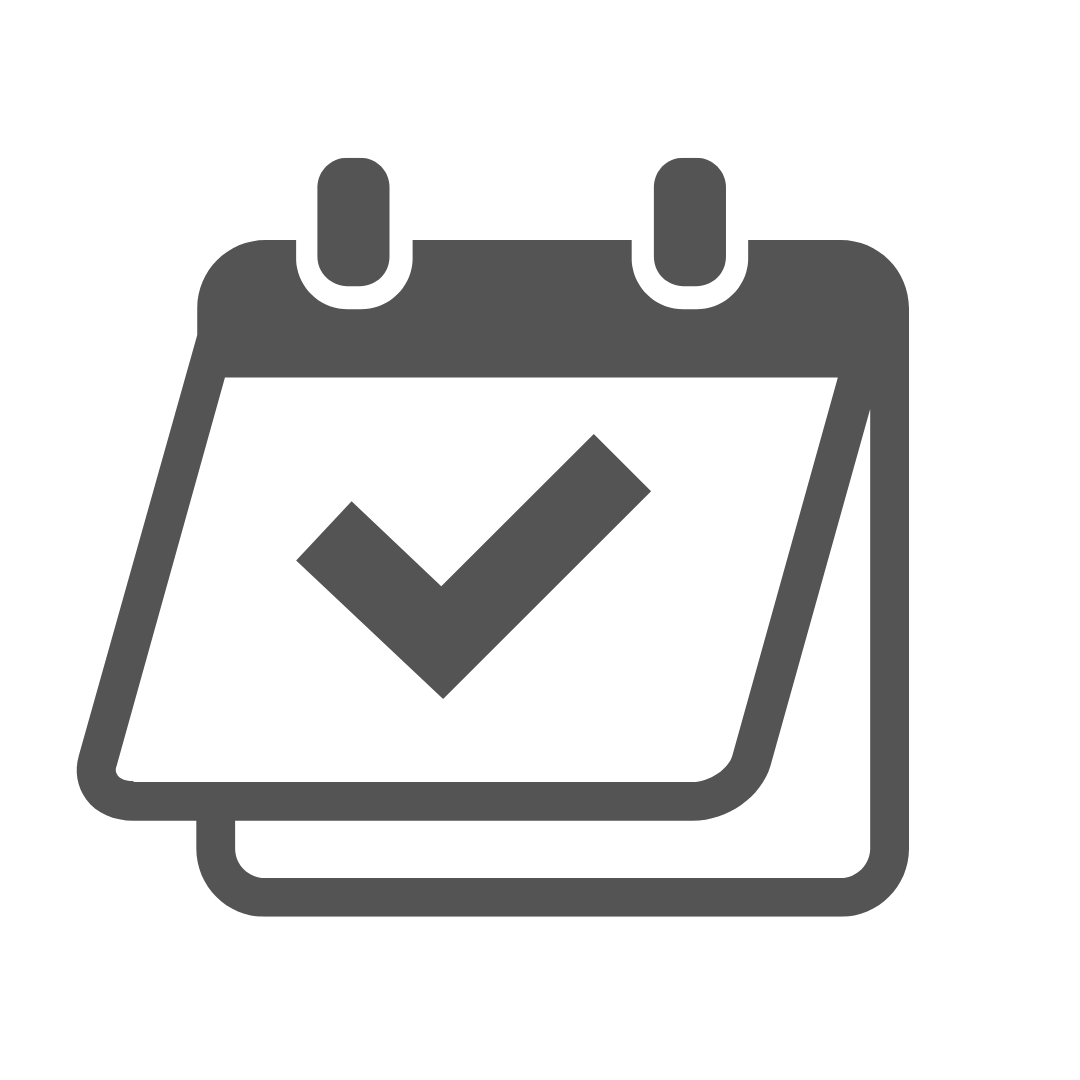
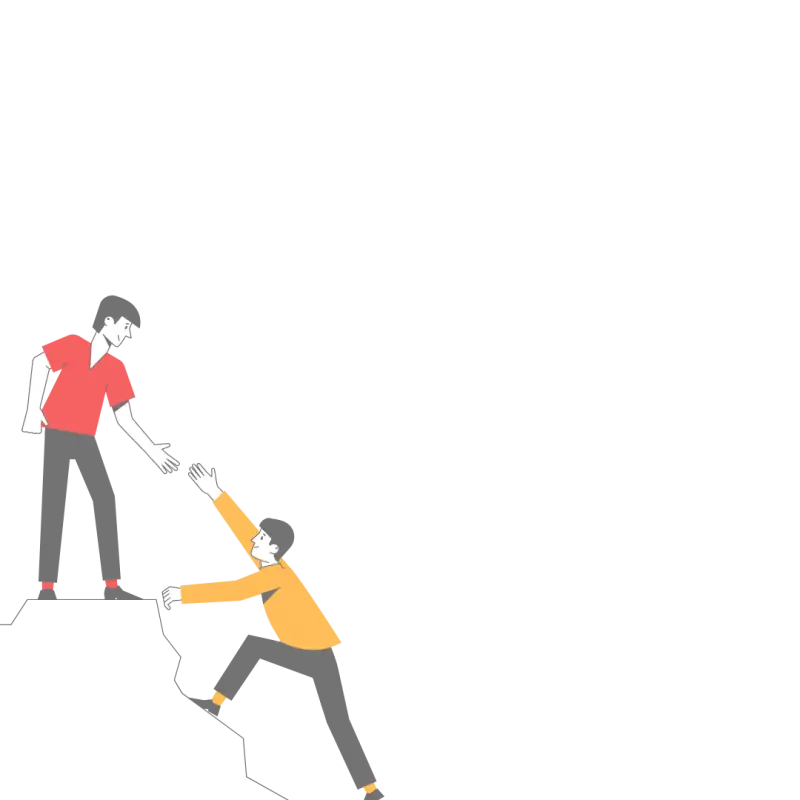
Thanks for your feedback!
Your contributions will help us to improve service.
How can I pass parameters using an arrow function in JSX's onClick
event handler in ReactJS?
In this React.js example, an App
component defines a handleClick
function that logs a parameter. Inside the component's JSX, a button triggers the function with a parameter using an arrow function. When clicked, the button invokes handleClick
with the parameter 'Hello'. The rendered output displays a heading and the button. Upon clicking the button, the console logs "Clicked with parameter: Hello". This demonstrates passing parameters via an arrow function in React.js's JSX syntax.
How do you pass parameters using the Function Bind syntax in React.js's onClick
event handler?
In this React.js code snippet, an App
component is defined. It includes a handleClick
function that logs a parameter when invoked. The return
section renders a container with a heading and a button. By using the bind
syntax, the handleClick
function is connected to the button's onClick
event, and the parameter 'Hello'
is passed when the button is clicked. This technique allows passing parameters to event handlers in React components without immediately invoking the function. The rendered output displays the button labeled "Click me." When clicked, the console logs "Clicked with parameter: Hello."
How can you use currying in React.js to pass parameters when defining an onClick
event handler?
The code snippet demonstrates a React.js app where clicking a button triggers a curried function. The handleClick
function accepts a parameter and returns another function. When the button is clicked, the curried function logs the provided parameter. The app renders a button labeled "Click me," and upon clicking it, the message "Clicked with parameter: Font Awesome Icons" appears. This technique of currying enables passing parameters to event handlers in React.js while maintaining separation between event triggering and parameter handling logic.
How can you use the data-*
attributes in React.js to pass parameters when handling an onClick
event?
The provided code demonstrates a ReactJS application using the data-param
attribute to pass a parameter when a button is clicked. The handleClick
function extracts the parameter value from the clicked button's data-param
attribute using event.target.getAttribute()
. Upon clicking the button labeled "Click me," the function logs the parameter value ("Font Awesome icons") to the console. The React component, defined within the script, renders a container with a title and the button