React Js Check Input Element is focused
React Js Check Input Element is focused:In Reactjs, determining if an input element is focused can be achieved using the state and event handlers. Create a state variable, like isFocused
, initialized as false
. Then, use the onFocus
and onBlur
event handlers on the input element to toggle the state. When the input is focused, set isFocused
to true
, and on blur, set it to false
. This state reflects the focus status of the input. To summarize, track the focus state using a state variable and event handlers, allowing you to ascertain whether the input element is currently focused or not in a Reactjs application.
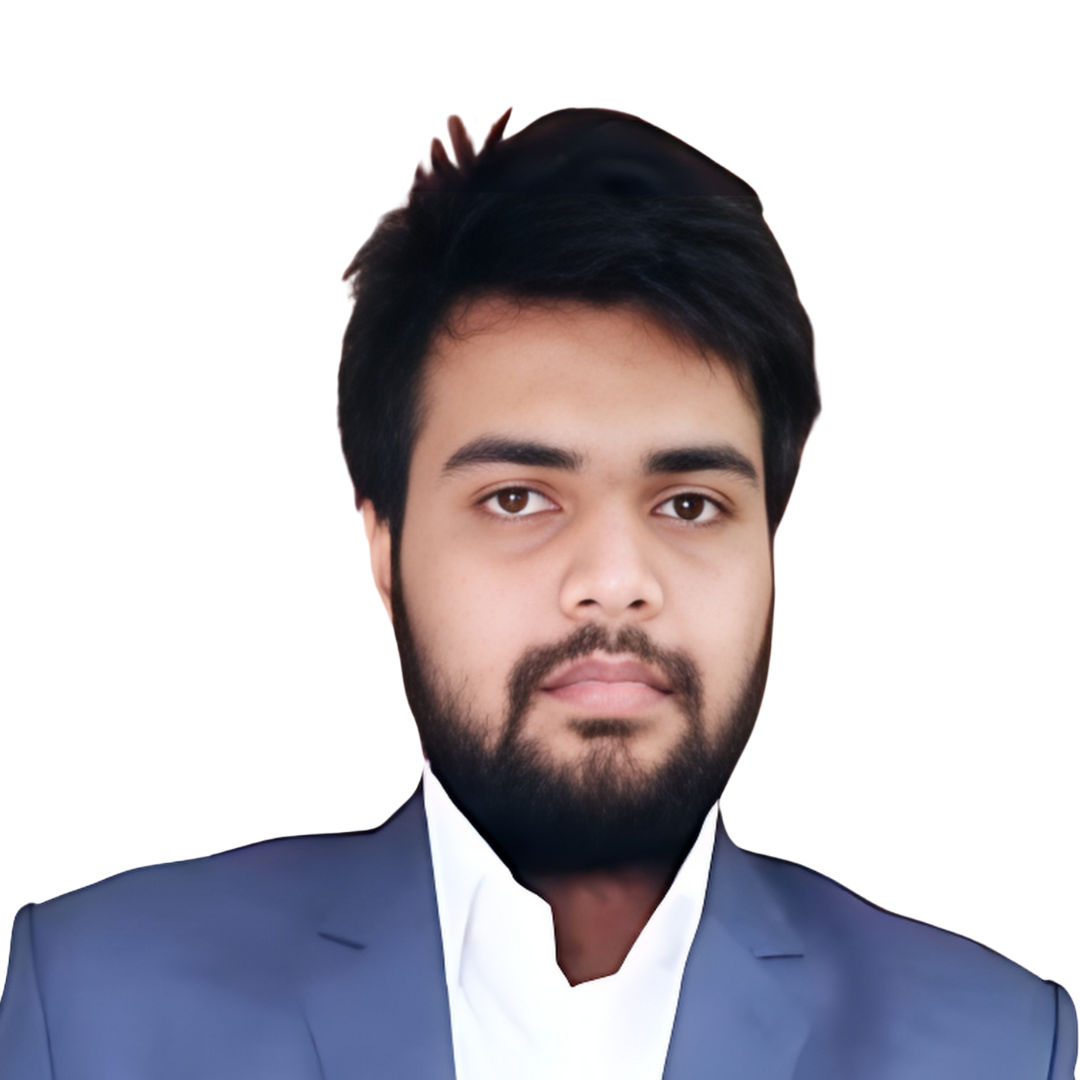
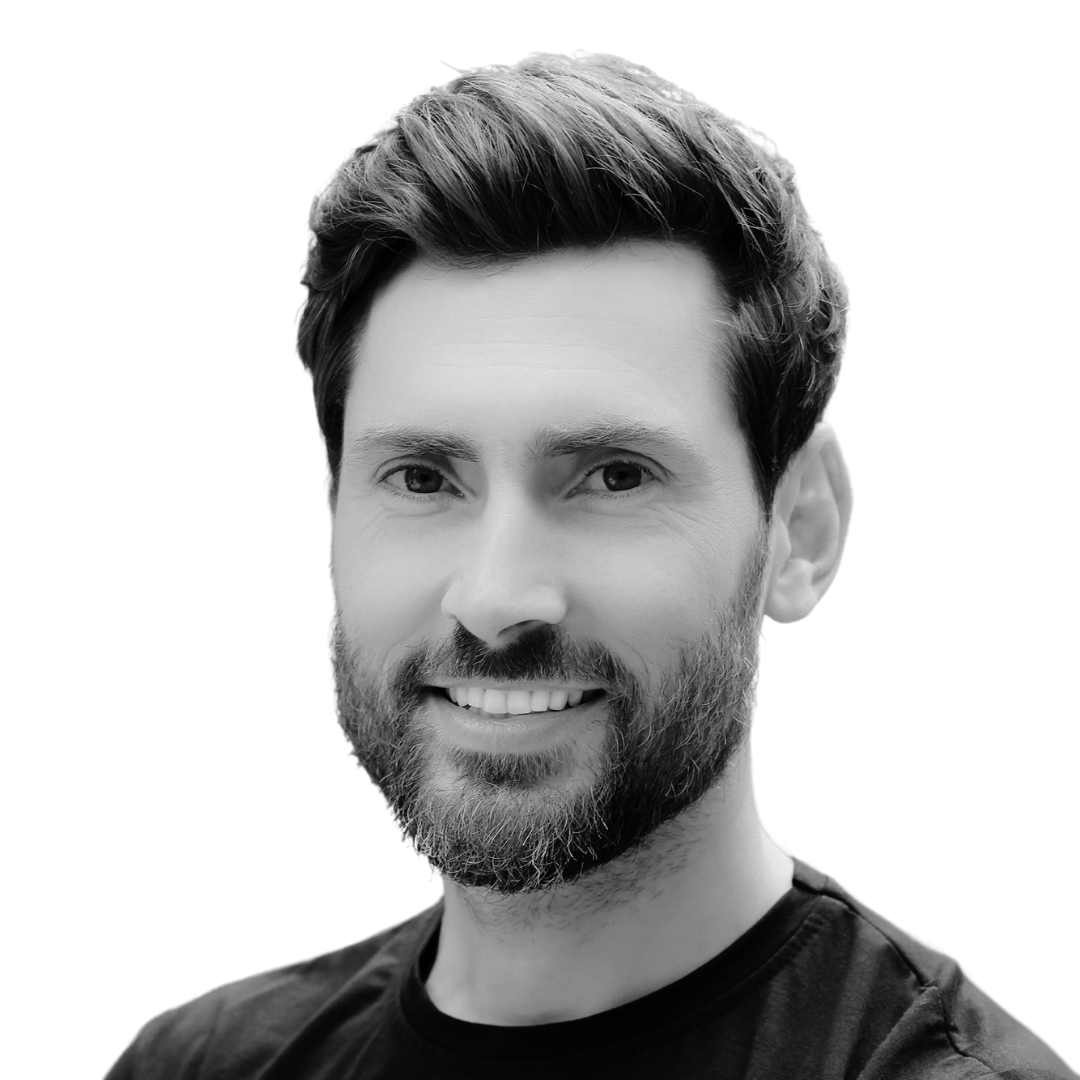
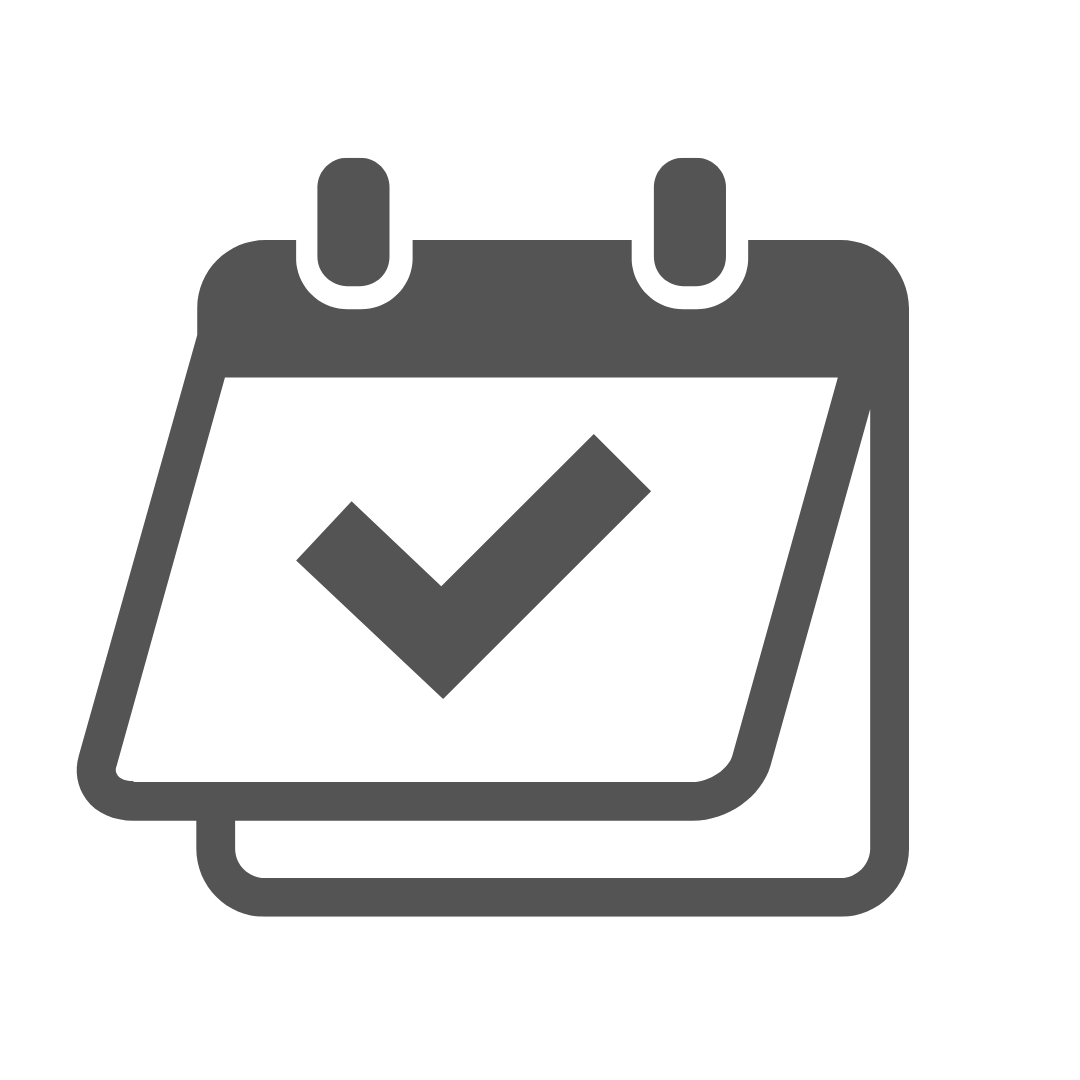
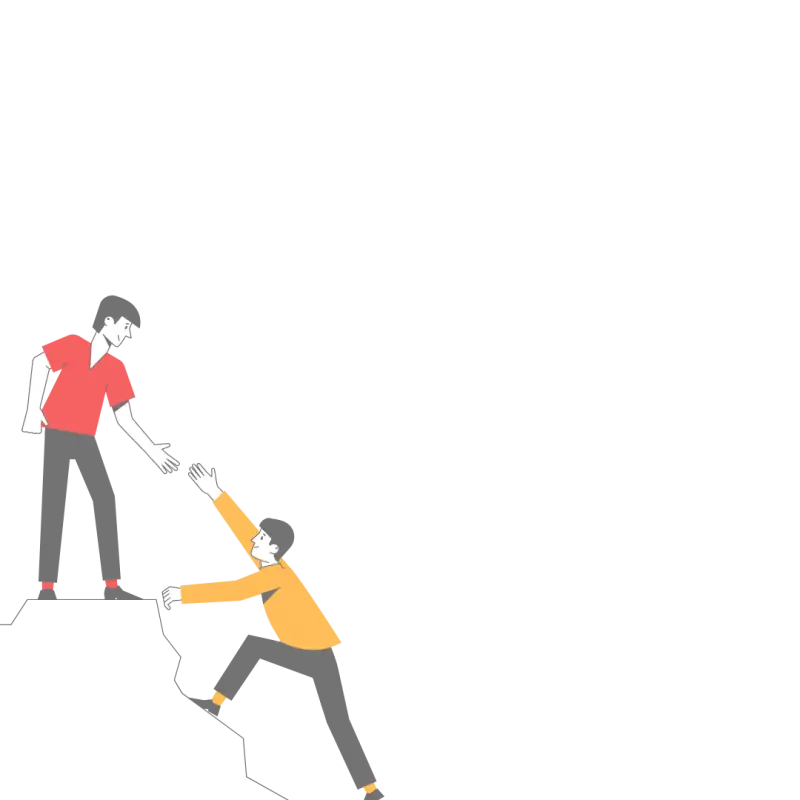
Thanks for your feedback!
Your contributions will help us to improve service.
How can you determine if an input element is currently focused in a Reactjs?
This ReactJS script uses the useState
hook to track whether an input element is focused. It responds to the onFocus
and onBlur
events, updating the isElementFocused
state accordingly. The user interface reflects whether the input is focused, displaying a message accordingly. The provided JSX renders an input field, and when focused or blurred, it updates the state and displays the focus status. The script showcases React's ability to manage and react to user interactions in the UI