React Js Conditional Rendering
React Js Conditional Rendering:React.js offers several ways to implement conditional rendering. The ternary operator can be used for simple conditions, where a condition is checked, and different components or elements are rendered based on the result.
The logical "&&" operator allows for rendering components or elements if a condition is true. The array length method enables conditional rendering based on the length of an array, displaying different content accordingly.
Finally, the "if-else" statements can be used in JSX within a function component to conditionally render components or elements. Each method provides flexibility and allows developers to handle conditional rendering in React.js efficiently.
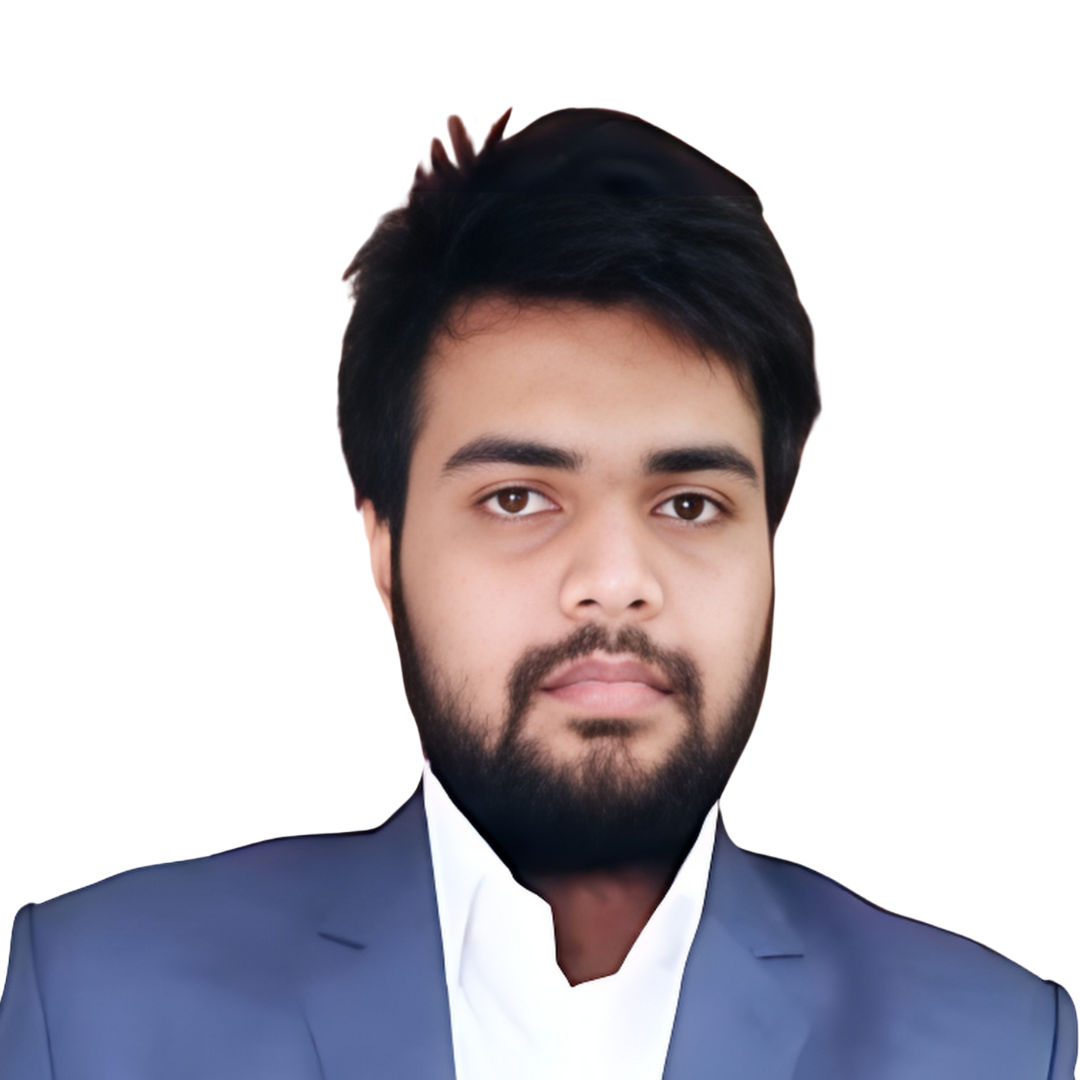
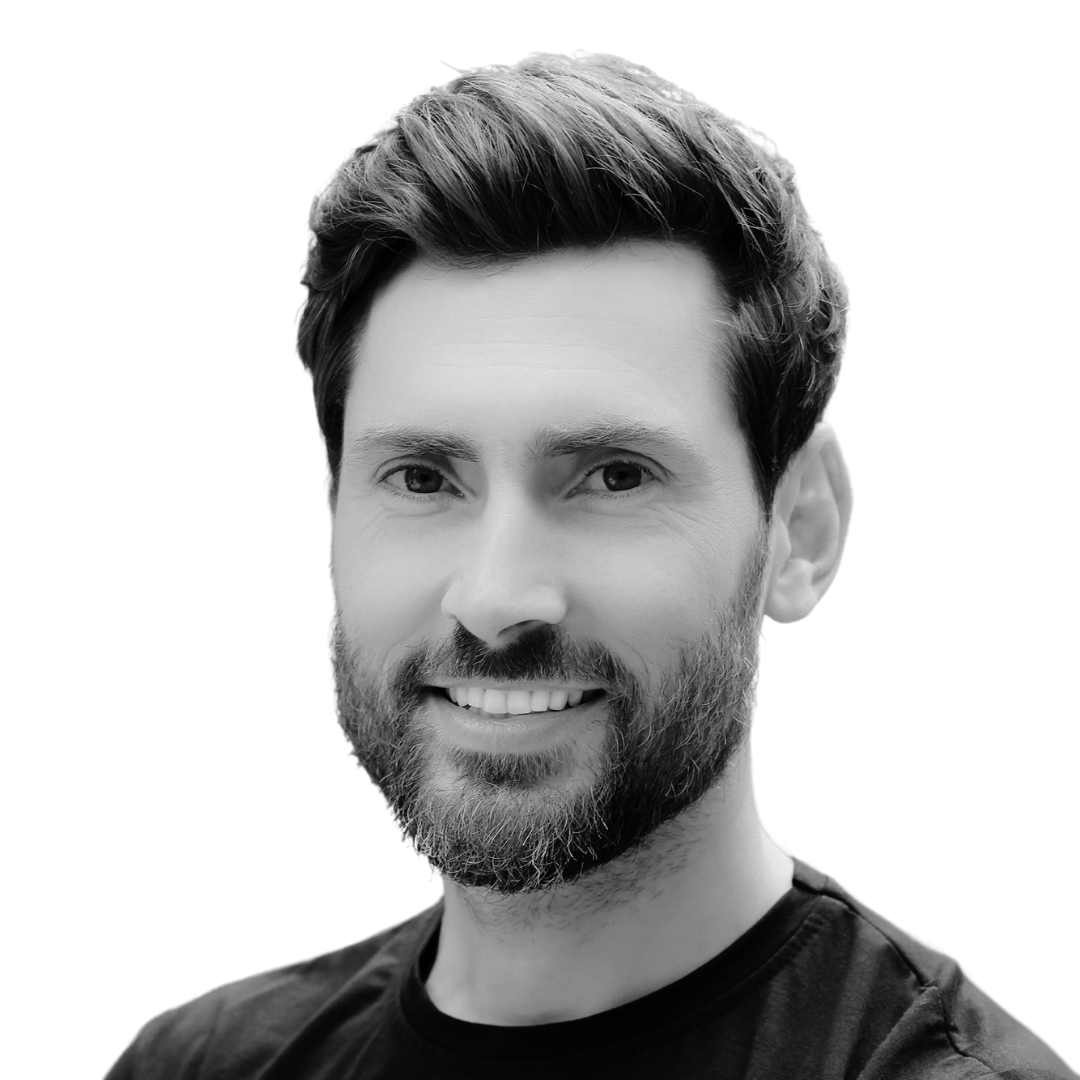
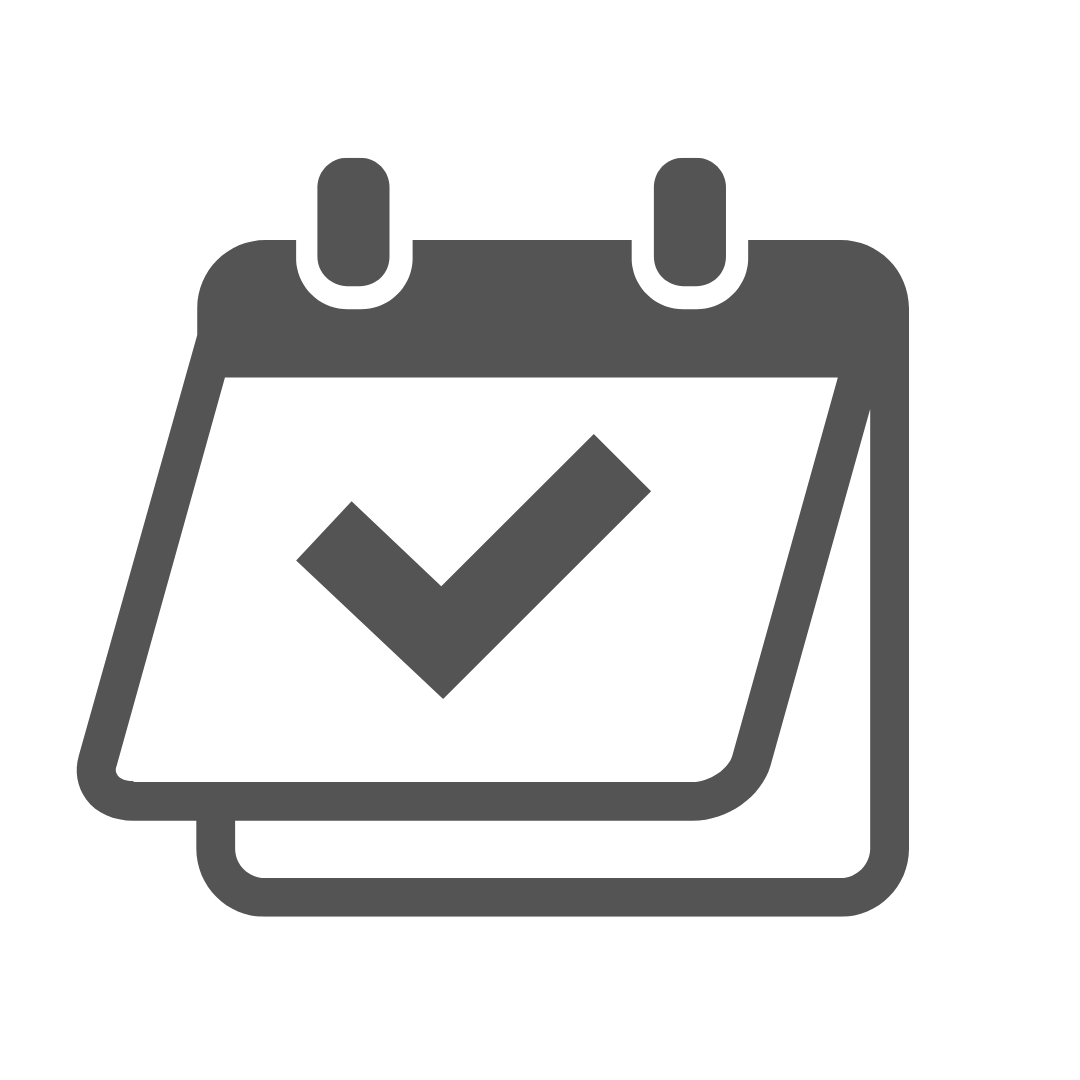
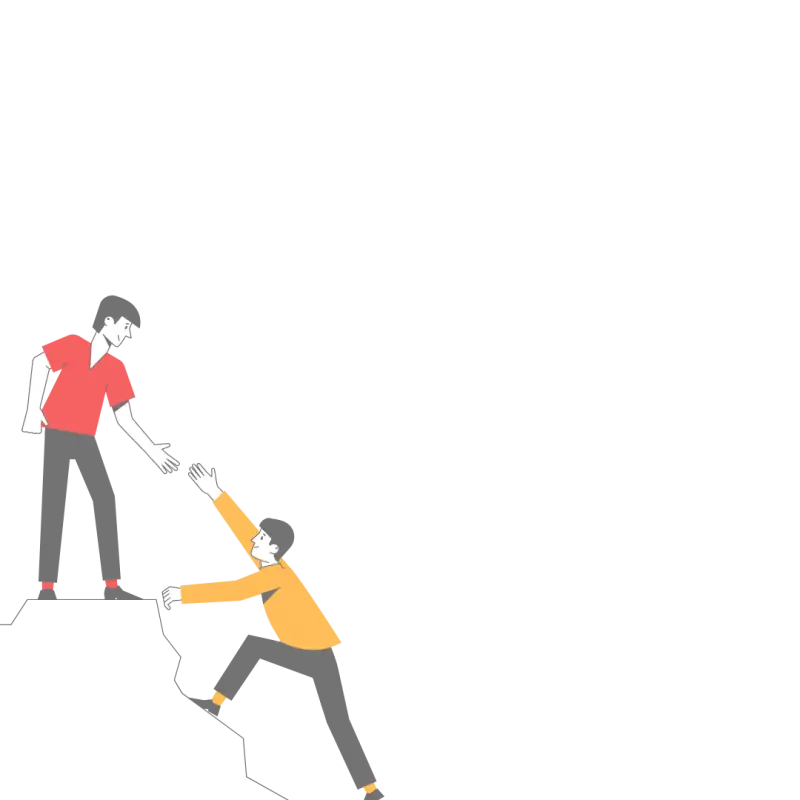
Thanks for your feedback!
Your contributions will help us to improve service.
How can conditional rendering be implemented using the ternary operator in React.js?
This React.js code demonstrates conditional rendering using a ternary operator. It initializes a state variable called isLoggedIn
to false
using the useState
hook.
When the user clicks the "Login" button, the handleLogin
function is called, setting isLoggedIn
to true
. Similarly, the handleLogout
function sets isLoggedIn
to false
when the "Logout" button is clicked.
The rendering logic uses the ternary operator to conditionally display different JSX elements based on the value of isLoggedIn
.
Output of React Js Conditional Rendering using tenary operator
How can I use the logical &&
operator for conditional rendering in React.js?
In this code snippet, React JS is used for conditional rendering using the logical "&&" operator. The component App
initializes the user
state as null
. After a delay of 2000 milliseconds (2 seconds), the user data is fetched asynchronously, and the user
state is updated with the user's name and admin status.
The rendering logic is based on the user
state. If user
is not null, it renders a welcome message with the user's name. Additionally, if the user is an admin, it displays a message indicating admin privileges. If user
is null, it shows a loading message.
The code uses the logical "&&" operator to conditionally render elements. If the condition before "&&" is true, the element after "&&" is rendered; otherwise, it is skipped.
Output of React Js Conditional Rendering using Logical && Operator
How can conditional rendering be achieved in React.js based on the length of an array?
This code snippet demonstrates conditional rendering in ReactJS based on the length of an array. It uses the useState
and useEffect
hooks from React. The array data
contains three elements: 'Boat', 'Bharatpe', and 'Lenskart'.
If the length of the array is greater than 0, it renders an unordered list (ul
) with each item as a list item (li
). Otherwise, it renders a paragraph (p
) stating "No data available."
The rendered content is wrapped in a div
with the class name 'container'.
How can conditional rendering be achieved in React.js using an if-else loop?
The code snippet demonstrates conditional rendering in React.js using an if-else loop. The renderContent
function checks the value of the count
variable.
If it's greater than zero, it renders a paragraph displaying the count. Otherwise, it renders a paragraph stating that no items are available. The rendered content is displayed within a container div.