React Js Get Last Item from Array
React Js Get Last Item from Array:To get the last item from an array in React.js, you can use multiple approaches. One way is to utilize the length property of the array. By subtracting 1 from the length, you can access the last item using array[length - 1].
Another method is to use the slice() method with a negative index, like array.slice(-1)[0], which extracts the last element. Alternatively, you can use the pop() method, which removes and returns the last element from the array, by simply calling array.pop(). All of these techniques achieve the goal of retrieving the last item from an array in React.js.
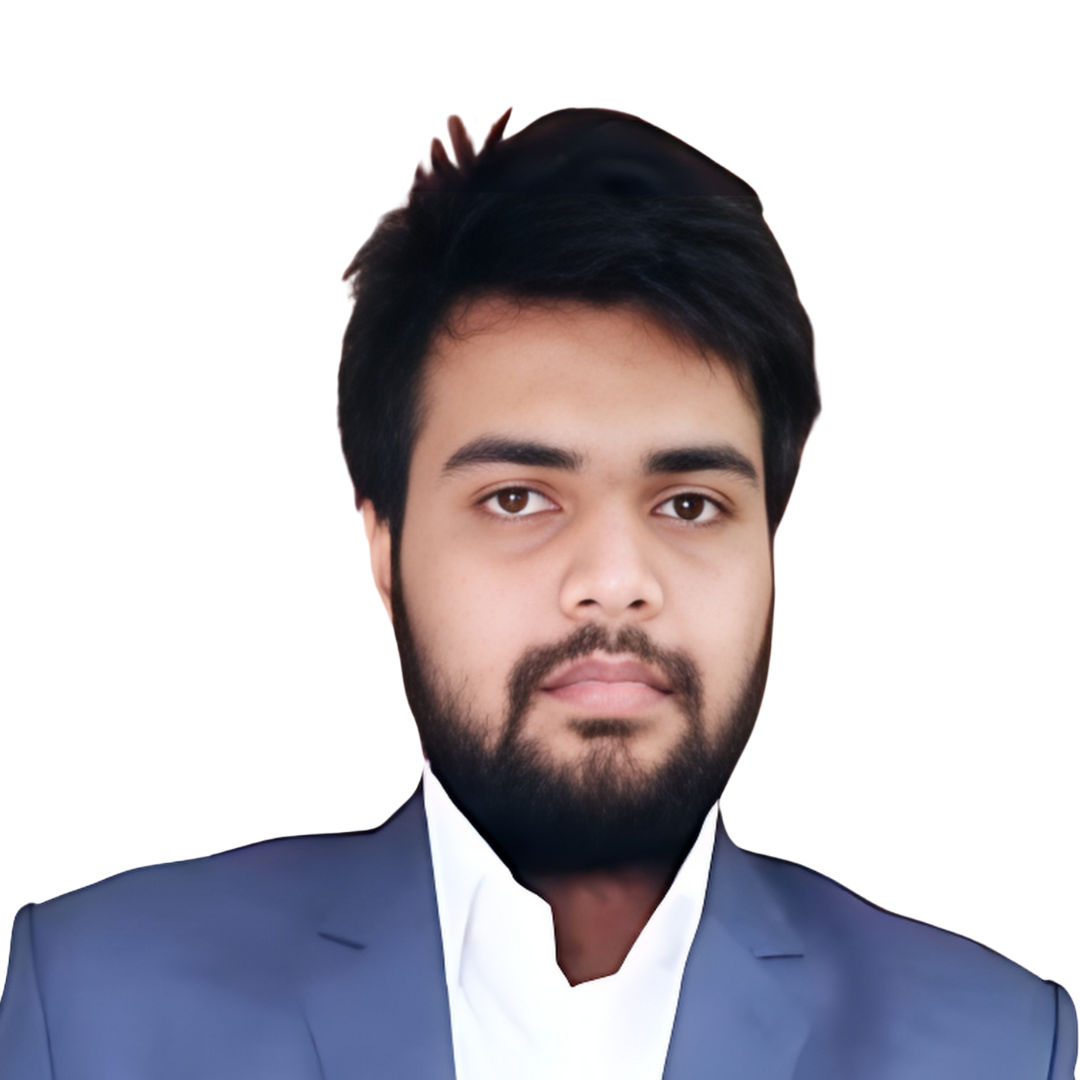
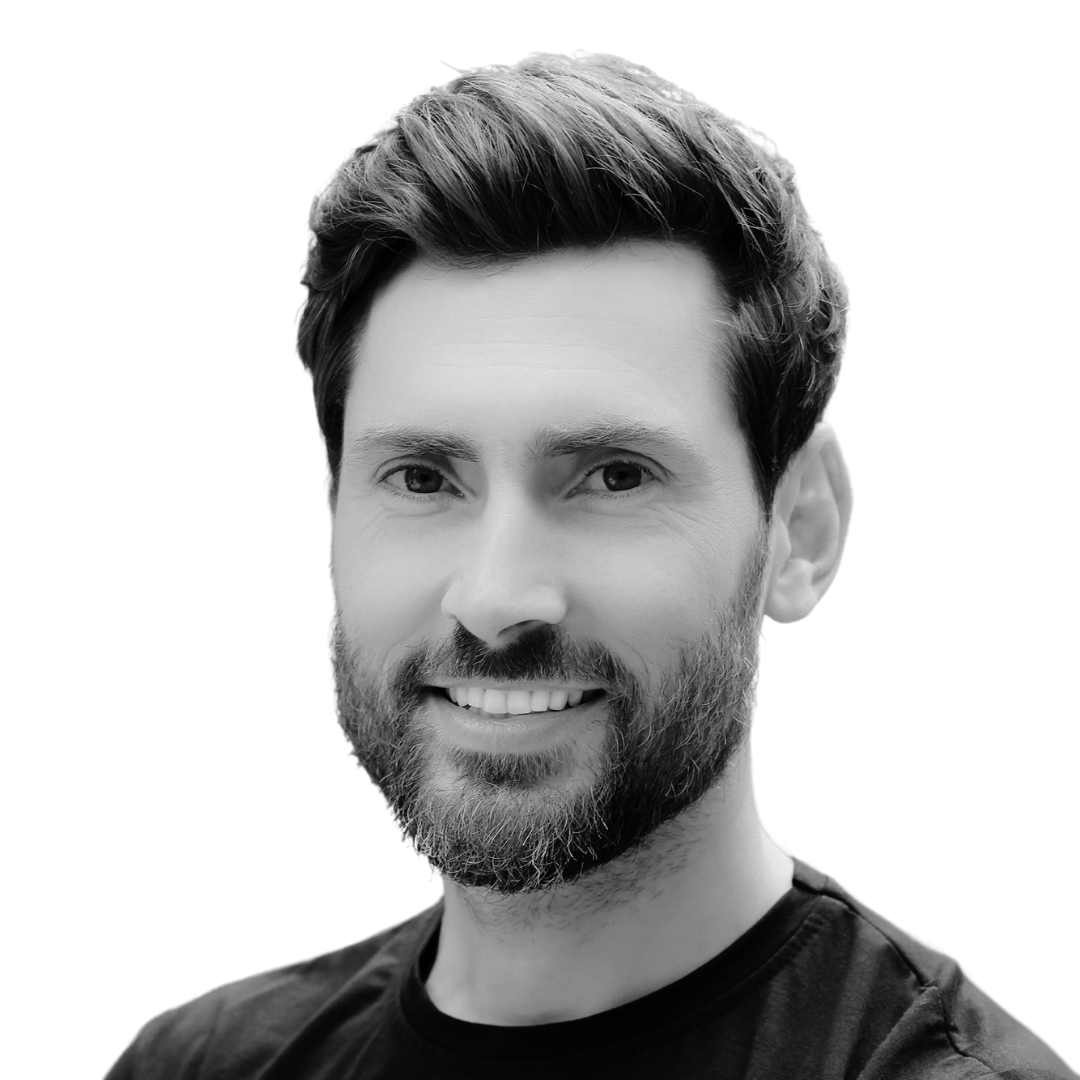
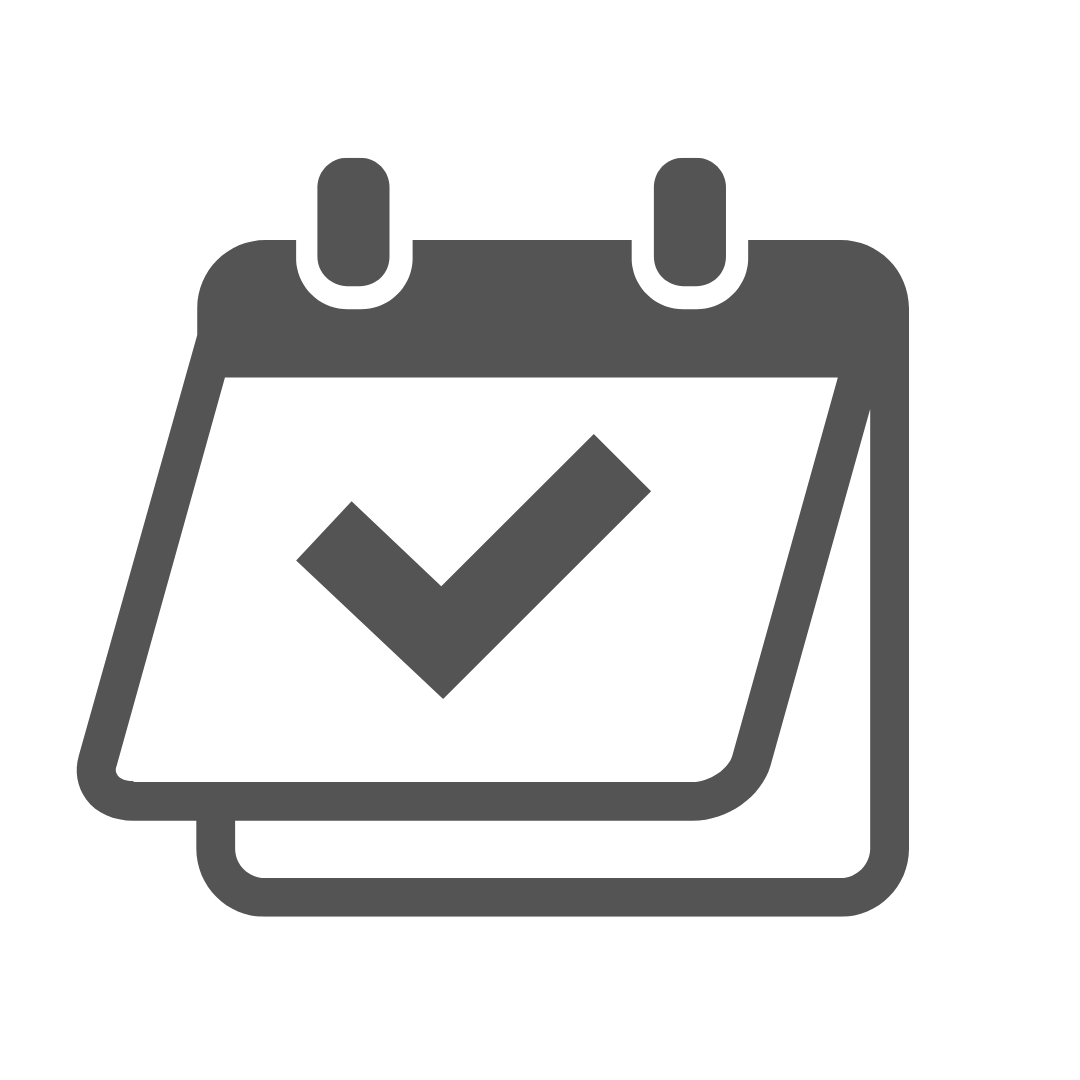
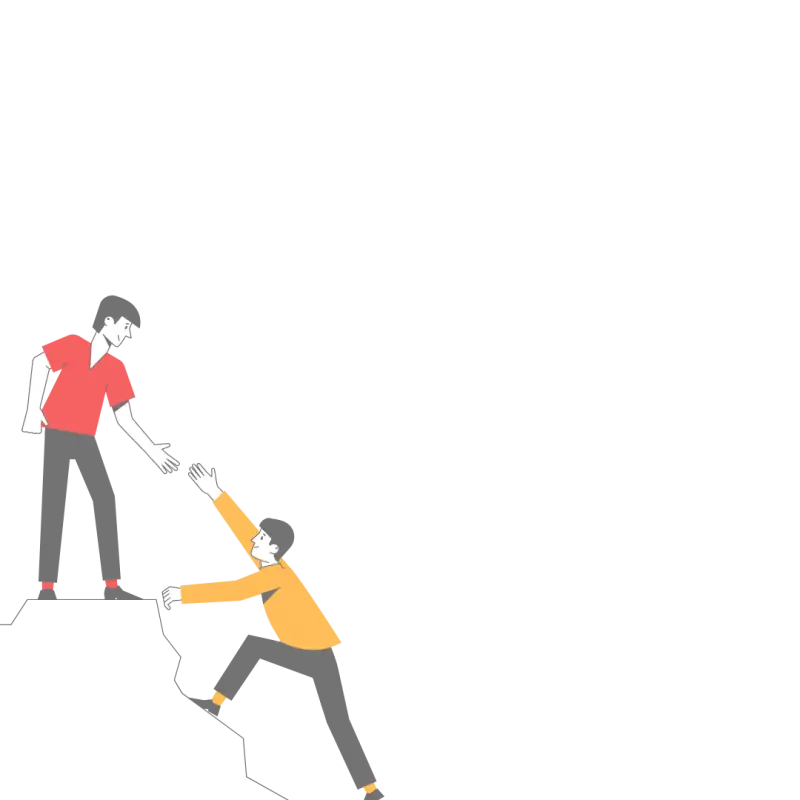
Thanks for your feedback!
Your contributions will help us to improve service.
How can you retrieve the last item of an array in React.js by utilizing the length property?
In this React.js code snippet, an array called array
is defined with four elements. To retrieve the last item of the array, the length property of the array is used. By subtracting 1 from the length, the index of the last item is obtained. The last item is then stored in the lastItem
variable. Finally, the array and the last item are displayed in the rendered HTML using JSX syntax.
Output of React Js Get Last Item from Array
How can the slice()
method be used in React.js to retrieve the last item of an array?
In the provided code snippet, React.js is used to get the last item of an array. The slice()
method is used with a negative index (-1) to extract the last element from the array. The value of lastItem
is assigned the result of the slice()
operation. The extracted last item is then displayed within the JSX code using the {lastItem}
expression.
How can you use the pop()
method in React.js to retrieve the last item of an array?
In the given code snippet, a React.js component named App
is defined. Inside this component, there is an array called array
containing four elements. The pop()
method is used on the array to remove and retrieve the last item, which is assigned to the variable lastItem
. The rendered output displays the original array and the last item using JSX syntax.
How can you retrieve the last object from an array of objects in React.js?
In this React.js code, an array of objects called myArray
is defined. To get the last object from this array, the variable lastObject
is assigned the value of myArray[myArray.length - 1]
. The lastObject
will hold the last element of the array. The code then renders a React component that displays the array elements and the name of the last object in the array.